


[PHP source code reading] explode and implode functions, explodeimplode_PHP tutorial
[PHP source code reading] explode and implode functions, explodeimplode
explode and implode functions are mainly used for conversion operations between strings and arrays, such as obtaining a parameter and then according to a certain Character splits the string, or combines the result of an array into a string output using one character. These two functions are often used in PHP, so it is necessary to understand their principles.
explode
<p>array explode ( string $delimiter, string $string, [ , $limit ] )</p>
The function returns an array composed of strings. Each element is a substring of string, separated by the string $delimiter as a boundary point.
Parameter description
limit
If $limit is set and is a positive number, the returned array contains at most $limit elements, and the last element will contain the remainder of $string.
If $limit is a negative number, return all elements except the last -$limit elements.
If $limit is 0, it will be treated as 1.
delimiter
If $delimiter is empty, the function returns FALSE. If delimiter is not in string and $limit is a negative number, an empty array is returned.
Run the example
<span>$str</span> = 'hello,world,heiheihei,php';
Let’s first look at the situation without setting a limit
<span>$arr</span> = <span>explode</span>(',', <span>$str</span><span>); </span><span>print_r</span>(<span>$arr</span>);
When limit is a positive number, limit is set to 1, and a maximum of 1 element is returned.
<span>$arr</span> = <span>explode</span>(',', <span>$str</span>, 1<span>); </span><span>print_r</span>(<span>$arr</span>);
limit is a negative number, limit is -1, and all elements except the last element are returned.
<span>$arr</span> = <span>explode</span>(',', <span>$str</span>, -1<span>); </span><span>print_r</span>(<span>$arr</span>);
limit is 0 and is treated as 1.
<span>$arr</span> = <span>explode</span>(',', <span>$str</span>, 0<span>); </span><span>print_r</span>(<span>$arr</span>);
explode execution steps
<p>1、接收参数,处理参数为空的情况</p> <p>2、创建函数中使用的局部变量</p> <p>3、根据limit的值调用不同的函数分隔字符串</p>
The core implementation of the explode function is the php_explode function. The following is the execution flow chart of this function:
if (p2 == NULL) {
// If the separator is not found, the entire string is returned directly
add_next_index_stringl(return_value, p1, Z_STRLEN_P(str), 1);
} else {
do {
// Add p1 to the return_value array
add_next_index_stringl(return_value, p1, p2 - p1, 1);
p1 = p2 Z_STRLEN_P(delim);
} while ((p2 = php_memnstr(p1, Z_STRVAL_P(delim), Z_STRLEN_P(delim), endp)) != NULL &&
--limit > 1);
// Add the last value to return_value
if (p1 endp)
add_next_index_stringl(return_value, p1, endp-p1, 1);
}
Source code interpretation
sizeof("") == 0. sizeof has two uses, sizeof(typename) and sizeof(expression). When the parameter is typename, that is, the type name, sizeof returns the size of the object corresponding to the type; when the parameter is expression expression, sizeof calculates the size of the object corresponding to the return type of the expression. Here, "" is an expression. sizeof calculates the space allocated to "" by the compiler during compilation. At this time, it must be included
If limit is not set, the default value of limit isLONG_MAX. In the php.h file, LONG_MAX is defined as 2147483647L.
In the implementation, if the limit is greater than 1, thephp_explode function is called; if the limit is less than 0, the php_explode_negative_limit function is called; if the limit is equal to 0, it is treated as 1. At this time, call the add_index_stringl function to add str to the array return_value.
When searching for the delimiter delimiter, thephp_memnstr function was called
php_memnstr(Z_STRVAL_P(str), Z_STRVAL_P(delim), Z_STRLEN_P(delim), endp); And php_memnstr is the macro definition of
zend_memnstr, which is implemented in zend_memnstr, so memchr in C is actually called to find the character delimiter.
add_next_index_stringl function to insert the separated string into the return array.
implode<p>string implode ( string $glue, array $pieces )<br />string implode ( array $pieces )</p>Convert the value of a one-dimensional array to a string
Parameter description
The implode function can receive two parameter orders. Also, if the first parameter is an array and the second parameter is empty, the second parameter is the default value ''. This function can be seen as the reverse process of explode.
Of course, use the documented order to avoid confusion.
