


NumPy arrays are better for numerical operations and multi-dimensional data, while the array module is suitable for basic, memory-efficient arrays. 1) NumPy excels in performance and functionality for large datasets and complex operations. 2) The array module is more memory-efficient and faster to initialize but limited in functionality. 3) Choose NumPy for scientific computing and data analysis; use the array module for simple, resource-constrained applications.
NumPy arrays and arrays created using the array
module in Python serve different purposes and have distinct characteristics. Let's dive into the details to understand how they differ and when you might choose one over the other.
When I first started working with numerical computations in Python, I was intrigued by the efficiency and power of NumPy arrays. They're not just another type of array; they're a game-changer for anyone dealing with large datasets or complex mathematical operations. On the other hand, the array
module offers a more basic, lightweight solution that's perfect for certain scenarios. Let's explore these differences, and I'll share some insights from my own experience along the way.
NumPy arrays are essentially multi-dimensional arrays that are incredibly efficient for numerical operations. They're built on top of C, which means they can handle operations at a speed that's much faster than Python's native lists or the arrays from the array
module. If you're working on data analysis, machine learning, or any field where you need to crunch numbers quickly, NumPy is your go-to tool.
Here's a quick example to show how you might create and manipulate a NumPy array:
import numpy as np # Create a 2D array arr = np.array([[1, 2, 3], [4, 5, 6]]) # Perform element-wise operations result = arr * 2 print(result)
This code will output:
[[ 2 4 6] [ 8 10 12]]
Now, let's contrast this with the array
module. The array
module provides a more basic type of array that's closer to C arrays. It's useful when you need to store a homogeneous collection of basic types like integers or floats, but it doesn't support multi-dimensional arrays or the rich set of operations that NumPy offers.
Here's how you might use the array
module:
from array import array # Create an array of integers arr = array('i', [1, 2, 3, 4, 5]) # Perform a simple operation for i in range(len(arr)): arr[i] *= 2 print(arr)
This will output:
array('i', [2, 4, 6, 8, 10])
From these examples, you can see that NumPy arrays are more versatile and powerful. They support broadcasting, slicing, and a wide range of mathematical functions out of the box. However, this power comes at the cost of memory usage and initialization time, which might not be ideal for all situations.
When choosing between NumPy arrays and the array
module, consider the following:
Performance: NumPy arrays are much faster for numerical operations, especially with large datasets. The
array
module is faster for initialization and uses less memory, but it's limited in functionality.Functionality: NumPy offers a vast ecosystem of functions and tools for data manipulation and analysis. The
array
module is more basic and doesn't support multi-dimensional arrays or advanced operations.Memory Usage: If memory is a concern, the
array
module might be a better choice. NumPy arrays can be memory-intensive, especially for large datasets.Use Case: If you're working on scientific computing, data analysis, or machine learning, NumPy is the way to go. For simple, lightweight applications where you just need a basic array, the
array
module could be sufficient.
In my experience, I've found that NumPy arrays are indispensable for any serious numerical work. They've saved me countless hours of coding and debugging by providing a robust and efficient way to handle data. However, I've also used the array
module when working on embedded systems or other resource-constrained environments where every byte counts.
One pitfall to watch out for with NumPy is the potential for memory issues. If you're not careful, you can easily create arrays that are too large for your system's memory, leading to crashes or slowdowns. Always be mindful of your array sizes and consider using memory-efficient data types when possible.
On the other hand, the array
module's simplicity can sometimes be a double-edged sword. While it's easy to use, its lack of advanced features means you might find yourself writing more code to achieve what NumPy can do with a single function call.
In conclusion, the choice between NumPy arrays and the array
module depends on your specific needs. If you're diving into the world of numerical computing, NumPy will be your best friend. But if you're looking for a lightweight, basic array solution, the array
module has its place. Understanding the strengths and weaknesses of each will help you make the right decision for your projects.
The above is the detailed content of How do NumPy arrays differ from the arrays created using the array module?. For more information, please follow other related articles on the PHP Chinese website!
