This article details implementing message queues in PHP using RabbitMQ and Redis. It compares their architectures (AMQP vs. in-memory), features, and reliability mechanisms (confirmations, transactions, persistence). Best practices for design, error
How to Implement Message Queues (RabbitMQ, Redis) in PHP?
Implementing message queues in PHP using RabbitMQ and Redis involves different approaches due to their architectural differences. RabbitMQ is a robust, feature-rich message broker implementing the AMQP protocol, while Redis offers a simpler, in-memory data store with queue functionality.
Implementing with RabbitMQ:
You'll need the php-amqplib
library. Install it using Composer: composer require php-amqplib/php-amqplib
.
Here's a basic example of sending and receiving messages:
// Sending a message $connection = new AMQPConnection([ 'host' => 'localhost', 'port' => 5672, 'login' => 'guest', 'password' => 'guest', 'vhost' => '/' ]); $channel = $connection->channel(); $channel->queue_declare('my_queue', false, false, false, false); $message = 'Hello World!'; $channel->basic_publish(new AMQPMessage($message), '', 'my_queue'); $channel->close(); $connection->close(); // Receiving a message $connection = new AMQPConnection([ 'host' => 'localhost', 'port' => 5672, 'login' => 'guest', 'password' => 'guest', 'vhost' => '/' ]); $channel = $connection->channel(); $channel->queue_declare('my_queue', false, false, false, false); $callback = function ($msg) { echo " [x] Received ", $msg->body, "\n"; $msg->delivery_info['channel']->basic_ack($msg->delivery_info['delivery_tag']); }; $channel->basic_consume('my_queue', '', false, false, false, false, $callback); while(count($channel->callbacks)) { $channel->wait(); } $channel->close(); $connection->close();
Implementing with Redis:
You'll need the predis/predis
library. Install it using Composer: composer require predis/predis
.
Here's a basic example using Redis lists as queues:
// Sending a message $redis = new Predis\Client(); $redis->rpush('my_queue', 'Hello World!'); // Receiving a message $message = $redis->lpop('my_queue'); if ($message !== null) { echo " [x] Received: " . $message . "\n"; }
What are the key differences between using RabbitMQ and Redis as message queues in a PHP application?
RabbitMQ and Redis differ significantly in their architecture and features, impacting their suitability for various use cases.
Feature | RabbitMQ | Redis |
---|---|---|
Architecture | Distributed message broker, AMQP protocol | In-memory data store, simpler queueing |
Persistence | Persistent message storage (configurable) | In-memory, data lost on server restart (unless configured for persistence) |
Features | Advanced features: routing, exchanges, message prioritization, guaranteed delivery | Simpler queueing, no advanced routing |
Scalability | Highly scalable, handles high message volume | Scalable but may face limitations at very high throughput |
Complexity | More complex to set up and manage | Easier to set up and use |
Use Cases | Complex, distributed systems, requiring high reliability and advanced features | Simpler applications, where message ordering isn't critical, and data loss is acceptable |
How can I ensure reliable message delivery and handling when using message queues with PHP?
Reliable message delivery and handling are crucial for preventing data loss and ensuring application integrity. Here's how to achieve it with RabbitMQ and Redis:
RabbitMQ:
-
Confirmations: Use publisher confirms (
$channel->confirm_select(1);
) to ensure messages are acknowledged by the broker. - Transactions: Use transactions to guarantee atomicity of message publishing and other operations.
-
Persistent Queues and Messages: Declare queues and messages as persistent (
durable
flag set totrue
). This ensures data survives broker restarts. - Dead-Letter Queues (DLQs): Configure DLQs to handle messages that fail to be processed. This allows for retry mechanisms and monitoring of failed messages.
-
ACKnowledgements: Consumers should acknowledge messages (
$msg->delivery_info['channel']->basic_ack($msg->delivery_info['delivery_tag']);
) only after successful processing. Use negative acknowledgements for failed processing to requeue the message.
Redis:
- Persistence: Configure Redis to use persistence (RDB or AOF) to prevent data loss on server restarts. This is crucial for reliable message delivery.
- Transactions (Lua Scripting): Use Lua scripting to perform atomic operations on Redis lists, ensuring data consistency.
- Retry Mechanisms: Implement retry logic in your consumer to handle temporary failures in message processing.
- Monitoring: Monitor queue lengths and processing times to identify potential bottlenecks and issues.
What are the best practices for designing and implementing a message queue system in a PHP application using RabbitMQ or Redis?
Designing and implementing a robust message queue system requires careful consideration. Here are some best practices:
- Choose the right tool: Select RabbitMQ for complex, distributed systems needing high reliability and advanced features; choose Redis for simpler applications where some data loss is acceptable.
- Clear message structure: Define a clear and consistent message format (e.g., JSON) for easy parsing and handling.
- Error handling: Implement comprehensive error handling and logging to track issues and ensure reliable operation.
- Dead-letter queues (DLQs): Utilize DLQs to handle failed messages, enabling retries and monitoring.
- Monitoring and alerting: Monitor queue lengths, processing times, and error rates to identify and address performance bottlenecks and issues proactively.
- Scalability: Design your system to scale horizontally by distributing message processing across multiple consumers.
- Rate limiting: Implement rate limiting to prevent overload on your message queue and consumers.
- Message ordering (if needed): If message order is critical, use features like RabbitMQ's exchanges and queues to guarantee it. Redis, with its simpler queueing, generally doesn't provide strong guarantees on message order.
- Testing: Thoroughly test your message queue system under various load conditions to ensure reliability and performance.
By following these best practices, you can build a reliable and efficient message queue system in your PHP application using either RabbitMQ or Redis, tailored to the specific needs of your project.
The above is the detailed content of How to Implement message queues (RabbitMQ, Redis) in PHP?. For more information, please follow other related articles on the PHP Chinese website!
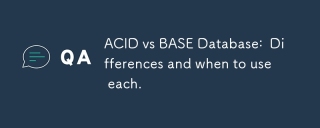
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
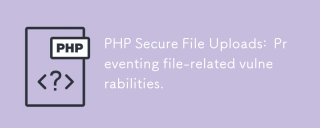
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
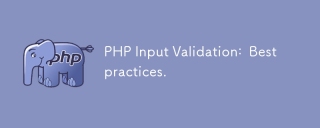
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
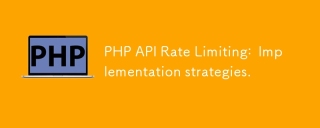
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
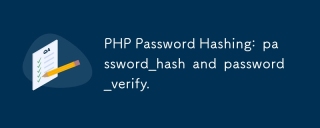
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
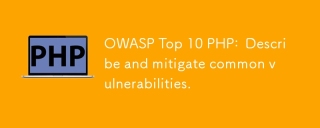
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
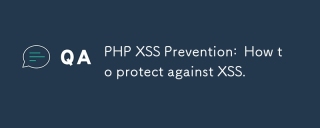
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
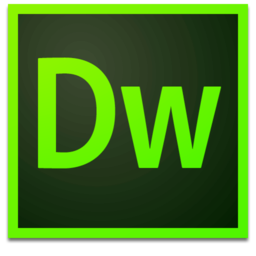
Dreamweaver Mac version
Visual web development tools
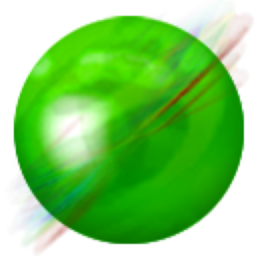
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment