


Sorting a Struct Array by Time.Time Member in Go
In Go, sorting a data structure by a specific member can be done using package sort. This is useful in various scenarios, such as when you want to order elements chronologically based on a date or time field.
To sort a slice of structs based on a time.Time member, you can define a custom type that implements the sort.Interface interface. This type must define the following methods:
- Len() int: Returns the length of the slice.
- Less(i, j int) bool: Determines if the element at index i is less than the element at index j.
- Swap(i, j int): Swaps the elements at indices i and j.
In the given code, you have defined a type timeSlice that implements these methods. However, the Less method compares the pointers to the time.Time values instead of the actual values. To fix this, you can use the Before method of time.Time to compare the time values directly:
func (p timeSlice) Less(i, j int) bool { return p[i].date.Before(p[j].date) }
Once you have defined the custom type, you can sort the slice using the sort.Sort function. However, for Go versions 1.8 and above, you can use the sort.Slice function, which is more efficient and concise:
sort.Slice(timeSlice, func(i, j int) bool { return timeSlice[i].date.Before(timeSlice[j].date) })
The sort.Slice function takes two arguments: the slice to be sorted and a closure that defines the comparison function. In this case, the closure returns true if the element at index i is less than the element at index j, and false otherwise.
After sorting the slice, the elements will be arranged in ascending order based on the date member.
The above is the detailed content of How to Efficiently Sort a Slice of Structs by a `time.Time` Member in Go?. For more information, please follow other related articles on the PHP Chinese website!
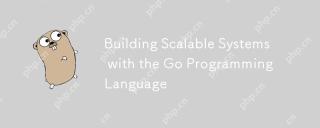
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
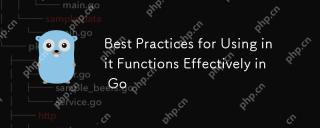
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
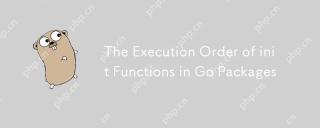
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations
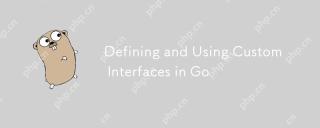
CustominterfacesinGoarecrucialforwritingflexible,maintainable,andtestablecode.Theyenabledeveloperstofocusonbehavioroverimplementation,enhancingmodularityandrobustness.Bydefiningmethodsignaturesthattypesmustimplement,interfacesallowforcodereusabilitya
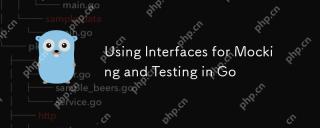
The reason for using interfaces for simulation and testing is that the interface allows the definition of contracts without specifying implementations, making the tests more isolated and easy to maintain. 1) Implicit implementation of the interface makes it simple to create mock objects, which can replace real implementations in testing. 2) Using interfaces can easily replace the real implementation of the service in unit tests, reducing test complexity and time. 3) The flexibility provided by the interface allows for changes in simulated behavior for different test cases. 4) Interfaces help design testable code from the beginning, improving the modularity and maintainability of the code.
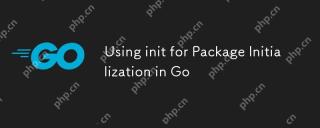
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
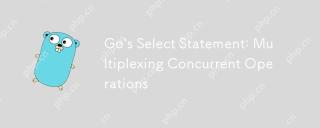
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
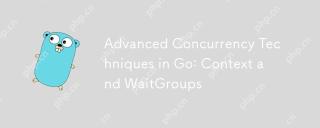
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
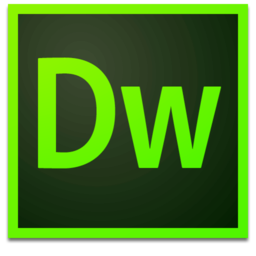
Dreamweaver Mac version
Visual web development tools
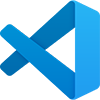
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
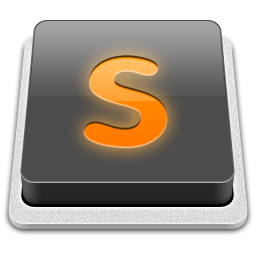
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
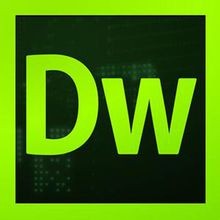
Dreamweaver CS6
Visual web development tools
