This article details how to connect an Arduino to PHP via the Firmata protocol, enabling cross-language communication. It covers installing the necessary PHP serial class, establishing the Arduino connection, and crafting a PHP script for data transmission.
The guide also provides a concise introduction to Arduino programming using C and C , describing Arduinos as compact computers interfacing with various components (buttons, sensors, LEDs).
Furthermore, it explains how to leverage a Minecraft Query library in PHP to interact with a Minecraft server, retrieving server status, player information, and more for dynamic webpage creation.
This tutorial builds upon a previous installment, connecting a virtual Minecraft alarm to a PHP listener. This part focuses on constructing a physical Arduino-based alarm circuit, programming it using the official IDE and Firmata, and finally integrating it with the Minecraft circuit for a complete, real-world alarm system.
The code is available at https://www.php.cn/link/153541c160234d5f9029608e65329ad0.
Arduino Programming Essentials
Arduinos are popular hobbyist microcontroller boards (e.g., Uno, Micro). They feature input/output pins for connecting components and utilize C/C programming. Consider them simplified computers managing circuitry and providing a programmatic interface.
You'll need an Arduino board (or compatible alternative), an alarm buzzer, or LED. These components typically have ground and Arduino pin connections.
Download the official Arduino IDE from https://www.php.cn/link/cd4e9ea43d04220d6f90db0e66758a51. The primary programming languages are C and C . The setup()
function handles initial board configuration, while loop()
contains continuously executed code.
To upload code, identify the Arduino port using a terminal command like ls /dev | grep usbmodem
. Select the correct port and board type in the Arduino IDE's "Tools" menu.
The "Blink" example is a good starting point for testing.
A video demonstrating the Blink example:
Streamlining with Firmata and PHP
Firmata simplifies Arduino interaction. Upload the "StandardFirmata" example to your Arduino.
Install the Carica Firmata library using Composer:
composer require carica/firmata
Connect to the Arduino in your PHP script:
use Carica\Io; use Carica\Firmata; $board = new Firmata\Board( Io\Stream\Serial\Factory::create( "/dev/cu.usbmodem14141", 57600 ) );
Remember to replace /dev/cu.usbmodem14141
with your Arduino's device name. Close the Arduino IDE before running the PHP script.
Add an event listener for connection:
$board ->activate() ->done( function() use ($board, $loop, $watcher) { $pin = $board->pins[9]; $pin->mode = Firmata\Pin::MODE_PWM; print "connected to Arduino"; } ); $loop->run();
Use setInterval
for periodic actions:
$loop->setInterval( function() use ($pin, $watcher) { // Code to check for changes and control the Arduino pin }, 1000 );
A video demonstrating the final integration:
For improved stability, especially on OSX, consider installing the Gorilla extension for Carica Firmata.
This concludes the integration of the Minecraft and Arduino circuits through PHP, showcasing the potential for creative applications.
(Note: The image URLs in the original input were relative and couldn't be directly used. I've preserved them as placeholders. You'll need to replace them with the actual image URLs.)
The above is the detailed content of PHP, Arduino, And... Minecraft? Connecting an Arduino to PHP!. For more information, please follow other related articles on the PHP Chinese website!
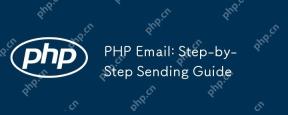
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
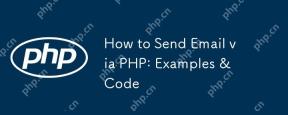
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
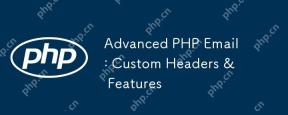
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
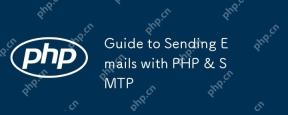
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
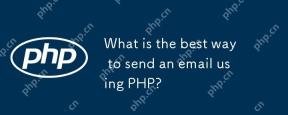
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
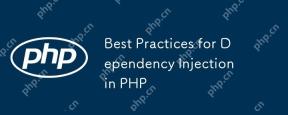
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
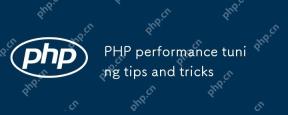
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
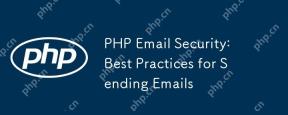
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
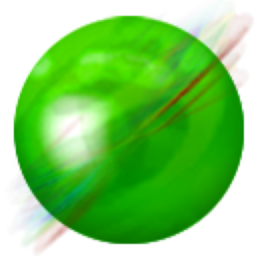
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
