


Deserted Saturday - PHP object-oriented (3), Saturday - PHP object-oriented_PHP tutorial
Desolate Saturday-PHP object-oriented (3), Saturday-php object-oriented
hi
It’s Kaizen Saturday again. The clothes I had accumulated for two weeks were finally almost finished. Come here to learn something in the afternoon~~
1. PHP object-oriented (3)
4. Advanced Practice of OOP
4.3 Static-static members
date_default_timezone_set("PRC");
/**
* 1. The definition of a class starts with the class keyword, followed by the name of the class. Class names are usually named with the first letter of each word capitalized.
* 2. Define the attributes of the class
* 3. Define the methods of the class
* 4. Instantiate the object of the class
* 5. Use the attributes and methods of the object
*/
class Human{
public $name;
public $height;
public $weight;
public function eat($food){
echo $this-> name."'s eating ".$food."
";
}
}
class Animal{
public $kind;
public $gender;
}
class NbaPlayer extends Human{
// Definition of class attributes
public $name="Jordan"; // Define attributes
public $height="198cm";
public $weight="98kg";
public $team="Bull";
public $playerNumber="23";
private $age="44";
public $president="David Stern";
// Definition of class method
public function changePresident($newP){
$this->president=$newP;
}
public function run() {
echo "Running
";
}
public function jump(){
echo "Jumping
";
}
public function dribble(){
echo "Dribbling
" ;
}
public function shoot(){
echo "Shooting
";
}
public function dunk(){
echo "Dunking
";
}
public function pass(){
echo "Passing
";
}
public function pass getAge(){
echo $this->name."'s age is ".$this->age;
}
function __construct($name, $height, $weight , $team, $playerNumber){
print $name . ";" . $height . ";" . $weight . ";" . $team . ";" . $playerNumber."n";
$this->name = $name; // $this is a pseudo variable in php, representing the object itself
$this->height = $height; // $this can be used to set the attribute value of the object
$this->weight = $weight;
$this->team = $team;
$this->playerNumber = $playerNumber;
}
}
/**
* 1. Use the new keyword when instantiating a class into an object, followed by new followed by the name of the class and a pair of parentheses.
* 2. Using objects can perform assignment operations just like using other values
*/
$jordan = new NbaPlayer("Jordan", "198cm","98kg","Bull","23");echo "
";
$james=new NbaPlayer("James", "203cm", "120kg", "Heat", "6");echo "
";
// Visit The syntax used for the properties of an object is the -> symbol, followed by the name of the property
echo $jordan->name."
";
// Used to call a method of the object The syntax is the -> symbol, followed by the name of the method and a pair of brackets
$jordan->run();
$jordan->pass();
//The subclass calls the parent class The method
$jordan->eat("apple");
//Try to call private, directly and through the internal public function
//$jordan->age;
$ jordan->getAge();echo "
";
$jordan->changePresident("Adam Silver");
echo $jordan->president."
";
echo $james->president."";
Let’s start directly from the above example.
What you want to achieve here is, change a certain variable of the two objects at the same time. ——Use static
public static $president="David Stern";
// Definition of class method
public static function changePresident($newP){
static::$president=$newP;//Replacing static with self here is more in line with the specification
}
Pay attention to the position of static here, and the ::
in the methodThe calling method has also changed.
echo NbaPlayer::$president;echo "
";
NbaPlayer::changePresident("Adam Silver");
echo NbaPlayer::$president;echo "
";
That is, as mentioned before, a static member is a constant, so it is not targeted at a specific object (not restricted by a specific object) - Based on this, definition, assignment, and call are not Requires specific target participation.
For internal calls, use self/static::$...
External call, class name::
The purpose is data shared by all objects.
--if the variable is in the parent class when called internally
For example, in the above example, write this sentence in the parent class human
public static $aaa="dafdfa";
Then in the subclass nbaplayer, when calling the static members of the parent class,
echo parent::$aaa;
For external calls, as mentioned above, class name::, so just use the parent class name directly
echo Human::$aaa;
--Others
In a static method, you cannot access other variables, that is, you cannot use $this->
--Summary
/**
* Static members
* 1. Static properties are used to save the public data of the class
* 2. Only static properties can be accessed in static methods
* 3. Static members do not need to instantiate objects. Access
* 4. Within a class, you can access its own static members through the self or static keyword
* 5. You can access the static members of the parent class through the parent keyword
* 6. You can access it through the class name External access to static members of a class
*/
4.4 Final members
--Question
Don’t want a class to have subclasses;
Don’t want the subclass to modify a variable in the parent class (avoid overriding?)
--final
》=php5 version
Give me an example
class BaseClass{
public function test(){
echo "BaseClass::test called
";
}
public function test1(){
echo "BaseClass::test1 called
";
}
}
class ChildClass extends BaseClass{
public function test(){
echo "ChildClass::test called
";
}
}
$obj=new ChildClass();
$obj->test();
Writing the same method name in the subclass as in the parent class (the content can be different) will complete the rewriting of the parent class method!
