In-depth understanding of coroutines and blocking in php
This article mainly introduces you to some understanding and thinking about coroutines and blocking in PHP. The article introduces it in detail through example code. It has certain reference learning value for everyone to learn or use PHP. Friends who need it Let’s learn with the editor below.
Preface
This article mainly introduces to you the understanding and thinking about coroutines and blocking in PHP, and shares it for your reference and study. Not much to say below, let’s take a look at the detailed introduction:
Process, thread, coroutine
About process , threads, and coroutines, there are very detailed and rich blogs or learning resources. I will not go into details here. I will briefly introduce these things here.
The process has its own independent heap and stack. It neither shares the heap nor the stack. The process is scheduled by the operating system.
Threads have their own independent stack and shared heap. The heap is shared but the stack is not shared. Threads are also scheduled by the operating system (standard threads are).
Coroutines share the heap like threads, but do not share stacks. Coroutines are scheduled explicitly by programmers in the code of the coroutine.
Coroutine implementation in PHP is based on yield
The fundamental implementation of yield is to generate Iterator class, and the iterator class is the implementation of the iterator interface:
Generator implements Iterator { public mixed current ( void ) // 返回当前产生的值 public mixed key ( void ) // 返回当前产生的键 public void next ( void ) // 生成器继续执行 public void rewind ( void ) // 重置迭代器,如果迭代已经开始了,这里会抛出一个异常。 // renwind的执行将会导致第一个yield被执行, 并且忽略了他的返回值. public mixed send ( mixed $value ) // 向生成器中传入一个值,并且当做 yield 表达式的结果,然后继续执行生成器。如果当这个方法被调用时,生成器 // 不在 yield 表达式,那么在传入值之前,它会先运行到第一个 yield 表达式。 public void throw ( Exception $exception ) // 向生成器中抛入一个异常 public bool valid ( void ) // 检查迭代器是否被关闭 public void __wakeup ( void ) // 序列化回调,抛出一个异常以表示生成器不能被序列化。 }
I will give an example based on the coroutine multi-task scheduling he implemented. And talk about some of my thoughts on blocking.
Example of customizing simple scheduled execution tasks:
(This example must rely on the above coroutine scheduling code implemented by Brother Niao )
class timer { private $start = 0; // 定时开始时间 private $timer; // 间隔的时间差,单位秒 private $value = 0; // 产生的结果值 private $callback; // 异步回调 private $isEnd = false; // 当前定时器任务是否结束 public function __construct($timer,callable $callback) { $this->start = time(); $this->timer = $timer; $this->callback = $callback; } public function run() { if($this->valid()) { $callback = $this->callback; $callback($this->value ++,$this); $this->start = time(); } } /** * 定时执行检查 */ public function valid() { $end = time(); if($end - $this->start >= $this->timer) { return true; } else { return false; } } public function setEnd($isEnd) { $this->isEnd = $isEnd; } public function getEnd() { return $this->isEnd; } } /** * 模拟阻塞的协程1 * */ function taskObject1() { $timer = new timer(1,function($value,timer $timer) { if($value >= 5) { $timer->setEnd(true); } echo '<br>'.'A '.$value; }); $tid = (yield getTaskId()); while (true) { if($timer->getEnd() == true) { break; } yield $timer->run(); } } /** * 模拟阻塞的协程2 * */ function taskObject2() { $timer = new timer(2,function($value,timer $timer) { if($value >= 3) { $timer->setEnd(true); } echo '<br>'.'B '.$value; }); $tid = (yield getTaskId()); while (true) { if($timer->getEnd() == true) { break; } yield $timer->run(); } } $scheduler = new Scheduler; $scheduler->newTask(taskObject1()); $scheduler->newTask(taskObject2()); $scheduler->run();
The above implementation is:
Generate two tasks, execute them in parallel, and give each task the execution time Simulate a few seconds of blocking;
Let the coroutine switch smoothly, and the task blocking does not affect each other;
Thinking:
Why should I do the above thing? Because I found that although the coroutine implementation is very powerful and interesting, and can enable multi-tasking in parallel, when I call the system function sleep()
in one of the tasks, the blocking task will prevent the coroutine switching. In fact, This is also the case from the book's perspective on the implementation principles of coroutines.
Then, I also want to simulate coroutine blocking, but do not cause blocking to see if it is feasible. PHP itself only provides generators to provide support for coroutine calls. If it does not rely on extensions, it does not provide a multi-threaded program implementation method. It is not as powerful as Java and can be implemented by opening sub-threads.
I have the impression that Java's sub-threads execute independently and do not block each other, so I am thinking that since PHP can implement a mechanism similar to multi-threading, can it achieve non-blocking during the call process? ?
After such an implementation and thinking, I fell into a misunderstanding at the beginning. It was a misunderstanding caused by the blocking of the PHP native function sleep()
, that is, in order to truly achieve something special, Blocking or asynchronous implementation must depend on the underlying language.
Later, I figured out a truth. Since a certain method or function will block during execution, then replace the current method with a custom one and make it non-blocking (relative to the entire protocol). In terms of scheduling, wouldn’t it be enough? For example, I implemented the above scheduled execution myself.
On the other hand, the purpose of coroutine scheduling itself is to cut the task execution process into as small pieces as possible, so as to quickly switch execution and achieve parallelism. From this perspective, coroutine should also be regarded as a programming idea.
The following is an example of cutting a program into as small pieces as possible for execution:
##
// 一个简单的例子 <?php function xrange($start, $end, $step = 1) { for ($i = $start; $i <= $end; $i += $step) { yield $i; } } foreach (xrange(1, 1000000) as $num) { echo $num, "\n"; }This example is to use range The method of generating a large integer array is switched to shard execution, which means that the specified value is obtained during traversal. From the code point of view, the memory consumption is very small compared to before.
Summarize
The above is the detailed content of In-depth understanding of coroutines and blocking in php. For more information, please follow other related articles on the PHP Chinese website!
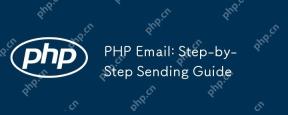
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
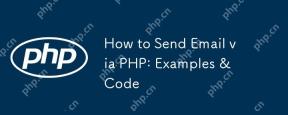
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
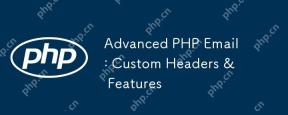
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
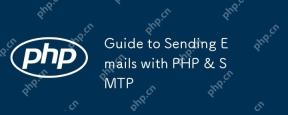
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
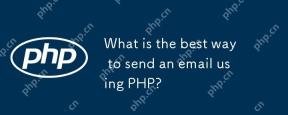
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
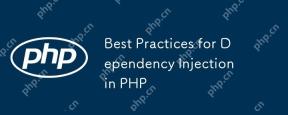
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
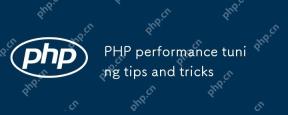
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
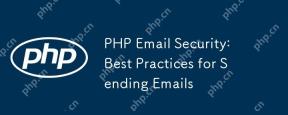
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
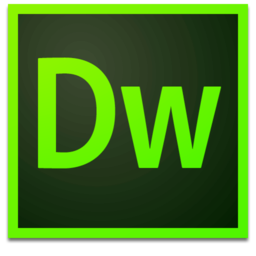
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
