Migrations in Laravel manage database schema, while models handle data interaction. 1) Migrations act as blueprints for database structure, allowing creation, modification, and deletion of tables. 2) Models represent data and provide an interface for interaction, enabling CRUD operations and defining relationships between tables.
When diving into the world of Laravel, understanding the difference between migrations and models is crucial. Let's break this down and then dive deeper into how each plays a vital role in your application.
Migrations vs. Models: A Quick Overview
Migrations in Laravel are all about managing your database schema. They're like the blueprints for your database, allowing you to create, modify, and delete tables with ease. Imagine you're building a house; migrations would be the architectural plans that define the structure.
On the other hand, models are the heart of your application's business logic. They represent the data in your database and provide an interface to interact with it. If migrations are the plans, models are the actual builders who bring the house to life, allowing you to work with the data in a meaningful way.
Diving Deeper into Migrations
Migrations are powerful tools for version control of your database schema. They help you manage changes over time, ensuring that your database evolves alongside your application. Here's a simple migration example to create a users
table:
use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateUsersTable extends Migration { public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); } public function down() { Schema::dropIfExists('users'); } }
This migration defines the structure of the users
table, including columns like id
, name
, email
, etc. The up
method is used to create the table, while the down
method is used to reverse the changes, dropping the table if needed.
One of the great things about migrations is the ability to roll back changes. If you mess up, you can easily revert to a previous state. However, this power comes with responsibility; you need to be careful about how you design your migrations to avoid conflicts and data loss.
Exploring Models
Models, conversely, are where the magic happens. They're the ORM (Object-Relational Mapping) layer that connects your database to your application. Here's a basic model for our users
table:
namespace App\Models; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; class User extends Authenticatable { use Notifiable; protected $fillable = [ 'name', 'email', 'password', ]; protected $hidden = [ 'password', 'remember_token', ]; protected $casts = [ 'email_verified_at' => 'datetime', ]; }
This model allows you to interact with the users
table as if it were a PHP object. You can create, read, update, and delete records using methods like create()
, find()
, update()
, and delete()
.
One of the advantages of models is the ease of defining relationships between tables. For example, if you have a posts
table related to users
, you can define this relationship in your User
model:
public function posts() { return $this->hasMany(Post::class); }
This way, you can easily fetch all posts for a user with $user->posts
.
Practical Insights and Best Practices
When working with migrations, always think about future changes. It's tempting to cram everything into one migration, but smaller, focused migrations are easier to manage and revert. Also, be cautious with foreign key constraints; they can complicate rollbacks.
For models, remember that they're not just for CRUD operations. Use them to encapsulate business logic and validation rules. For instance, you might add a method to your User
model to check if a user can perform a certain action:
public function canEditPost(Post $post) { return $this->id === $post->user_id; }
This approach keeps your logic organized and makes your application more maintainable.
Performance and Pitfalls
Migrations can be a performance bottleneck if not managed correctly. Running a large number of migrations on every deployment can slow down your CI/CD pipeline. Consider using Laravel's migrate:fresh
command sparingly and perhaps use migrate:refresh
with a specific migration to speed things up.
For models, watch out for the N 1 query problem. If you're not careful, you might end up with a lot of unnecessary database queries. Use eager loading to fetch related data efficiently:
$users = User::with('posts')->get();
This will load all posts for the users in a single query, rather than one query per user.
Conclusion
In essence, migrations and models serve different but complementary purposes in Laravel. Migrations are your database schema's version control, while models are your gateway to interact with and manipulate that data. Understanding and using both effectively can significantly enhance your development workflow and the robustness of your application. Keep experimenting, and don't be afraid to dive into the Laravel documentation for more advanced techniques and best practices.
The above is the detailed content of Laravel: What is the difference between migration and model?. For more information, please follow other related articles on the PHP Chinese website!

MigrationsinLaravelmanagedatabaseschema,whilemodelshandledatainteraction.1)Migrationsactasblueprintsfordatabasestructure,allowingcreation,modification,anddeletionoftables.2)Modelsrepresentdataandprovideaninterfaceforinteraction,enablingCRUDoperations

SoftdeletesinLaravelarebetterformaintaininghistoricaldataandrecoverability,whilephysicaldeletesarepreferablefordataminimizationandprivacy.1)SoftdeletesusetheSoftDeletestrait,allowingrecordrestorationandaudittrails,butmayincreasedatabasesize.2)Physica

SoftdeletesinLaravelareafeaturethatallowsyoutomarkrecordsasdeletedwithoutremovingthemfromthedatabase.Toimplementsoftdeletes:1)AddtheSoftDeletestraittoyourmodelandincludethedeleted_atcolumn.2)Usethedeletemethodtosetthedeleted_attimestamp.3)Retrieveall

LaravelMigrationsareeffectiveduetotheirversioncontrolandreversibility,streamliningdatabasemanagementinwebdevelopment.1)TheyencapsulateschemachangesinPHPclasses,allowingeasyrollbacks.2)Migrationstrackexecutioninalogtable,preventingduplicateruns.3)They

Laravelmigrationsarebestwhenfollowingthesepractices:1)Useclear,descriptivenamingformigrations,like'AddEmailToUsersTable'.2)Ensuremigrationsarereversiblewitha'down'method.3)Considerthebroaderimpactondataintegrityandfunctionality.4)Optimizeperformanceb
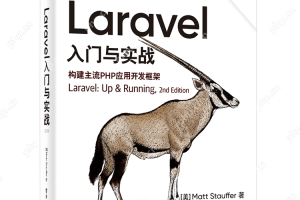
Single-page applications (SPAs) can be built using Laravel and Vue.js. 1) Define API routing and controller in Laravel to process data logic. 2) Create a componentized front-end in Vue.js to realize user interface and data interaction. 3) Configure CORS and use axios for data interaction. 4) Use VueRouter to implement routing management and improve user experience.
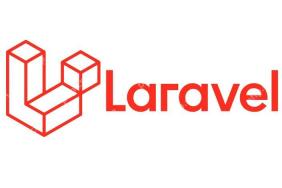
The steps to create a custom helper function in Laravel are: 1. Add an automatic loading configuration in composer.json; 2. Run composerdump-autoload to update the automatic loader; 3. Create and define functions in the app/Helpers directory. These functions can simplify code, improve readability and maintainability, but pay attention to naming conflicts and testability.
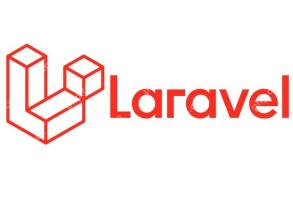
When handling database transactions in Laravel, you should use the DB::transaction method and pay attention to the following points: 1. Use lockForUpdate() to lock records; 2. Use the try-catch block to handle exceptions and manually roll back or commit transactions when needed; 3. Consider the performance of the transaction and shorten execution time; 4. Avoid deadlocks, you can use the attempts parameter to retry the transaction. This summary fully summarizes how to handle transactions gracefully in Laravel and refines the core points and best practices in the article.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
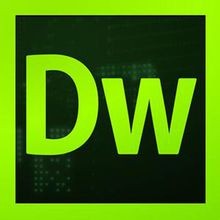
Dreamweaver CS6
Visual web development tools
