This article explains PHP's Reflection API, enabling runtime inspection and manipulation of classes, methods, and properties. It details common use cases (documentation generation, ORMs, dependency injection) and cautions against performance overhea
How to Use Reflection to Analyze and Manipulate PHP Code?
Reflection in PHP allows you to inspect and manipulate the structure and behavior of classes, methods, functions, and properties at runtime. It provides a powerful way to dynamically interact with your code, enabling metaprogramming capabilities. The core of reflection lies in the Reflection
classes, which provide methods to access information about various code elements.
For example, to analyze a class, you'd use the ReflectionClass
class:
<?php $reflectionClass = new ReflectionClass('MyClass'); // Get class name echo $reflectionClass->getName() . "\n"; // Get class methods foreach ($reflectionClass->getMethods() as $method) { echo "Method: " . $method->getName() . "\n"; } // Get class properties foreach ($reflectionClass->getProperties() as $property) { echo "Property: " . $property->getName() . "\n"; } // Check if a method exists if ($reflectionClass->hasMethod('myMethod')) { echo "Method 'myMethod' exists\n"; } ?>
Similarly, ReflectionMethod
, ReflectionProperty
, and ReflectionFunction
allow you to inspect individual methods, properties, and functions respectively. You can access modifiers (public, private, protected), parameters, return types, and more. The key is to create the appropriate Reflection
object and then utilize its methods to extract the desired information. Remember to handle potential exceptions, such as ReflectionException
, which can be thrown if the reflected element doesn't exist.
What are the common use cases for reflection in PHP?
Reflection in PHP serves a variety of purposes beyond simple code analysis. Some common use cases include:
- Generating Documentation: Reflection can automatically generate documentation based on code structure. Tools can analyze classes and methods using reflection to create API documentation or developer guides.
- Building ORM (Object-Relational Mappers): ORMs often use reflection to map database tables to PHP classes and automatically handle data persistence. They dynamically inspect class properties and map them to database columns.
- Creating Dependency Injection Containers: Dependency injection frameworks leverage reflection to automatically instantiate classes and inject dependencies based on constructor parameters or property annotations.
- Implementing Dynamic Proxies: Reflection enables the creation of dynamic proxies, allowing you to intercept method calls and modify their behavior. This is useful for aspects like logging, security checks, or transaction management.
- Unit Testing: Reflection can be used in unit tests to access private or protected methods and properties for testing purposes. While generally discouraged for regular code, it's a valuable tool for thorough testing.
- Code Inspection and Analysis Tools: Static analysis tools and linters utilize reflection to examine code for potential problems, coding style violations, or security vulnerabilities.
How can I use reflection to modify the behavior of existing PHP classes at runtime?
Modifying the behavior of existing classes at runtime using reflection primarily involves using ReflectionClass
to access and manipulate properties or methods. However, direct modification of private or protected members is generally discouraged due to encapsulation concerns. Instead, consider these approaches:
-
Modifying Public Properties: You can directly access and change the values of public properties using
ReflectionProperty
:
<?php $reflectionProperty = new ReflectionProperty('MyClass', 'myPublicProperty'); $reflectionProperty->setValue($myObject, 'new value'); ?>
-
Calling Methods: You can invoke methods using
ReflectionMethod
:
<?php $reflectionMethod = new ReflectionMethod('MyClass', 'myMethod'); $result = $reflectionMethod->invoke($myObject, 'argument1', 'argument2'); ?>
- Using Proxies: For more complex modifications, creating a proxy class is a better approach. The proxy intercepts calls to the original class and can modify the behavior before or after the original method is executed.
- Extending Classes: While not strictly reflection, extending a class allows you to override methods and add new functionality. This is a cleaner and more maintainable way to modify behavior if possible.
Remember that directly manipulating private or protected members can lead to brittle code and break encapsulation. It's crucial to carefully consider the implications before resorting to such practices.
What are the potential performance implications of using reflection in my PHP application?
Reflection has performance overhead compared to direct method calls or property accesses. The process of creating Reflection
objects and accessing their properties involves significant processing. Therefore, overuse of reflection can negatively impact application performance.
Consider these factors:
-
Object Creation: Creating
Reflection
objects is relatively expensive. Avoid creating them repeatedly within loops or frequently called functions. -
Method Calls: Invoking methods through
ReflectionMethod::invoke()
is slower than directly calling the method. -
Caching: Cache the results of reflection operations whenever possible. If you need to repeatedly access the same information, cache the
Reflection
objects or the data extracted from them. - Alternatives: Before using reflection, consider whether simpler alternatives exist. If possible, design your code to avoid the need for runtime manipulation.
In summary, use reflection judiciously. While it provides powerful capabilities, it's crucial to be aware of its performance implications and employ optimization techniques to mitigate negative impacts on your application's speed and responsiveness. Profiling your application can help identify reflection-related performance bottlenecks.
The above is the detailed content of How to Use Reflection to Analyze and Manipulate PHP Code?. For more information, please follow other related articles on the PHP Chinese website!
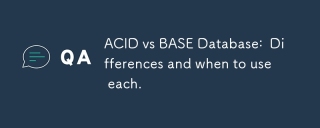
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
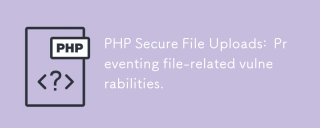
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
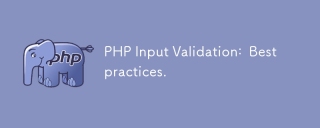
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
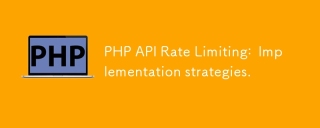
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
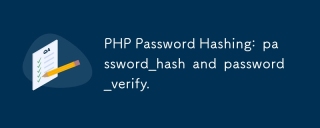
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
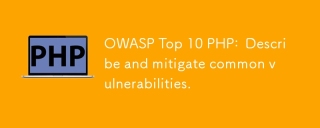
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
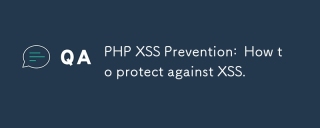
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
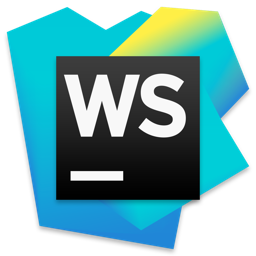
WebStorm Mac version
Useful JavaScript development tools
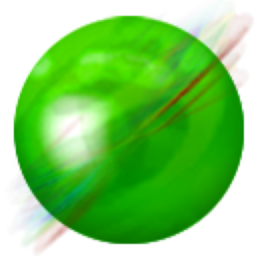
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment