


Sometimes in the project we need to use PHP to encrypt specific information, that is, to generate an encrypted string through the encryption algorithm. These encrypted strings can be decrypted through the decryption algorithm to facilitate the program to process the decrypted information. .
The most common applications are in user login and some API data exchange scenarios. The most common applications are in user login and some API data exchange scenarios. The principle of encryption and decryption is generally to use a certain encryption and decryption algorithm, add the key to the algorithm, and finally obtain the encryption and decryption results.
Without further ado, let’s get straight to the code.
1. The first reversible encryption function for ID can also be used for invitation codes and the like. The decrypted data is relatively simple
Example: lockcode(28)=》 000X unlockcode('000X')=》28
//加密函数 function lockcode($code) { static $source_string = 'E5FCDG3HQA4B1NOPIJ2RSTUV67MWX89KLYZ'; $num = $code; $code = ''; while ( $num > 0) { $mod = $num % 35; $num = ($num - $mod) / 35; $code = $source_string[$mod].$code; } if(empty($code[3])) $code = str_pad($code,4,'0',STR_PAD_LEFT); return $code; } //解密函数 function unlockcode($code) { static $source_string = 'E5FCDG3HQA4B1NOPIJ2RSTUV67MWX89KLYZ'; if (strrpos($code, '0') !== false) $code = substr($code, strrpos($code, '0')+1); $len = strlen($code); $code = strrev($code); $num = 0; for ($i=0; $i < $len; $i++) { $num += strpos($source_string, $code[$i]) * pow(35, $i); } return $num; }
2. The second is the encryption function that I searched on the Internet. It is very easy to use, reversible encryption, and supports salt parameters.
Example:
encrypt('abcd','1234')=》nkiV93IfJ decrypt('nkiV93IfJ','1234')=》abcd
//加密函数 function encrypt($data,$key='CHENI'){ $chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; $nh = rand(0,64); $ch = $chars[$nh]; $mdKey = md5($key.$ch); $mdKey = substr($mdKey,$nh%8, $nh%8+7); $data= base64_encode($data); $tmp = ''; $i=0;$j=0;$k = 0; for ($i=0; $i<strlen($data); $i++) { $k = $k == strlen($mdKey) ? 0 : $k; $j = ($nh+strpos($chars,$data[$i])+ord($mdKey[$k++]))%64; $tmp .= $chars[$j]; } return urlencode($ch.$tmp); } //解密函数 function decrypt($data,$key='CHENI'){ $txt = urldecode($data); $chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789"; $ch = $txt[0]; $nh = strpos($chars,$ch); $mdKey = md5($key.$ch); $mdKey = substr($mdKey,$nh%8, $nh%8+7); $txt = substr($txt,1); $tmp = ''; $i=0;$j=0; $k = 0; for ($i=0; $i<strlen($txt); $i++) { $k = $k == strlen($mdKey) ? 0 : $k; $j = strpos($chars,$txt[$i])-$nh - ord($mdKey[$k++]); while ($j<0) $j+=64; $tmp .= $chars[$j]; } return base64_decode($tmp); }
Third, the third type is similar to the above, and also supports salt value parameters
Example: encrypt('abcd','1234')=》mZPHxw== decrypt( 'mZPHxw==','1234')=》abcd
function encrypt($data, $key) { $char=""; $str=""; $key = md5($key); $x = 0; $len = strlen($data); $l = strlen($key); for ($i = 0; $i < $len; $i++) { if ($x == $l) { $x = 0; } $char .= $key{$x}; $x++; } for ($i = 0; $i < $len; $i++){ $str .= chr(ord($data{$i}) + (ord($char{$i})) % 256); } return base64_encode($str); } function decrypt($data, $key) { $key = md5($key); $x = 0; $data = base64_decode($data); $len = strlen($data); $l = strlen($key); for ($i = 0; $i < $len; $i++) { if ($x == $l){ $x = 0;} $char .= substr($key, $x, 1); $x++; } for ($i = 0; $i < $len; $i++){ if (ord(substr($data, $i, 1)) < ord(substr($char, $i, 1))){ $str .= chr((ord(substr($data, $i, 1)) + 256) - ord(substr($char, $i, 1))); }else{ $str .= chr(ord(substr($data, $i, 1)) - ord(substr($char, $i, 1))); } } return $str; }
4. This is the best one I have ever used, the encryption and decryption algorithm used in discuz
//加密算法 function authcode($string,$key='',$operation=false,$expiry=0){ $ckey_length = 4; $key = md5($key ? $key : DEFAULT_KEYS); $keya = md5(substr($key, 0, 16)); $keyb = md5(substr($key, 16, 16)); $keyc = $ckey_length ? ($operation? substr($string, 0, $ckey_length):substr(md5(microtime()), -$ckey_length)) : ''; $cryptkey = $keya.md5($keya.$keyc); $key_length = strlen($cryptkey); $string = $operation? base64_decode(substr($string, $ckey_length)) : sprintf('%010d', $expiry ? $expiry + time() : 0).substr(md5($string.$keyb), 0, 16).$string; $string_length = strlen($string); $result = ''; $box = range(0, 255); $rndkey = array(); for($i = 0; $i <= 255; $i++) { $rndkey[$i] = ord($cryptkey[$i % $key_length]); } for($j = $i = 0; $i < 256; $i++) { $j = ($j + $box[$i] + $rndkey[$i]) % 256; $tmp = $box[$i]; $box[$i] = $box[$j]; $box[$j] = $tmp; } for($a = $j = $i = 0; $i < $string_length; $i++) { $a = ($a + 1) % 256; $j = ($j + $box[$a]) % 256; $tmp = $box[$a]; $box[$a] = $box[$j]; $box[$j] = $tmp; $result .= chr(ord($string[$i]) ^ ($box[($box[$a] + $box[$j]) % 256])); } if($operation) { if((substr($result, 0, 10) == 0 || substr($result, 0, 10) - time() > 0) && substr($result, 10, 16) == substr(md5(substr($result, 26).$keyb), 0, 16)) { return substr($result, 26); } else { return ''; } } else { return $keyc.str_replace('=', '', base64_encode($result)); } } echo authcode('123456','key'); echo '<br>'; echo authcode('7d49kn9k07uSBZvha8as+/qm4UoLfpy88PFg12glPeDtlzc','key',true);
For more PHP related knowledge, please visit PHP Tutorial!
The above is the detailed content of Four useful PHP custom encryption functions (reversible/irreversible). For more information, please follow other related articles on the PHP Chinese website!
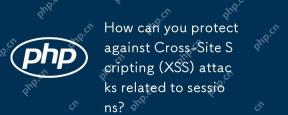
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
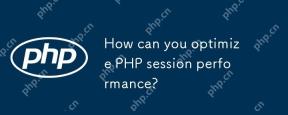
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
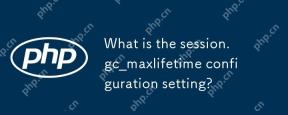
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
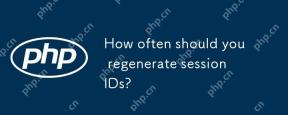
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
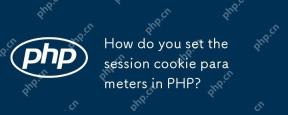
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
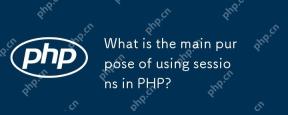
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
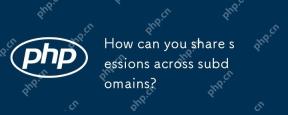
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
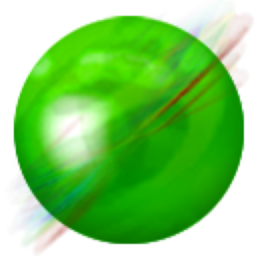
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
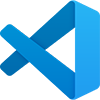
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version