In this post, we will learn how to integrate JWT (JSON Web Tokens) middleware into Lithe, providing robust and secure authentication for your API. Using JWT allows you to authenticate users and secure sensitive routes simply and efficiently.
What is JWT?
JSON Web Token (JWT) is an open standard (RFC 7519) that defines a compact, self-contained method for transmitting information between parties as a JSON object. These tokens can be used for authentication, allowing you to maintain a user's session without needing to store information on the server. JWT is composed of three parts: header, payload and signature.
Step 1: Configuring the Environment
- Lithe Installation First, install Lithe if you haven't already. Run the following command in the terminal:
composer create-project lithephp/lithephp nome-do-projeto cd nome-do-projeto
Step 2: Installing the JWT Middleware
- Installing the JWT Package To use the JWT middleware, you need to install the lithemod/jwt package. Run:
composer require lithemod/jwt
- Starting the application Open the main src/App.php file and add the following code to start the application:
use function Lithe\Orbis\Http\Router\router; $app = new \Lithe\App; $app->use('/api', router(__DIR__ . '/routes/api')); $app->listen();
Step 3: Securing Routes with JWT
- Creating a Protected Route In your Lithe project, you can create a route that requires authentication. For example, create a file called src/routes/api.php and add:
use Lithe\Http\{Request, Response}; use function Lithe\Orbis\Http\Router\{get}; $auth = new \Lithe\Auth\JWT(); get('/protected', $auth, function(Request $req, Response $res) { return $res->json(['message' => 'Este é um conteúdo protegido!']); });
Step 4: Generating JWT Tokens
- Creating a Login Route Create a route for authentication where users can obtain a JWT token. Add the following in the same src/routes/api.php file:
use Lithe\Http\{Request, Response}; use function Lithe\Orbis\Http\Router\{post}; post('/login', function(Request $req, Response $res) { $body = $req->body(); // Supondo que o corpo da requisição contenha 'username' e 'password' // Aqui você deve validar as credenciais do usuário (exemplo simplificado) if ($body->username === 'admin' && $body->password === 'senha') { $user = ['id' => 1]; // Exemplo de usuário $token = (new \Lithe\Auth\JWT())->generateToken($user); return $res->send(['token' => $token]); } return $res->status(401)->json(['message' => 'Credenciais inválidas']); });
Of course! Here is the updated section with information about setting a secure and secret key when using JWT:
Final Considerations
With this, you have successfully integrated JWT middleware with Lithe, enabling secure authentication and protection of sensitive routes. It is important to remember that when using JWT, you must define a secure and secret key when instantiating the JWT object, passing it as the first parameter: new JWT('your_secret_key'). This key must be complex and kept secret to avoid fraud.
Now you can expand your application as needed and implement additional functionality such as token revocation and session management.
To learn more about JWT, you can check out the official documentation here.
Feel free to share your experiences and questions in the comments!
The above is the detailed content of Integrating JWT Authentication into Lithe. For more information, please follow other related articles on the PHP Chinese website!
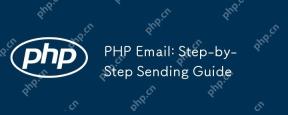
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
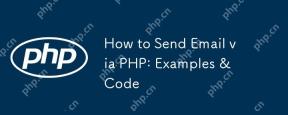
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
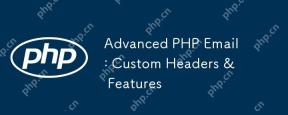
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
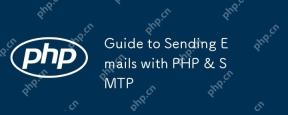
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
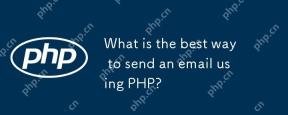
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
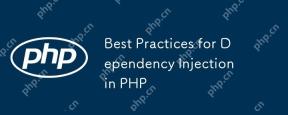
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
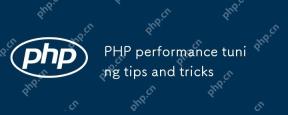
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
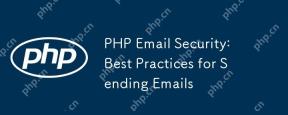
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