Therefore, for methods in the parent class that you do not want to be overridden, write final
final public function test(){
And so on, for a parent class that does not want to have subclasses, write final
in the class namefinal class BaseClass{
--Summary
/**
* Rewriting and Final
* 1. Writing a method in the subclass that is exactly the same as the parent class can complete the rewriting of the parent class method
* 2. For classes that do not want to be inherited by any class, you can Add the final keyword
before class * 3. For methods that do not want to be overwritten (overwrite, modified) by subclasses, you can add the final keyword
in front of the method definition.*/
4.5 Data Access
Remove the final first
--parent
Then write
in the method in the subclassparent::test();
After running, you will find that you can still call the parent class through the parent keyword, even if it is rewritten data
--self
Then write
in the method test in the parent classself::test1();
After running, it was found that self can call data in the same class (other methods/static variables/constants)
--Summary
/**
* Data access supplement
* 1. The parent keyword can be used to call the overridden class members of the parent class
* 2. The self keyword can be used to access the member methods of the class itself, or it can be used It is used to access its own static members and class constants; it cannot be used to access the properties of the class itself; when accessing class constants, there is no need to add the $ symbol in front of the constant name
* 3. The static keyword is used to access static members defined by the class itself. When accessing static properties, you need to add the $ symbol
in front of the property name.*/
4.6 Object Interface
Very important! ! !
--Question
Different classes have the same behavior, but the same behavior has different implementation methods.
For example, both humans and animals eat, but the ways of eating are different.
--Definition
Interface defines the common behaviors of different classes, and then implements different functions in different classes.
--Chestnut
//Define an interface
interface ICanEat{
public function eat($food);
}
As you can see, there is no specific implementation of the method in the interface, but there must be a method!
So, here it is, "Humans can eat"
//Specific object, connected to the interface
class Human implements ICanEat{
public function eat($food){
echo "Human eating ".$food .".
";
}
}
$obj=new Human();
$obj->eat("shit");
Please ignore the "food" I gave.
Note that does not use extends anymore, but implements. Then, the same method name is exactly the same. Then the object must/preferably implement the method.
Continue
interface ICanEat{
public function eat($food);
}
//Specific object, connected to the interface
class Human implements ICanEat{
public function eat($food){
echo "Human eating ".$food.".
";
}
}
class Animal implements ICanEat{
public function eat($food){
echo "Animal eating ".$food.".
";
}
}
$obj=new Human();
$obj->eat("shit");
$monkey=new Animal();
$monkey->eat("banana");
Let the animals eat too!
--Reverse operation
Determine whether an object is connected to an interface.
var_dump($obj instanceof ICanEat);
will return a boolean value.
--More chestnuts
interface ICanPee extends ICanEat{
public function pee();
}
class Demon implements ICanPee{
public function pee(){
echo "Can demon pee?";
}
public function eat($food){
echo "Can demon eat ".$food;
}
}
$ghost=new Demon();
$ghost->pee();
$ghost->eat("shit");
Interfaces are essentially classes and can be inherited/inherited. However, when using an inherited interface, all methods of the parent class and "grandfather class" must have specific implementations.
--Summary
/**
* Interface
* 1. The basic concepts and basic usage of interfaces
* 2. The methods in the interface have no specific implementation
* 3. The class that implements an interface must provide the interface Defined methods
* 4. You cannot use interfaces to create objects, but you can determine whether an object implements an interface
* 5. Interfaces can inherit interfaces (interface extends interface)
* 6. In interfaces All methods defined must be public, which is a characteristic of interfaces.
*/
aaaaaaaaaaaaaa
bu xiang xie le......................
ming tian yao ge............
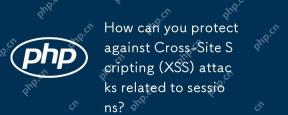
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
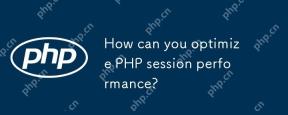
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
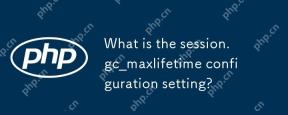
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.
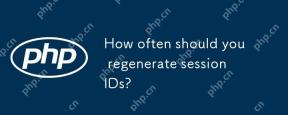
The session ID should be regenerated regularly at login, before sensitive operations, and every 30 minutes. 1. Regenerate the session ID when logging in to prevent session fixed attacks. 2. Regenerate before sensitive operations to improve safety. 3. Regular regeneration reduces long-term utilization risks, but the user experience needs to be weighed.
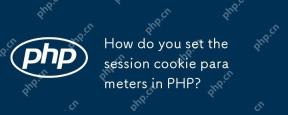
Setting session cookie parameters in PHP can be achieved through the session_set_cookie_params() function. 1) Use this function to set parameters, such as expiration time, path, domain name, security flag, etc.; 2) Call session_start() to make the parameters take effect; 3) Dynamically adjust parameters according to needs, such as user login status; 4) Pay attention to setting secure and httponly flags to improve security.
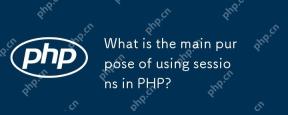
The main purpose of using sessions in PHP is to maintain the status of the user between different pages. 1) The session is started through the session_start() function, creating a unique session ID and storing it in the user cookie. 2) Session data is saved on the server, allowing data to be passed between different requests, such as login status and shopping cart content.
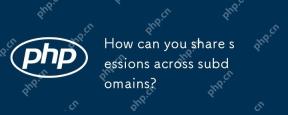
How to share a session between subdomains? Implemented by setting session cookies for common domain names. 1. Set the domain of the session cookie to .example.com on the server side. 2. Choose the appropriate session storage method, such as memory, database or distributed cache. 3. Pass the session ID through cookies, and the server retrieves and updates the session data based on the ID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),