


Detailed explanation of php magic method comparison and use of example code
In PHP, methods starting with two underscores are called magic methods. These methods play a decisive role in PHP.
construct() and destruct()
Constructor function and destructor should be familiar, they are used in object creation and destruction is called when. For example, we need to open a file, open it when the object is created, and close it when the object dies
<?php class FileRead { protected $handle = NULL; function construct(){ $this->handle = fopen(...); } function destruct(){ fclose($this->handle); } } ?>
These two methods can be expanded when inheriting, for example:
<?php class TmpFileRead extends FileRead { function construct(){ parent::construct(); } function destruct(){ parent::destruct(); } } ?>
call() and callStatic()
These two methods will be called when an inaccessible method is called in the object, and the latter is a static method. These two methods may be used in variable method (Variable functions) calls.
<?php class MethodTest { public function call ($name, $arguments) { echo "Calling object method '$name' ". implode(', ', $arguments). "\n"; } public static function callStatic ($name, $arguments) { echo "Calling static method '$name' ". implode(', ', $arguments). "\n"; } } $obj = new MethodTest; $obj->runTest('in object context'); MethodTest::runTest('in static context'); ?>
get(), set(), isset() and unset()
These two functions are called when get/set a member variable of a class. For example, we save the object variables in another array instead of the member variables of the object itself
<?php class MethodTest { private $data = array(); public function set($name, $value){ $this->data[$name] = $value; } public function get($name){ if(array_key_exists($name, $this->data)) return $this->data[$name]; return NULL; } public function isset($name){ return isset($this->data[$name]) } public function unset($name){ unset($this->data[$name]); } } ?>
sleep() and wakeup()
When we are executing serialize () and unserialize(), these two functions will be called first. For example, when we serialize an object, the object has a database link. If we want to restore the link state during deserialization, we can restore the link by reconstructing these two functions. Examples are as follows:
<?php class Connection { protected $link; private $server, $username, $password, $db; public function construct($server, $username, $password, $db) { $this->server = $server; $this->username = $username; $this->password = $password; $this->db = $db; $this->connect(); } private function connect() { $this->link = mysql_connect($this->server, $this->username, $this->password); mysql_select_db($this->db, $this->link); } public function sleep() { return array('server', 'username', 'password', 'db'); } public function wakeup() { $this->connect(); } } ?>
toString()
The response method when the object is treated as a string. For example, use echo $obj; to output an object
<?php // Declare a simple class class TestClass { public function toString() { return 'this is a object'; } } $class = new TestClass(); echo $class; ?>
This method can only return a string, and you cannot throw an exception in this method, otherwise a fatal error will occur.
invoke()
The response method when calling an object by calling a function. As follows
<?php class CallableClass { function invoke() { echo 'this is a object'; } } $obj = new CallableClass; var_dump(is_callable($obj)); ?>
set_state()
When calling var_export() to export a class, this static method will be called.
<?php class A { public $var1; public $var2; public static function set_state ($an_array) { $obj = new A; $obj->var1 = $an_array['var1']; $obj->var2 = $an_array['var2']; return $obj; } } $a = new A; $a->var1 = 5; $a->var2 = 'foo'; var_dump(var_export($a)); ?>
clone()
Called when the object copy is completed. For example, in the singleton mode implementation method mentioned in the article Detailed Explanation of Design Patterns and PHP Implementation: Singleton Mode, this function is used to prevent objects from being cloned.
<?php public class Singleton { private static $_instance = NULL; // 私有构造方法 private function construct() {} public static function getInstance() { if (is_null(self::$_instance)) { self::$_instance = new Singleton(); } return self::$_instance; } // 防止克隆实例 public function clone(){ die('Clone is not allowed.' . E_USER_ERROR); } } ?>
The above is the detailed content of Detailed explanation of php magic method comparison and use of example code. For more information, please follow other related articles on the PHP Chinese website!
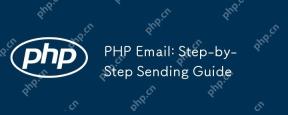
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
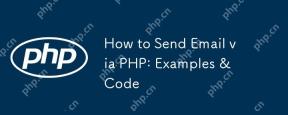
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
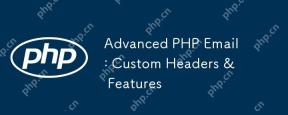
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
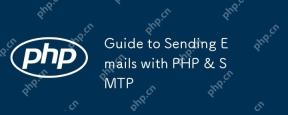
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
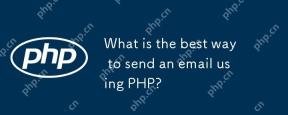
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
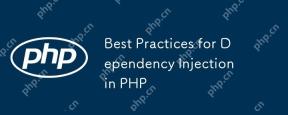
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
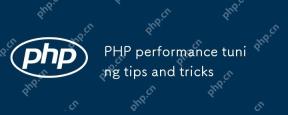
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
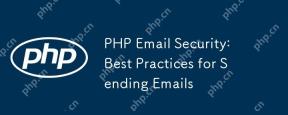
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
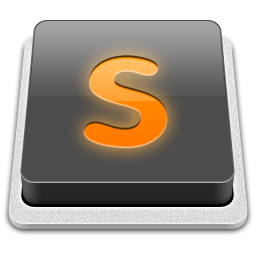
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version
