


PHP implementation code for operating XML, reading data and writing data, phpxml
xml file
<?xml version="1.0" encoding="utf-8"?> <vip> <id>23</id> <username>开心的路飞</username> <sex>男</sex> <face>face/43.jpg</face> <email>123@qq.com</email> <qq>1212121212</qq> </vip>
PHP parses XML to get the value in the tag
/* * _get_xml 获取的XML文件 * @access public 表示函数对外公开 * @param $_xmlfile xml文件 * $_html 从XML中取出的数据数组 * */ function _get_xml($_xmlfile){ $_html = array(); if(file_exists($_xmlfile)){ $_xml = file_get_contents($_xmlfile); preg_match_all('/<vip>(.*)<\/vip>/', $_xml,$_dom); foreach($_dom[1] as $_value){ preg_match_all('/<id>(.*)<\/id>/', $_value,$_id); preg_match_all('/<username>(.*)<\/username>/', $_value,$_username); preg_match_all('/<sex>(.*)<\/sex>/', $_value,$_sex); preg_match_all('/<face>(.*)<\/face>/', $_value,$_face); preg_match_all('/<email>(.*)<\/email>/', $_value,$_email); preg_match_all('/<qq>(.*)<\/qq>/', $_value,$_qq); $_html['id'] = $_id[1][0]; $_html['username'] = $_username[1][0]; $_html['sex'] = $_sex[1][0]; $_html['face'] = $_face[1][0]; $_html['email'] = $_email[1][0]; $_html['qq'] = $_qq[1][0]; } }else{ _alert_back("文件不存在"); } return $_html; }
php writes data to XML file
/* * _set_xml将信息写入XML文件 * @access public 表示函数对外公开 * @param $_xmlfile xml文件 * @param $_clean 要写入的信息的数组 * */ function _set_xml($_xmlfile,$_clean){ $_fp = @fopen('newuser.xml','w'); if(!$_fp){ exit('系统错误,文件不存在!'); } flock($_fp,LOCK_EX); $_string = "<?xml version=\"1.0\" encoding=\"utf-8\"?>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "<vip>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "\t<id>{$_clean['id']}</id>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "\t<username>{$_clean['username']}</username>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "\t<sex>{$_clean['sex']}</sex>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "\t<face>{$_clean['face']}</face>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "\t<email>{$_clean['email']}</email>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "\t<qq>{$_clean['url']}</qq>\r\t"; fwrite($_fp, $_string,strlen($_string)); $_string = "</vip>"; fwrite($_fp, $_string,strlen($_string)); flock($_fp,LOCK_UN); fclose($_fp); }
I hope some information found on the Internet can help you introduce the related functions of xml reading in php: Quote:---------------------- -------------------------------------------------- --------Object XML parsing function description
Element xml_set_element_handler() The beginning and end of the element
Character data xml_set_character_data_handler() The beginning of character data
External entity xml_set_external_entity_ref_handler() The external entity appears
Unparsed external entity xml_set_unparsed_entity_decl_handler() The occurrence of unresolved external entity
The occurrence of processing instruction xml_set_processing_instruction_handler() The occurrence of processing instruction
The occurrence of notation declaration xml_set_notation_decl_handler() The occurrence of notation declaration
Default xml_set_default_handler() Others are not specified Handling function events------------------------------------------------- ---------------------------------- Let me give you a small example to read using the parser function. xml data: $parser = xml_parser_create(); //Create a parser editor
xml_set_element_handler($parser, "startElement", "endElement");//Set the corresponding function when the tag is triggered Here are startElement and endElenment respectively
xml_set_character_data_handler($parser, "characterData");//Set up the corresponding function when reading data
$xml_file="1.xml";//Specify the xml file to be read , can be url
$filehandler = fopen($xml_file, "r");//Open file
while ($data = fread($filehandler, 4096))
{
xml_parse($ parser, $data, feof($filehandler));
}//Take out 4096 bytes each time for processing fclose($filehandler);
xml_parser_free($parser);//Close and release the parser parser
$name=false;
$position=false;
function startElement($parser_instance, $element_name, $attrs) //Function of start tag event
{
global $name, $position;
if($element_name=="NAME")
{
$name=true;
$position=false;
echo "name:";
}
if($element_name=="POSITION")
{$name=false;
$position=true;
echo...the rest of the text>>
My idea is to use dynamic xml directly and let flash read the document, so that there is no need to generate xml files in real time. Of course, this xml file is in .php format, so you must change the read file address to php in flash, just like you write a php page. The difference is that the content output by this php file is in an xml format. text.
