


How to Apply the Factory Pattern in PHP for Flexible Object Creation?
Applying the Factory Pattern in PHP
The Factory Pattern is a creational design pattern that provides an interface for creating objects without specifying their concrete classes. In PHP, this typically involves a factory class that contains methods to create different object instances. These methods handle the object instantiation logic, abstracting away the specifics from the client code. Let's illustrate with an example creating different types of cars:
<?php interface Car { public function drive(): string; } class SportsCar implements Car { public function drive(): string { return "Driving a fast sports car!"; } } class Sedan implements Car { public function drive(): string { return "Driving a comfortable sedan."; } } class CarFactory { public static function createCar(string $type): Car { switch ($type) { case 'sports': return new SportsCar(); case 'sedan': return new Sedan(); default: throw new InvalidArgumentException("Invalid car type."); } } } // Client code $sportsCar = CarFactory::createCar('sports'); echo $sportsCar->drive() . PHP_EOL; // Output: Driving a fast sports car! $sedan = CarFactory::createCar('sedan'); echo $sedan->drive() . PHP_EOL; // Output: Driving a comfortable sedan! ?>
This example demonstrates a simple factory method. The CarFactory
class's createCar
method handles the instantiation of different Car
implementations based on the input $type
. The client code only needs to call the factory method, without needing to know the concrete classes involved. More complex scenarios might involve dependency injection within the factory methods.
What are the benefits of using the Factory Pattern over direct object instantiation in PHP?
Benefits of Using the Factory Pattern
Using the Factory Pattern offers several advantages over direct object instantiation:
- Loose Coupling: The client code doesn't depend directly on the concrete classes of the objects it uses. This makes the code more flexible and easier to maintain, as you can change the concrete classes without affecting the client code.
- Improved Code Organization: The factory class centralizes the object creation logic, making the code cleaner and more organized. This is especially beneficial in larger applications with many object types.
- Enhanced Flexibility: You can easily add new object types without modifying the client code. You simply add a new case to the factory method or extend the factory class's functionality.
- Simplified Testing: Testing becomes easier because you can easily mock or stub the factory methods during testing, isolating your tests from the complexities of object creation.
- Reduced Code Duplication: The factory method handles the instantiation logic, preventing code duplication in multiple parts of the application.
How can I handle different object types and dependencies effectively using a Factory Pattern in my PHP application?
Handling Different Object Types and Dependencies
The Factory Pattern excels at managing different object types and their dependencies. Here's how you can handle them effectively:
- Multiple Factory Methods: For a larger number of object types, consider having multiple factory methods within the factory class, each responsible for creating a specific type of object. This improves readability and organization.
- Dependency Injection: Inject dependencies into the factory methods as parameters. This allows the factory to create objects with customized configurations based on the provided dependencies.
- Abstract Factory: For more complex scenarios involving families of related objects, consider using the Abstract Factory Pattern. This pattern provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Configuration: Use configuration files (e.g., YAML, JSON) to define object types and their dependencies. The factory can then read this configuration and create the appropriate objects.
Example with Dependency Injection:
<?php interface Car { public function drive(): string; } class SportsCar implements Car { public function drive(): string { return "Driving a fast sports car!"; } } class Sedan implements Car { public function drive(): string { return "Driving a comfortable sedan."; } } class CarFactory { public static function createCar(string $type): Car { switch ($type) { case 'sports': return new SportsCar(); case 'sedan': return new Sedan(); default: throw new InvalidArgumentException("Invalid car type."); } } } // Client code $sportsCar = CarFactory::createCar('sports'); echo $sportsCar->drive() . PHP_EOL; // Output: Driving a fast sports car! $sedan = CarFactory::createCar('sedan'); echo $sedan->drive() . PHP_EOL; // Output: Driving a comfortable sedan! ?>
This enhanced example demonstrates dependency injection of an Engine
object into the Car
constructor, allowing for flexible engine choices.
Can I use the Factory Pattern in PHP to improve code maintainability and reduce code duplication when creating objects?
Improving Maintainability and Reducing Code Duplication
Yes, the Factory Pattern significantly improves code maintainability and reduces code duplication.
- Centralized Object Creation: The factory class centralizes the logic for creating objects, preventing scattered object creation code throughout the application. This reduces the risk of inconsistencies and makes it easier to manage changes.
- Easier Modification: Adding new object types or modifying existing ones requires changes only within the factory class, minimizing the impact on the rest of the application.
- Improved Readability: The code becomes more readable and understandable because object creation is abstracted away from the client code.
- Reduced Complexity: The Factory Pattern simplifies the code by decoupling object creation from the client code, reducing overall complexity and making the code easier to test and debug.
In summary, the Factory Pattern is a powerful tool in PHP for managing object creation, promoting better code organization, flexibility, maintainability, and reducing code duplication. Choosing between a simple factory, factory method, or abstract factory depends on the complexity of your object creation needs.
The above is the detailed content of How to Apply the Factory Pattern in PHP for Flexible Object Creation?. For more information, please follow other related articles on the PHP Chinese website!
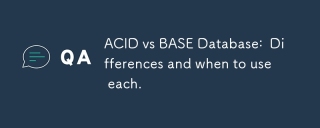
The article compares ACID and BASE database models, detailing their characteristics and appropriate use cases. ACID prioritizes data integrity and consistency, suitable for financial and e-commerce applications, while BASE focuses on availability and
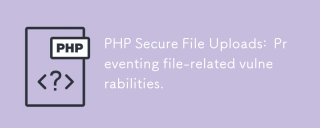
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
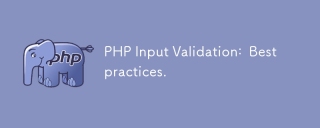
Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
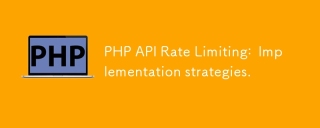
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
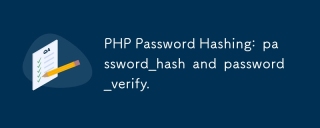
The article discusses the benefits of using password_hash and password_verify in PHP for securing passwords. The main argument is that these functions enhance password protection through automatic salt generation, strong hashing algorithms, and secur
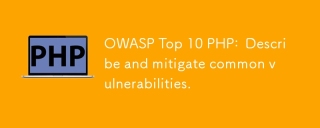
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
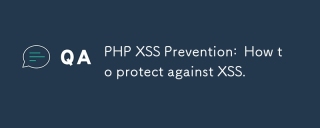
The article discusses strategies to prevent XSS attacks in PHP, focusing on input sanitization, output encoding, and using security-enhancing libraries and frameworks.

The article discusses the use of interfaces and abstract classes in PHP, focusing on when to use each. Interfaces define a contract without implementation, suitable for unrelated classes and multiple inheritance. Abstract classes provide common funct


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use
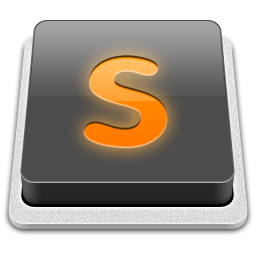
SublimeText3 Mac version
God-level code editing software (SublimeText3)