This article details best practices for managing Laravel dependencies using Composer. It addresses common issues like dependency conflicts, emphasizing version constraints, regular updates (with thorough testing), and utilizing tools like Laravel S
What Are the Best Ways to Manage Dependencies and Packages in Laravel?
Laravel leverages Composer, a powerful PHP dependency manager, to handle packages and dependencies. Effectively managing these is crucial for maintainability and project health. Here's a breakdown of best practices:
-
Use Composer's
require
command: This is the primary way to add new packages. For example,composer require vendor/package
will install the specified package and add it to yourcomposer.json
file. Always specify a version constraint (e.g.,^2.0
for version 2.x) to avoid unexpected updates. -
Maintain a well-structured
composer.json
file: This file is the heart of your dependency management. Keep it clean, organized, and up-to-date. Understand the difference betweenrequire
(dependencies your application needs) andrequire-dev
(dependencies only needed for development). -
Utilize Composer's autoloading: Laravel uses Composer's autoloading capabilities to automatically load classes from your packages. This eliminates the need for manual
require
statements. Ensure your packages are properly configured for autoloading. -
Regularly run
composer update
(with caution): This command updates all packages to their latest versions, respecting the version constraints specified incomposer.json
. However, it's crucial to test thoroughly after runningcomposer update
as updates can introduce breaking changes. Consider using a dedicated branch for updates and testing before merging them into your main branch. -
Employ version constraints effectively: Using semantic versioning constraints (e.g.,
^1.2
,~1.2
,1.2.x
) is vital to prevent unexpected major version bumps that could break your application. Carefully choose the appropriate constraint based on your tolerance for changes. - Use a dedicated package manager (e.g., Laravel Shift): While Composer is the core, tools like Laravel Shift can help streamline package management by providing features such as automated dependency updates, conflict resolution, and improved workflow.
How can I efficiently resolve conflicting dependencies in my Laravel project?
Dependency conflicts arise when two or more packages require different versions of the same library. Here's how to tackle them:
-
Identify the conflict: Composer will usually report conflicts during the
install
orupdate
process. Pay close attention to the error messages, which will pinpoint the conflicting packages and their required versions. -
Analyze package dependencies: Examine the
composer.json
files of the conflicting packages to understand their dependencies. Sometimes, a less strict version constraint in one package can resolve the conflict. -
Use the
composer diagnose
command: This command helps identify potential problems, including dependency conflicts. -
Manually resolve the conflict (carefully): If Composer can't automatically resolve the conflict, you might need to manually adjust version constraints in your
composer.json
file. This requires a good understanding of the involved packages and their compatibility. Always test thoroughly after making manual changes. - Consider using a dependency resolution tool: Tools like Composer itself, and dedicated package managers can often help automatically resolve conflicts by selecting compatible versions.
- Update packages: Sometimes, updating one or more of the conflicting packages to their latest versions can resolve the incompatibility. Always test after updating.
- Replace conflicting packages: If all else fails, you might need to replace one of the conflicting packages with an alternative that has better compatibility.
What are the common pitfalls to avoid when managing packages in a Laravel application?
Several common mistakes can hinder your Laravel project's health:
-
Ignoring version constraints: Using loose constraints (
*
) can lead to unexpected breaking changes during updates. Always specify appropriate version constraints. -
Neglecting regular updates: Outdated packages can introduce security vulnerabilities and compatibility issues. Regularly run
composer update
(after careful testing) and stay informed about security advisories. - Overlooking dependency conflicts: Ignoring or poorly resolving conflicts can lead to application instability and unexpected errors.
- Not testing after updates: Always thoroughly test your application after updating packages or resolving conflicts to ensure everything works correctly.
- Failing to understand package dependencies: It's essential to understand the dependencies of each package you include to avoid unexpected conflicts or issues.
- Using packages without proper vetting: Ensure you are using reputable packages from trusted sources to minimize the risk of malicious code or poorly written software.
What tools or techniques can streamline the process of updating and maintaining Laravel packages?
Several tools and techniques can help simplify the process:
- Composer: The core tool for dependency management in Laravel, it offers features for updating, resolving conflicts, and managing dependencies.
- Laravel Shift: This tool helps automate dependency updates, manages package versions, and simplifies the update process.
- Version control (Git): Using a version control system like Git is crucial for tracking changes, reverting to previous versions if needed, and collaborating effectively on updates.
- Automated testing: Implementing a robust testing suite helps ensure that updates don't introduce regressions.
- Continuous Integration/Continuous Deployment (CI/CD): CI/CD pipelines automate the testing and deployment process, making updates easier and more reliable.
- Semantic Versioning: Understanding and adhering to semantic versioning principles helps anticipate potential breaking changes during updates.
- Package managers (beyond Composer): Explore other package managers if your workflow necessitates a different approach. However, Composer remains the standard for Laravel.
The above is the detailed content of What Are the Best Ways to Manage Dependencies and Packages in Laravel?. For more information, please follow other related articles on the PHP Chinese website!

MigrationsinLaravelmanagedatabaseschema,whilemodelshandledatainteraction.1)Migrationsactasblueprintsfordatabasestructure,allowingcreation,modification,anddeletionoftables.2)Modelsrepresentdataandprovideaninterfaceforinteraction,enablingCRUDoperations

SoftdeletesinLaravelarebetterformaintaininghistoricaldataandrecoverability,whilephysicaldeletesarepreferablefordataminimizationandprivacy.1)SoftdeletesusetheSoftDeletestrait,allowingrecordrestorationandaudittrails,butmayincreasedatabasesize.2)Physica

SoftdeletesinLaravelareafeaturethatallowsyoutomarkrecordsasdeletedwithoutremovingthemfromthedatabase.Toimplementsoftdeletes:1)AddtheSoftDeletestraittoyourmodelandincludethedeleted_atcolumn.2)Usethedeletemethodtosetthedeleted_attimestamp.3)Retrieveall

LaravelMigrationsareeffectiveduetotheirversioncontrolandreversibility,streamliningdatabasemanagementinwebdevelopment.1)TheyencapsulateschemachangesinPHPclasses,allowingeasyrollbacks.2)Migrationstrackexecutioninalogtable,preventingduplicateruns.3)They

Laravelmigrationsarebestwhenfollowingthesepractices:1)Useclear,descriptivenamingformigrations,like'AddEmailToUsersTable'.2)Ensuremigrationsarereversiblewitha'down'method.3)Considerthebroaderimpactondataintegrityandfunctionality.4)Optimizeperformanceb
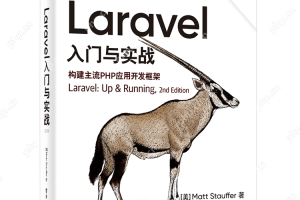
Single-page applications (SPAs) can be built using Laravel and Vue.js. 1) Define API routing and controller in Laravel to process data logic. 2) Create a componentized front-end in Vue.js to realize user interface and data interaction. 3) Configure CORS and use axios for data interaction. 4) Use VueRouter to implement routing management and improve user experience.
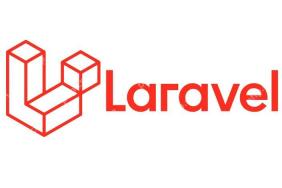
The steps to create a custom helper function in Laravel are: 1. Add an automatic loading configuration in composer.json; 2. Run composerdump-autoload to update the automatic loader; 3. Create and define functions in the app/Helpers directory. These functions can simplify code, improve readability and maintainability, but pay attention to naming conflicts and testability.
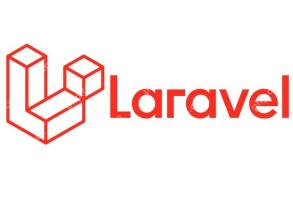
When handling database transactions in Laravel, you should use the DB::transaction method and pay attention to the following points: 1. Use lockForUpdate() to lock records; 2. Use the try-catch block to handle exceptions and manually roll back or commit transactions when needed; 3. Consider the performance of the transaction and shorten execution time; 4. Avoid deadlocks, you can use the attempts parameter to retry the transaction. This summary fully summarizes how to handle transactions gracefully in Laravel and refines the core points and best practices in the article.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
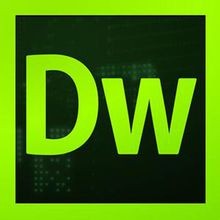
Dreamweaver CS6
Visual web development tools
