In Python, a "list" is a versatile, mutable sequence that can hold mixed data types, while an "array" is a more memory-efficient, homogeneous sequence requiring elements of the same type. 1) Lists are ideal for diverse data storage and manipulation due to their flexibility. 2) Arrays are better for large datasets needing memory optimization and for numerical operations in scientific computing.
When diving into Python, understanding the nuances between "array" and "list" is crucial for any developer looking to harness the full power of the language. Let's unpack these concepts, explore their practical applications, and share some insights from my own coding journey.
In Python, a "list" is a versatile, mutable sequence type that can hold elements of different data types. It's like a Swiss Army knife for data storage—incredibly flexible and widely used. You can think of lists as the go-to container in Python, perfect for everything from simple data collections to complex data structures. For instance, here's how you might create and manipulate a list:
# Creating a list with mixed data types my_list = [1, 'hello', 3.14, True] # Appending an element my_list.append('world') # Accessing an element print(my_list[1]) # Output: hello # Slicing the list print(my_list[1:3]) # Output: ['hello', 3.14]
On the other hand, an "array" in Python is a bit of a misnomer if you're coming from languages like C or Java. Python's array
module provides a more memory-efficient way to store a sequence of elements, but it's still not as commonly used as lists. Arrays in Python are homogeneous; they require all elements to be of the same type. Here's a quick look at how you might use an array:
from array import array # Creating an array of integers my_array = array('i', [1, 2, 3, 4, 5]) # Appending an element my_array.append(6) # Accessing an element print(my_array[0]) # Output: 1 # Getting the length of the array print(len(my_array)) # Output: 6
Now, let's dive deeper into why you might choose one over the other and share some real-world insights.
Lists are incredibly powerful because of their flexibility. They can grow or shrink dynamically, and you can mix and match data types within them. This makes them ideal for scenarios where you need to store and manipulate diverse data. For example, in my projects, I often use lists to manage data structures like stacks or queues, or to handle data from APIs where the response might contain various types of information.
However, this flexibility comes at a cost. Lists in Python are not as memory-efficient as arrays because they store pointers to objects rather than the objects themselves. If you're working with large datasets and need to optimize for memory, arrays might be a better choice. I once worked on a project that required processing millions of integers. Switching from a list to an array significantly reduced the memory footprint, which was critical for the application's performance.
Another consideration is performance. While lists are generally faster for operations like appending or inserting elements, arrays can be faster for numerical operations due to their homogeneous nature. This is particularly important in scientific computing or data analysis tasks. I've found that using arrays from the numpy
library, which is built on top of Python's array, can dramatically improve performance in these scenarios.
When choosing between lists and arrays, it's also worth considering the context of your project. Lists are part of Python's core language, making them universally accessible and easy to use. Arrays, on the other hand, require importing a module, which might be a minor inconvenience but can affect readability and maintainability.
In terms of best practices, always consider the trade-offs. If you're working with a small dataset and need flexibility, lists are your friend. But if you're dealing with large amounts of homogeneous data and need to optimize for memory or performance, arrays might be the better choice. From my experience, it's also crucial to document your choice clearly, especially in collaborative projects, so that other developers understand the reasoning behind your data structure selection.
To wrap up, understanding the difference between lists and arrays in Python is more than just a technical detail—it's about making informed decisions that can impact the performance, readability, and maintainability of your code. Whether you're a beginner or a seasoned developer, mastering these concepts will undoubtedly enhance your Python toolkit.
The above is the detailed content of Define 'array' and 'list' in the context of Python.. For more information, please follow other related articles on the PHP Chinese website!
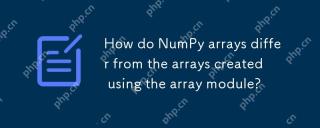
NumPyarraysarebetterfornumericaloperationsandmulti-dimensionaldata,whilethearraymoduleissuitableforbasic,memory-efficientarrays.1)NumPyexcelsinperformanceandfunctionalityforlargedatasetsandcomplexoperations.2)Thearraymoduleismorememory-efficientandfa
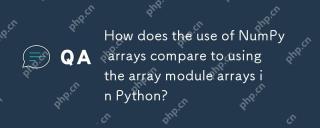
NumPyarraysarebetterforheavynumericalcomputing,whilethearraymoduleismoresuitableformemory-constrainedprojectswithsimpledatatypes.1)NumPyarraysofferversatilityandperformanceforlargedatasetsandcomplexoperations.2)Thearraymoduleislightweightandmemory-ef
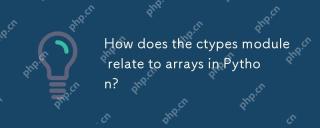
ctypesallowscreatingandmanipulatingC-stylearraysinPython.1)UsectypestointerfacewithClibrariesforperformance.2)CreateC-stylearraysfornumericalcomputations.3)PassarraystoCfunctionsforefficientoperations.However,becautiousofmemorymanagement,performanceo
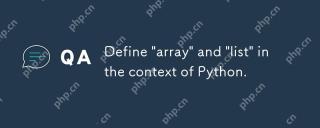
InPython,a"list"isaversatile,mutablesequencethatcanholdmixeddatatypes,whilean"array"isamorememory-efficient,homogeneoussequencerequiringelementsofthesametype.1)Listsareidealfordiversedatastorageandmanipulationduetotheirflexibility
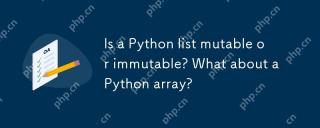
Pythonlistsandarraysarebothmutable.1)Listsareflexibleandsupportheterogeneousdatabutarelessmemory-efficient.2)Arraysaremorememory-efficientforhomogeneousdatabutlessversatile,requiringcorrecttypecodeusagetoavoiderrors.
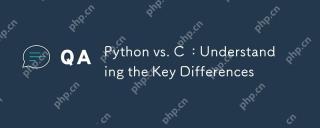
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
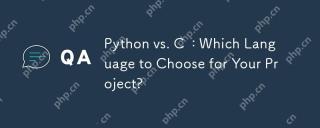
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
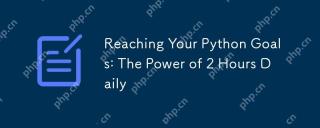
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Zend Studio 13.0.1
Powerful PHP integrated development environment
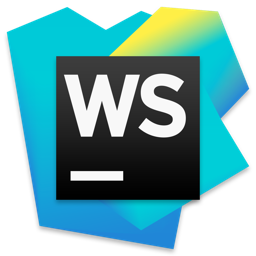
WebStorm Mac version
Useful JavaScript development tools
