In order to prevent some people from uploading executable files such as exe to the server, we can write a program to determine the type of the uploaded file, and then files that do not meet the type will be refused to upload.
The following is the PHP program that implements this function:
function ($file_name, $pass_type = array('jpg','jpeg','gif','bmp','png') ) { // 允许文件类型的后缀组成的数组 $file = $pass_type; // 截取上传文件的文件名的后缀 $kzm = substr(strrchr($file_name,"."),1); // 判断此后缀是否在数组中 $is_img = in_array(strtolower($kzm),$file); if($is_img) { return true; } else { return false; } }
in_array() function
in_array() function searches an array for a given value. Usage: in_array(value,array,type).
- Parameter value, required. Specifies the value to search for in the array.
- Parameter array, required. Specifies the array to search.
- Parameter type, optional. If this parameter is set to true, it is checked whether the type of the searched data and the value of the array are the same.
Returns true if the given value value exists in the array array. If the third parameter is set to true, the function returns true only if the element exists in the array and has the same data type as the given value.
If the parameter is not found in the array, the function returns false.
If the value parameter is a string and the type parameter is set to true, the search is case-sensitive.
<?php $people = array("Peter", "Joe", "Glenn", "Cleveland"); if (in_array("Glenn",$people)) { echo "Match found"; } else { echo "Match not found"; } ?>
Program output:
Match found
Another sample program:
<?php $people = array("Peter", "Joe", "Glenn", "Cleveland", 23); if (in_array("23",$people, TRUE)) { echo "Match found<br />"; } else { echo "Match not found<br />"; } if (in_array("Glenn",$people, TRUE)) { echo "Match found<br />"; } else { echo "Match not found<br />"; } if (in_array(23,$people, TRUE)) { echo "Match found<br />"; } else { echo "Match not found<br />"; } ?>
Program output:
Match not found Match found Match found
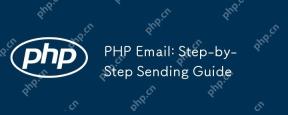
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
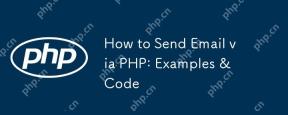
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
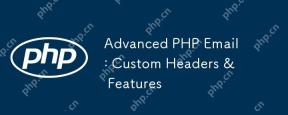
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
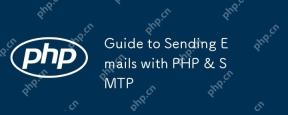
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
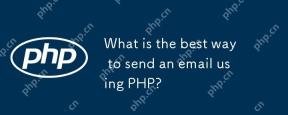
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
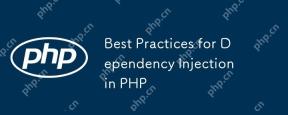
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
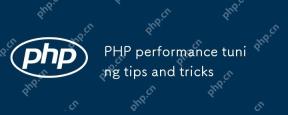
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
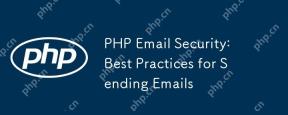
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
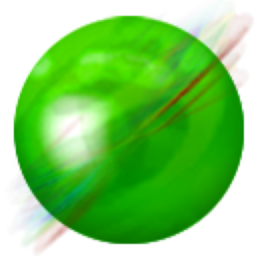
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