For example, if you now create the file xml.php
echo "
//If there is dynamic information here, call it as needed
echo"
< ;items>";
//Loop your image data here
$data = ??
while( $data ) {
echo "
}
echo '> ;';
?>
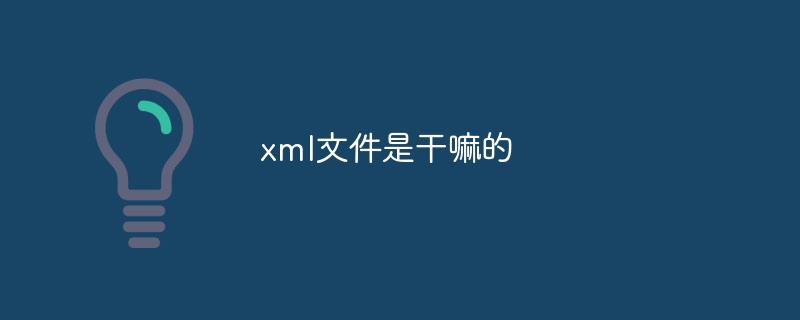
XML文件是用于描述和传输数据的一种标记语言。它以其可扩展性、可读性和灵活性而闻名,广泛应用于Web应用程序、数据交换和Web服务。XML的格式和结构使得数据的组织和解释变得简单明了,从而提高了数据的交换和共享效率 。
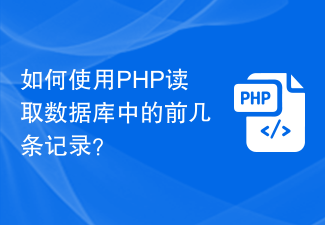
如何使用PHP读取数据库中的前几条记录?在开发Web应用程序时,我们经常需要从数据库中读取数据并展示给用户。有时候,我们只需要显示数据库中的前几条记录,而不是全部内容。本文将教您如何使用PHP读取数据库中的前几条记录,并提供具体的代码示例。首先,假设您已经连接到数据库并选择了要操作的表。以下为一个简单的数据库连接示例:
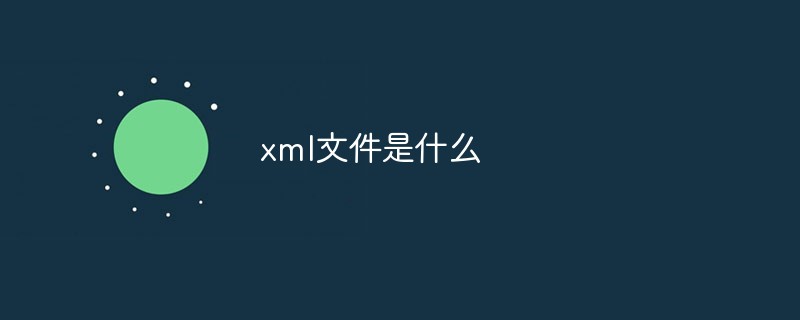
xml文件一般指里面写有可扩展标记语言的文件,XML是可扩展标记语言,标准通用标记语言的子集,是一种用于标记电子文件使其具有结构性的标记语言。
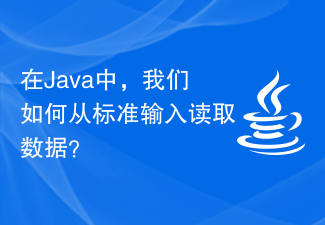
标准输入(stdin)在Java中可以用System.in来表示。System.in是InputStream类的一个实例。这意味着它的所有方法都适用于字节,而不是字符串。要从键盘读取任何数据,我们可以使用Reader类或Scanner类。示例1importjava.io.*;publicclassReadDataFromInput{ publicstaticvoidmain(String[]args){ &nbs