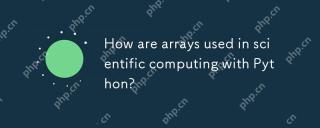
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
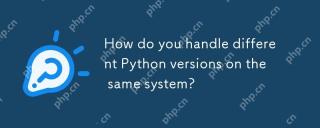
You can manage different Python versions by using pyenv, venv and Anaconda. 1) Use pyenv to manage multiple Python versions: install pyenv, set global and local versions. 2) Use venv to create a virtual environment to isolate project dependencies. 3) Use Anaconda to manage Python versions in your data science project. 4) Keep the system Python for system-level tasks. Through these tools and strategies, you can effectively manage different versions of Python to ensure the smooth running of the project.
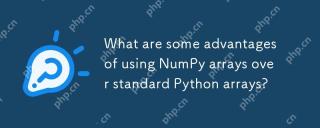
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making
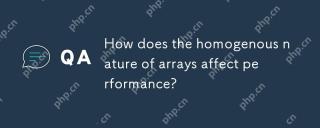
The impact of homogeneity of arrays on performance is dual: 1) Homogeneity allows the compiler to optimize memory access and improve performance; 2) but limits type diversity, which may lead to inefficiency. In short, choosing the right data structure is crucial.
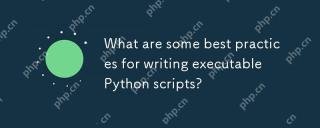
TocraftexecutablePythonscripts,followthesebestpractices:1)Addashebangline(#!/usr/bin/envpython3)tomakethescriptexecutable.2)Setpermissionswithchmod xyour_script.py.3)Organizewithacleardocstringanduseifname=="__main__":formainfunctionality.4
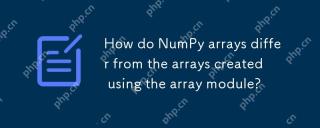
NumPyarraysarebetterfornumericaloperationsandmulti-dimensionaldata,whilethearraymoduleissuitableforbasic,memory-efficientarrays.1)NumPyexcelsinperformanceandfunctionalityforlargedatasetsandcomplexoperations.2)Thearraymoduleismorememory-efficientandfa
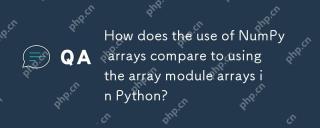
NumPyarraysarebetterforheavynumericalcomputing,whilethearraymoduleismoresuitableformemory-constrainedprojectswithsimpledatatypes.1)NumPyarraysofferversatilityandperformanceforlargedatasetsandcomplexoperations.2)Thearraymoduleislightweightandmemory-ef
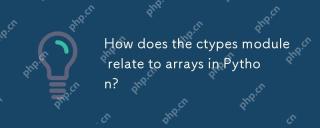
ctypesallowscreatingandmanipulatingC-stylearraysinPython.1)UsectypestointerfacewithClibrariesforperformance.2)CreateC-stylearraysfornumericalcomputations.3)PassarraystoCfunctionsforefficientoperations.However,becautiousofmemorymanagement,performanceo


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
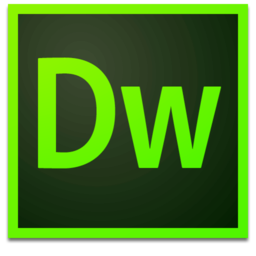
Dreamweaver Mac version
Visual web development tools
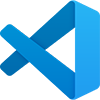
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
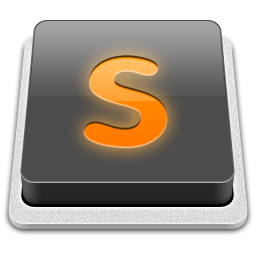
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
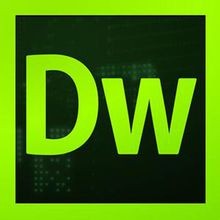
Dreamweaver CS6
Visual web development tools
