


Modifying Structs via Value Receivers: Understanding the Shadowing Effect
In Go, the behavior of method receivers can have a profound impact on the mutability of structs. Understanding the difference between value receivers and pointer receivers is crucial for manipulating structs correctly.
Problem Context
The example provided demonstrates a seemingly unexpected behavior. A struct's field is modified within a method, but the modification does not persist outside of the method.
type Test struct { someStrings []string } func (this Test) AddString(s string) { this.someStrings = append(this.someStrings, s) this.Count() // will print "1" } func (this Test) Count() { fmt.Println(len(this.someStrings)) }
When this code is executed, it prints:
1 0
This behavior arises because the AddString method uses a value receiver, which essentially creates a copy of the struct when the method is invoked. The modifications made within the method are applied to this copy, not the original struct. Consequently, when Count is invoked outside the method, it operates on the original, unmodified struct.
Pointer Receivers vs. Value Receivers
To resolve this issue, a pointer receiver must be used instead of a value receiver. Pointer receivers create a reference to the original struct, allowing for direct manipulation.
func (t *Test) AddString(s string) { t.someStrings = append(t.someStrings, s) t.Count() // will print "1" }
With a pointer receiver, the AddString method operates directly on the receiver's struct. The modification to someStrings is thus reflected in the original struct. As a result, when Count is invoked outside the method, it operates on the modified struct.
Conclusion
By understanding the difference between value receivers and pointer receivers, you can effectively manipulate structs in Go. Value receivers create copies, while pointer receivers provide direct access to the original struct. This nuance is crucial for ensuring that changes made within methods are persistent outside of those methods.
The above is the detailed content of Why Doesn't Modifying a Go Struct's Field Within a Value Receiver Method Persist?. For more information, please follow other related articles on the PHP Chinese website!
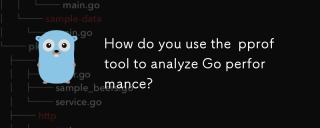
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
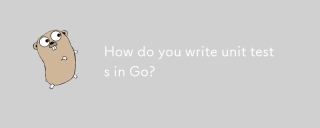
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
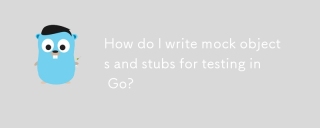
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
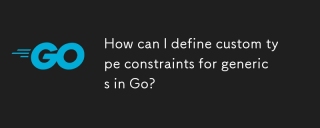
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
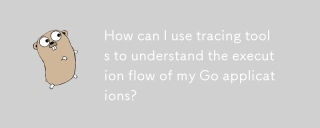
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
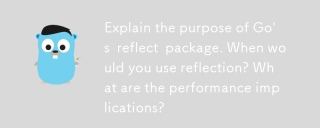
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
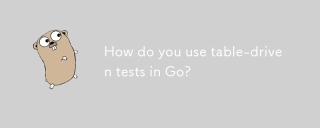
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
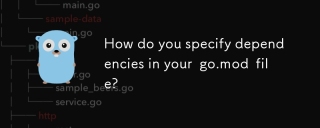
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
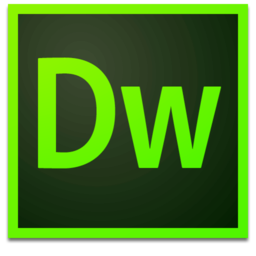
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
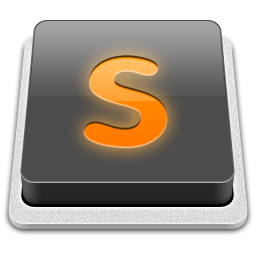
SublimeText3 Mac version
God-level code editing software (SublimeText3)