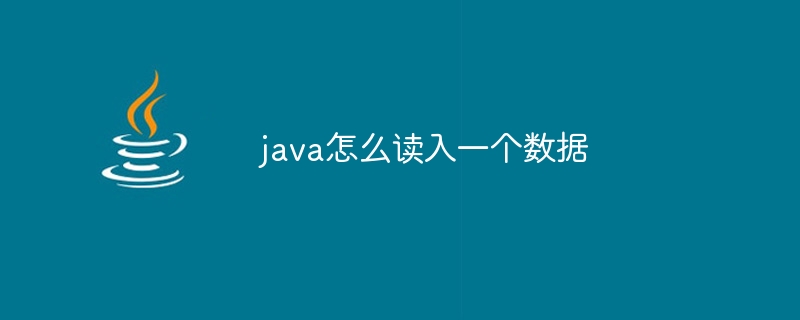
在Java中,读取数据的方式取决于数据来源和格式。常见方法包括: - **从控制台读取数据:**使用 Scanner 类读取用户输入的数据。 - **从文件中读取数据:**使用 BufferedReader 和 FileReader 类读取文本文件。对于二进制文件,可以使用 Files 和 Paths 类(Java 8及以上版本)。 - **从数据库读取数据:**使用 JDBC(Java Database Connectivity)来连接到关系型数据库并执行查询。 - **从其他来源读取数据:
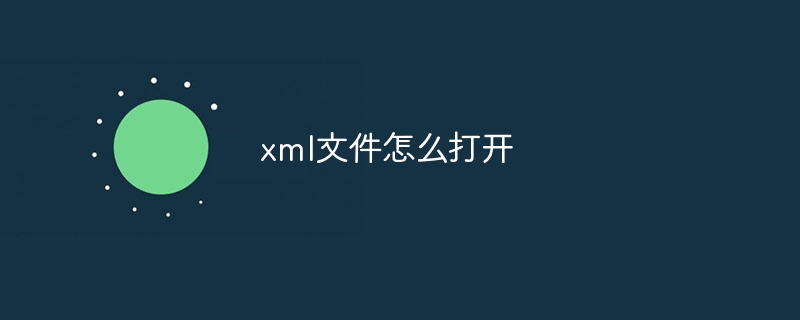
打开 XML即可扩展标记语言文件的方法是:1、在计算机上安装一个文本编辑软件;2、打开文本编辑软件,然后在菜单中选择“文件”->“打开”;3、在弹出的文件浏览器窗口中,找到要打开的 XML 文件,选中它并点击“打开”;4、系统将使用您选择的文本编辑软件打开 XML 文件,并显示其内容。
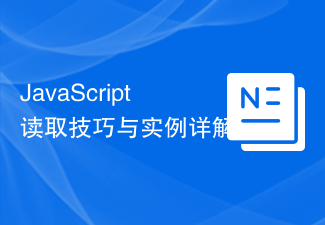
JavaScript是一种广泛应用于网页开发中的编程语言,它具有许多强大的功能和灵活性,使得开发者能够实现各种交互效果和动态功能。在日常的开发过程中,经常需要从页面中读取数据,操作元素或执行其他操作。本文将详细介绍JavaScript中的一些读取技巧,并给出详细的实例代码。1.通过id获取元素在JavaScript中,可以通过元素的id属性来获取页面中的特


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
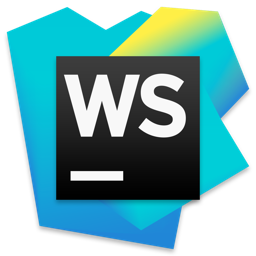
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
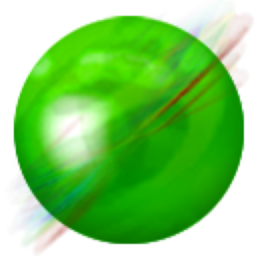
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
