Golang’s method of calling a function: use a map variable to explicitly associate the string with the function, and obtain the function object through [funcs["foo"]], the code is [funcs := map[string ]interface{} "foo": foo].
Golang’s method of calling a function:
Application scenario
We know a function name, Represent the function name through a string variable, and then how to call the function of this function.
func foo() { fmt.Println("in foo"); } var funcname string = "foo" func callFunc(funcname string) { ... } callFunc(funcname)
The following question is how do we implement the content of callFunc()
to complete the required function.
The currently known methods do not seem to work. It is impossible to obtain a function object by name from the global domain.
(The same is true for variables, you cannot get a variable object by name from the global domain)
Feasible methods
Use a map variable to explicitly combine the string with the function ( Variables) are associated:
funcs := map[string]interface{} { "foo": foo, }
In this way, we can get the function object through funcs["foo"]
, and then we can operate the function.
Example of function operation
The following is an example of a function call, including the passing of parameters and the processing of return values.
package main import "fmt" import "reflect" import "errors" type MyStruct struct { i int s string } func foo0() int { fmt.Println("running foo0: ") return 100 } func foo1(a int) (string, string) { fmt.Println("running foo1: ", a) return "aaaa", "bbb" } func foo2(a, b int, c string) MyStruct { fmt.Println("running foo2: ", a, b, c) return MyStruct{10, "ccc"} } func Call(m map[string]interface{}, name string, params ... interface{}) (result []reflect.Value, err error) { f := reflect.ValueOf(m[name]) if len(params) != f.Type().NumIn() { err = errors.New("The number of params is not adapted.") return } in := make([]reflect.Value, len(params)) for k, param := range params { in[k] = reflect.ValueOf(param) } result = f.Call(in) return } func main() { funcs := map[string]interface{} { "foo0": foo0, "foo1": foo1, "foo2": foo2, } // call foo0 if result, err := Call(funcs, "foo0"); err == nil { for _, r := range result { fmt.Printf(" return: type=%v, value=[%d]\n", r.Type(), r.Int()) } } // call foo1 if result, err := Call(funcs, "foo1", 1); err == nil { for _, r := range result { fmt.Printf(" return: type=%v, value=[%s]\n", r.Type(), r.String()) } } // call foo2 if result, err := Call(funcs, "foo2", 1, 2, "aa"); err == nil { for _, r := range result { fmt.Printf(" return: type=%v, value=[%+v]\n", r.Type(), r.Interface().(MyStruct)) } } }
Compile and run:
running foo0: return: type=int, value=[100] running foo1: 1 return: type=string, value=[aaaa] return: type=string, value=[bbb] running foo2: 1 2 aa return: type=main.MyStruct, value=[{i:10 s:ccc}]
Related learning recommendations: Go language tutorial
The above is the detailed content of How to call functions in golang?. For more information, please follow other related articles on the PHP Chinese website!
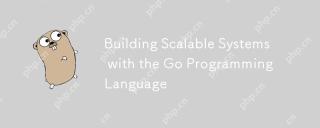
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
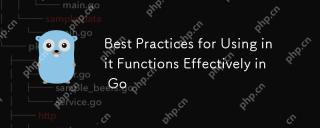
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
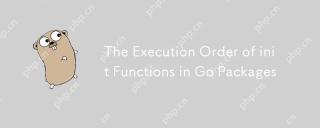
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations
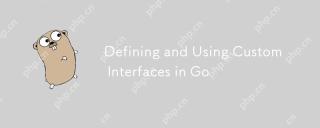
CustominterfacesinGoarecrucialforwritingflexible,maintainable,andtestablecode.Theyenabledeveloperstofocusonbehavioroverimplementation,enhancingmodularityandrobustness.Bydefiningmethodsignaturesthattypesmustimplement,interfacesallowforcodereusabilitya
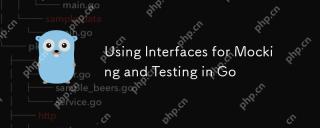
The reason for using interfaces for simulation and testing is that the interface allows the definition of contracts without specifying implementations, making the tests more isolated and easy to maintain. 1) Implicit implementation of the interface makes it simple to create mock objects, which can replace real implementations in testing. 2) Using interfaces can easily replace the real implementation of the service in unit tests, reducing test complexity and time. 3) The flexibility provided by the interface allows for changes in simulated behavior for different test cases. 4) Interfaces help design testable code from the beginning, improving the modularity and maintainability of the code.
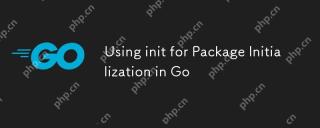
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
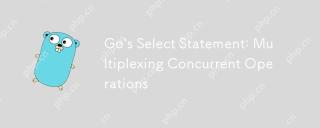
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
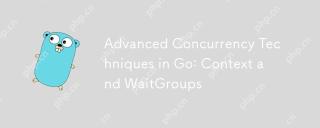
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
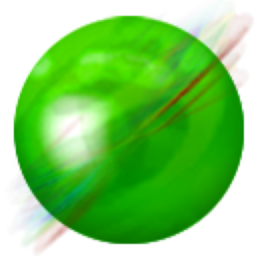
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
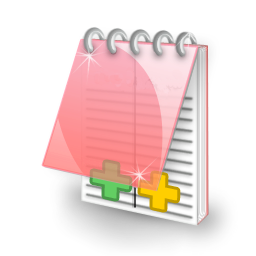
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