Run the example
<span>$arr</span> = <span>array</span>('hello', 'world');
Parameters in document order
<span>$str</span> = <span>implode</span>('-‘, $arr);// 输出"hello-world"
The first parameter is an array
<span>$str</span> = <span>implode</span>(<span>$arr</span><span>); // 输出"helloworld" </span><span>$str</span> = <span>implode</span>(<span>$arr</span>, '-'); // 输出"hello-world"
implode执行步骤
<p>1、接收参数并赋值<br />2、如果第二个参数为空,则判断第一个参数的类型是否为数组,如果不是,则报错。否则,则使用""对glue赋值,使用其作为连接符。<br />3、如果第二个参数不为空,那么,如果第一个参数是数组类型,则将第二个参数转换成字符串类型;否则,如果第二个参数是数组类型,则将第一个参数转换成字符串类型。<br />4、调用php_implode函数做字符串的连接。</p>
在implode函数设置完参数之后,底层就调用php_implode函数进行字符串连接,php_implode函数的执行流程图如下:
// 遍历数组的每一个元素,判断其类型,然后调用smart_str_appendl函数将值追加到字符串中
while (zend_hash_get_current_data_ex(Z_ARRVAL_P(arr), (void **) &tmp, &pos) == SUCCESS) {
switch ((*tmp)->type) {
case IS_STRING:
smart_str_appendl(&implstr, Z_STRVAL_PP(tmp), Z_STRLEN_PP(tmp));
break;
case IS_LONG: {
char stmp[MAX_LENGTH_OF_LONG + 1];
str_len = slprintf(stmp, sizeof(stmp), "%ld", Z_LVAL_PP(tmp));
smart_str_appendl(&implstr, stmp, str_len);
}
break;
case IS_BOOL:
if (Z_LVAL_PP(tmp) == 1) {
smart_str_appendl(&implstr, "1", sizeof("1")-1);
}
break;
case IS_NULL:
break;
case IS_DOUBLE: {
char *stmp;
str_len = spprintf(&stmp, 0, "%.*G", (int) EG(precision), Z_DVAL_PP(tmp));
smart_str_appendl(&implstr, stmp, str_len);
efree(stmp);
}
break;
case IS_OBJECT: {
int copy;
zval expr;
zend_make_printable_zval(*tmp, &expr, ©);
smart_str_appendl(&implstr, Z_STRVAL(expr), Z_STRLEN(expr));
if (copy) {
zval_dtor(&expr);
}
}
break;
default:
tmp_val = **tmp;
zval_copy_ctor(&tmp_val);
convert_to_string(&tmp_val);
smart_str_appendl(&implstr, Z_STRVAL(tmp_val), Z_STRLEN(tmp_val));
zval_dtor(&tmp_val);
break;
}
// 添加glue字符
if (++i != numelems) {
smart_str_appendl(&implstr, Z_STRVAL_P(delim), Z_STRLEN_P(delim));
}
zend_hash_move_forward_ex(Z_ARRVAL_P(arr), &pos);
}
// 在尾部添加结束字符0
smart_str_0(&implstr);
源码解读
php_implode会逐个获取数组里面的内容,然后判断每个元素的类型,再做必要的数据类型转换之后,调用smart_str_appendl函数将值追加到返回的字符串后面。最后,还要在字符串后面加上结束符,这是个必须的操作,以后编程时也应注意。
smart_str_appendl是函数smart_str_appendl_ex的宏定义,该函数调用了memcpy做字符串的复制。
小结
原创文章,文笔有限,才疏学浅,文中若有不正之处,万望告知。
暂且写这么多吧,还有更多的优化和PHP源码中常用的函数,将会在以后的源码阅读中慢慢讲述。
如果本文对你有帮助,请点下推荐吧,谢谢^_^
最后,我在github有对PHP源码更详细的注解。感兴趣的可以围观一下,给个star。PHP5.4源码注解。
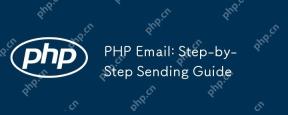
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
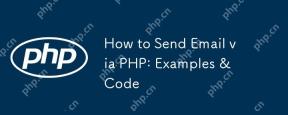
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
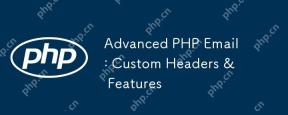
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
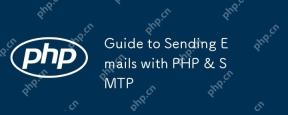
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
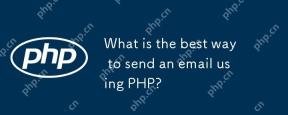
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
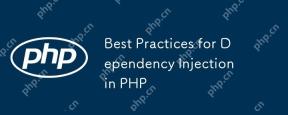
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
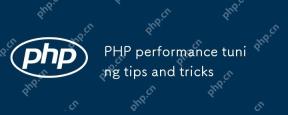
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
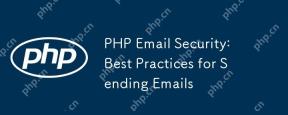
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
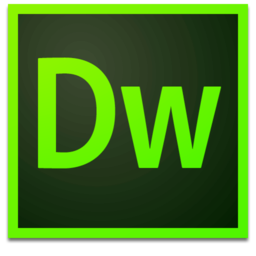
Dreamweaver Mac version
Visual web development tools
