What is the purpose of mysqli_query() and mysqli_fetch_assoc()?
The mysqli_query()
function in PHP is used to execute SQL queries against a MySQL database. This function can be used to perform various types of SQL operations, such as SELECT, INSERT, UPDATE, and DELETE. When mysqli_query()
is called, it sends the SQL query to the MySQL server, and the server processes the query. The function returns a result object if the query was a SELECT statement, or TRUE/FALSE for other types of queries indicating success or failure.
On the other hand, mysqli_fetch_assoc()
is a function used to retrieve the results of a SELECT query executed by mysqli_query()
. Specifically, it returns the next row from a result set as an associative array, where the column names are the keys, and the data from the corresponding columns are the values. This function is called repeatedly until there are no more rows to fetch, at which point it will return NULL.
How does mysqli_query() differ from mysqli_fetch_assoc() in their functionality?
The primary difference between mysqli_query()
and mysqli_fetch_assoc()
lies in their specific roles within the process of interacting with a MySQL database.
mysqli_query()
is responsible for executing SQL commands. It sends the SQL query to the MySQL server and handles the communication aspect. Whether it's retrieving data, updating records, inserting new data, or deleting data, mysqli_query()
acts as the gateway through which these commands are executed. It returns either a result object for queries that fetch data, or a boolean value indicating the success or failure of the operation for queries that do not fetch data.
In contrast, mysqli_fetch_assoc()
does not execute queries but instead processes the results of a SELECT query that was executed by mysqli_query()
. It takes the result object returned by mysqli_query()
and retrieves the data row by row in the form of an associative array. This function is used to iterate through the results of a query and access data in a structured manner.
Can you explain a practical example of using mysqli_query() and mysqli_fetch_assoc() together in a PHP script?
Let's consider an example where we want to fetch all user records from a database table named users
and display them on a webpage. Here’s how you would use mysqli_query()
and mysqli_fetch_assoc()
together in a PHP script:
<?php // Assuming the connection to the database has already been established and stored in $conn // Execute the query to fetch all users $result = mysqli_query($conn, "SELECT id, username, email FROM users"); // Check if the query was successful if ($result) { // Start HTML table output echo "<table border='1'> <tr> <th>ID</th> <th>Username</th> <th>Email</th> </tr>"; // Iterate through the result set while ($row = mysqli_fetch_assoc($result)) { echo "<tr>"; echo "<td>" . $row['id'] . "</td>"; echo "<td>" . $row['username'] . "</td>"; echo "<td>" . $row['email'] . "</td>"; echo "</tr>"; } // Close the table echo "</table>"; } else { // Output error message echo "Error: " . mysqli_error($conn); } // Close the database connection mysqli_close($conn); ?>
In this example, mysqli_query()
is used to execute a SELECT query to fetch data from the users
table. The result of this query is then processed using mysqli_fetch_assoc()
in a loop, which fetches each row one by one as an associative array. The data from these rows is used to build an HTML table for display.
What are the benefits of using mysqli_fetch_assoc() over other mysqli_fetch functions?
Using mysqli_fetch_assoc()
has several benefits compared to other mysqli_fetch
functions, such as mysqli_fetch_array()
, mysqli_fetch_row()
, and mysqli_fetch_object()
:
-
Ease of Access: With
mysqli_fetch_assoc()
, you can access the data by column name (key) in the associative array. This makes the code more readable and easier to understand, especially in complex applications with many columns. -
Flexibility: Although
mysqli_fetch_array()
can return data as both associative arrays and numerically indexed arrays, usingmysqli_fetch_assoc()
specifically for associative arrays can help in maintaining a consistent approach to data handling throughout your script. - Intuitive Data Handling: Associative arrays align well with the way data is structured in databases and how it is typically processed in PHP. This can lead to more intuitive data manipulation and traversal in your scripts.
-
Reduced Complexity: By focusing on associative arrays, you don't have to deal with the additional complexity that comes with using both associative and numeric indices, as is the case with
mysqli_fetch_array()
.
Overall, mysqli_fetch_assoc()
offers a straightforward and flexible method for handling result sets from MySQL queries in PHP, which can improve both the readability and maintainability of your code.
The above is the detailed content of What is the purpose of mysqli_query() and mysqli_fetch_assoc()?. For more information, please follow other related articles on the PHP Chinese website!
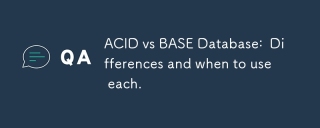
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
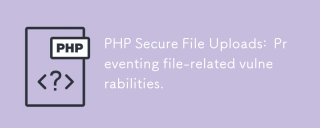
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
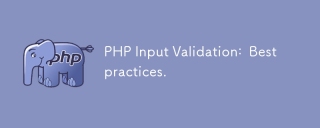
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
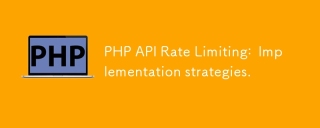
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
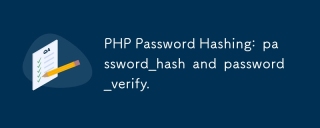
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
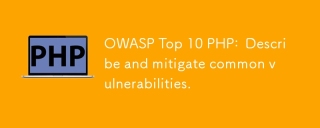
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
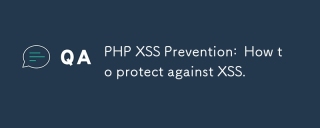
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
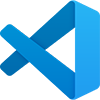
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.