Core points
- PHP generator provides an easy way to implement an iterator without the need for a complex Iterator interface, but instead use the
yield
keyword instead ofreturn
to save its state and continue from the interrupt when called again . - Generators are very memory-saving when working with large datasets, because they only need to allocate memory for the current result, without storing all values in memory at once like an array.
- Although the generator works like an iterator, it is essentially a function that returns and receives external values by calling the
send()
method of the generator object. It can also be used in another generator, which is called a generator delegate.
If you followed my previous article on iterators, you will know that iteration is an important programming concept, but implementing the necessary interface to create iterable objects is a hassle at best because it requires a lot of boilerplate code. With the release of PHP 5.5, we finally have a generator! In this article, we will learn about the generator, which provides an easy way to implement a simple iterator without the overhead or complexity of the Iterator interface.
How the generator works
According to Wikipedia's definition, the generator is "very similar to a function that returns an array, because the generator has parameters that can be called and generates a series of values". The generator is basically a normal function, but instead of returning a value, it produces as many values as needed. It looks like a function, but behaves like an iterator. The generator uses the yield
keyword instead of return
. It works similar to return
because it returns the value to the caller of the function, but yield
does not remove the function from the stack, but saves its state. This allows the function to continue execution from the interrupt. In fact, you cannot return a value from the generator, although you can terminate its execution with return
without a value. The PHP manual states: "When a generator function is called, it returns an object that can be iterated." This is an object of the internal Generator class that implements the Iterator interface in the same way as a forward-iter object only. When you iterate over the object, PHP calls the generator every time a value is needed. When the generator generates a value, the state is saved so that it can be restored when the next value is needed.
<?php function nums() { echo "The generator has started\n"; for ($i = 0; $i < 5; $i++) { yield $i; echo "Yielded $i\n"; } echo "The generator has ended\n"; } foreach (nums() as $v); ?>
The output of the above code will be:
<code>The generator has started Yielded 0 Yielded 1 Yielded 2 Yielded 3 Yielded 4 The generator has ended</code>
Our first generator
Generator is not a new concept. It already exists in languages such as C#, Python, JavaScript, and Ruby (enumerator), and is usually recognized by using the yield
keyword. Here is a Python example:
def file_lines(filename): file = open(filename) for line in file: yield line file.close() for line in file_lines('somefile'): #do some work here
Let's rewrite the Python generator example in PHP. (Note that neither of these code snippets perform any error checking.)
<?php function nums() { echo "The generator has started\n"; for ($i = 0; $i < 5; $i++) { yield $i; echo "Yielded $i\n"; } echo "The generator has ended\n"; } foreach (nums() as $v); ?>
The generator function opens a file and then generates each line of the file as needed. Each time the generator is called, it continues to execute from the interrupt. It does not start from scratch because when the yield
statement is executed, its state is saved. Once all rows are read, the generator simply terminates and the loop ends.
Return key
PHP iterator consists of key/value pairs. In our example, only one value is returned, so the key is a number (by default the key is a number). If you want to return an associated pair, just change the yield
statement to include the keys using the array syntax.
<code>The generator has started Yielded 0 Yielded 1 Yielded 2 Yielded 3 Yielded 4 The generator has ended</code>
Injection value
yield
not only returns a value; it can also receive external values. This is done by calling the send()
method of the generator object with the value you want to pass. This value can then be used to calculate or perform other operations. This method sends the value as a result of the yield
expression to the generator and resumes execution.
def file_lines(filename): file = open(filename) for line in file: yield line file.close() for line in file_lines('somefile'): #do some work here
The output will be:
<?php function file_lines($filename) { $file = fopen($filename, 'r'); while (($line = fgets($file)) !== false) { yield $line; } fclose($file); } foreach (file_lines('somefile') as $line) { // do some work here } ?>
Save memory using generator
Generator is useful when you calculate large collections and don't want to allocate memory for all results at the same time, or when you don't know if all results are needed. Due to the way the results are processed, memory usage can be reduced to very low levels by allocating memory only for the current results. Imagine the file()
function, which returns all lines in the file as an array. Run a simple benchmark for the file()
function and our demo file_lines()
function, each using the same random 100-segment text file generated using Lipsum, and the result shows that the file
function uses at most a generator 110 times the
<?php function file_lines($filename) { // ... yield $key => $line; // ... } foreach (file_lines('somefile') as $key => $line) { // do some work here } ?>
Conclusion
With the introduction of generators, PHP provides developers with a powerful tool. We can now write iterators quickly while saving a lot of memory. Through this tutorial, I hope you have gained enough knowledge to start using them yourself in your project. Personally, I have already thought of a lot of objects to rewrite. If you have any ideas or comments, please leave your comment.
FAQs for PHP generator
(The FAQs listed in the original text should be included here, and due to space limitations, it is omitted here.)
The above is the detailed content of PHP Master | Generators in PHP. For more information, please follow other related articles on the PHP Chinese website!
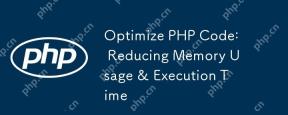
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
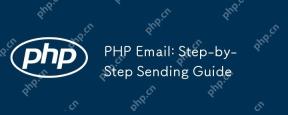
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
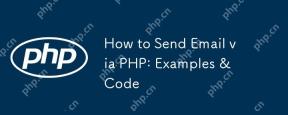
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
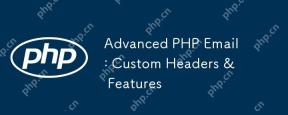
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
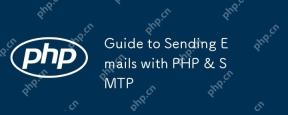
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
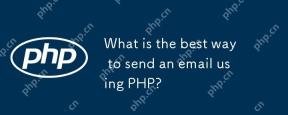
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
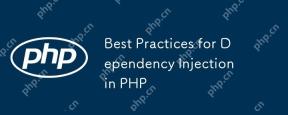
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
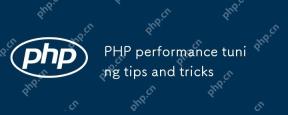
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
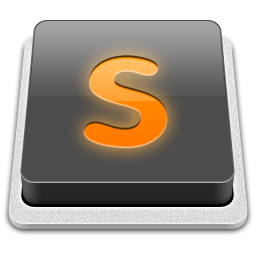
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
