Laravel provides multiple ways to control the format of dates when the model is serialized into an array or JSON. From global formatting to customization of specific properties, you can ensure consistency in date display throughout the application.
The following is an example of setting the global date format in the base class:
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; use DateTimeInterface; class BaseModel extends Model { protected function serializeDate(DateTimeInterface $date) { return $date->format('Y-m-d H:i:s'); } }
Let's look at a practical example of managing different date formats in the booking system:
<?php namespace App\Models; use Illuminate\Database\Eloquent\Model; use Illuminate\Database\Eloquent\Casts\Attribute; use DateTimeInterface; class Booking extends Model { protected $casts = [ 'check_in' => 'datetime:Y-m-d', 'check_out' => 'datetime:Y-m-d', 'created_at' => 'datetime:Y-m-d H:i:s', ]; protected function serializeDate(DateTimeInterface $date) { return $date->format('Y-m-d H:i:s'); } protected function checkInFormatted(): Attribute { return Attribute::make( get: fn () => $this->check_in->format('l, F j, Y') ); } protected function duration(): Attribute { return Attribute::make( get: fn () => $this->check_in->diffInDays($this->check_out) ); } public function toArray() { return array_merge(parent::toArray(), [ 'check_in_formatted' => $this->checkInFormatted, 'duration_nights' => $this->duration, 'human_readable' => sprintf( '%s for %d nights', $this->check_in->format('M j'), $this->duration ) ]); } }
This example shows how to format a date for a specific property using the $casts
property, and how to create a custom accessor using the Attribute
class to generate a formatted date and duration. The toArray()
method demonstrates how to add these custom properties to the model's array representation.
Laravel's date serialization feature ensures consistency in date formats throughout the application while providing flexibility for specific use cases.
The above is the detailed content of Customizing Model Date Formats in Laravel. For more information, please follow other related articles on the PHP Chinese website!
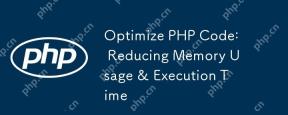
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
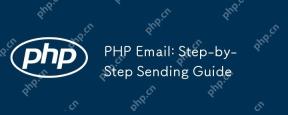
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
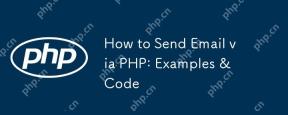
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
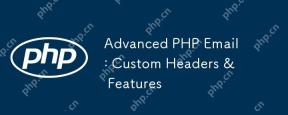
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
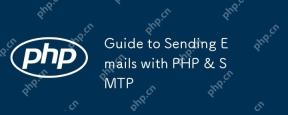
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
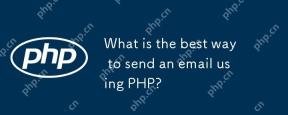
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
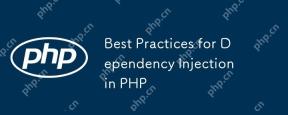
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
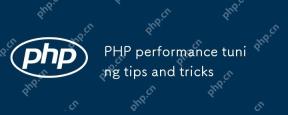
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
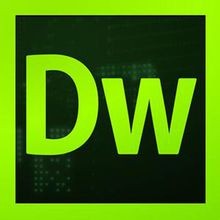
Dreamweaver CS6
Visual web development tools
