


Preserve Key Order When Sorting Associative Arrays in PHP
Associative arrays in PHP store key-value pairs. When sorting such arrays using PHP's built-in sorting functions, it is important to consider whether or not to preserve the original key order. In this article, we will explore the current behavior of sorting in PHP and provide a custom solution for preserving key order.
PHP's Sort Functions and Stability
In PHP versions prior to 4.1.0, the sorting functions, such as asort() and uasort(), provided a stable sort. This means that when multiple keys had the same value, the order of those keys would be preserved in the sorted result. However, starting with PHP 4.1.0, stability is no longer guaranteed.
Custom Stable Sort Function
Since PHP's built-in sorting functions do not support stable sorting, we need to implement our own. The merge sort algorithm is commonly used for this purpose due to its guaranteed O(n*log(n)) time complexity:
<code class="php">function mergesort(&$array, $cmp_function = 'strcmp') { ... // Logic for splitting, merging, and sorting the array ... }</code>
This custom function can be used with uasort() to sort the associative array while preserving the original key order:
<code class="php">uasort($arr, function($a, $b) { return mergesort([$a, $b]); });</code>
Example Usage
<code class="php">$arr = [ 'key-1' => 10, 'key-2' => 20, 'key-3' => 10, 'key-4' => 30, ]; uasort($arr, function($a, $b) { return mergesort([$a, $b]); }); print_r($arr);</code>
Output:
Array ( [key-1] => 10 [key-3] => 10 [key-2] => 20 [key-4] => 30 )
By using the custom stable sort function, the key order of the original array is preserved in the sorted output.
The above is the detailed content of How to Preserve Key Order When Sorting Associative Arrays in PHP?. For more information, please follow other related articles on the PHP Chinese website!
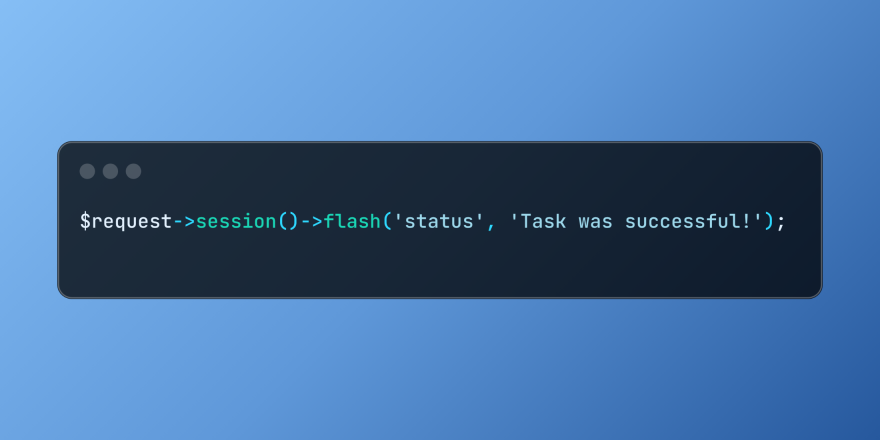
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
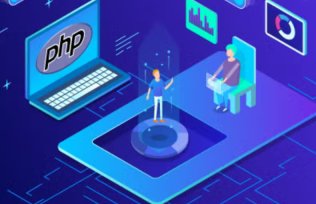
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
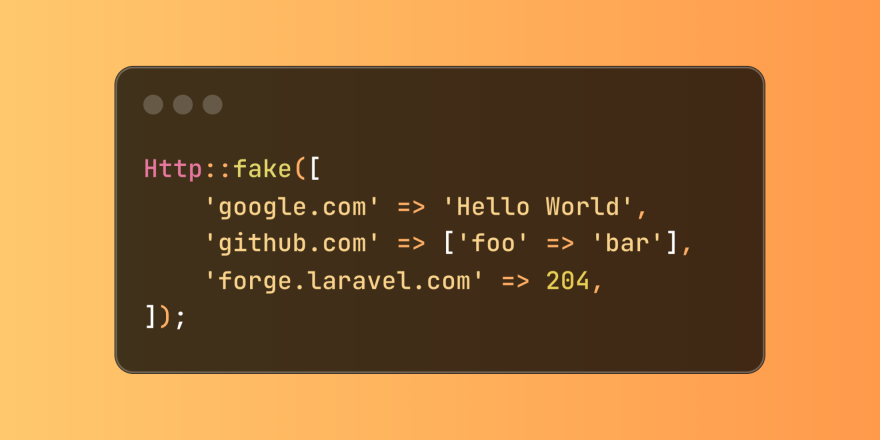
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
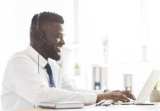
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
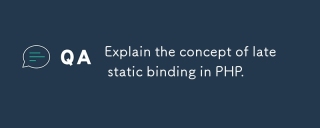
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
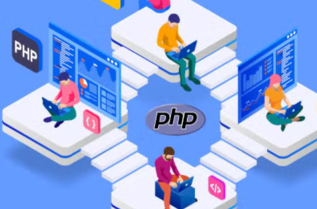
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
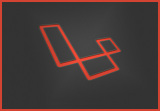
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove
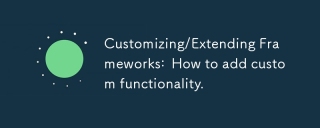
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
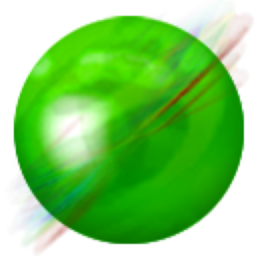
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
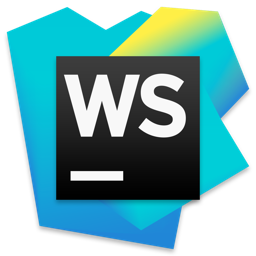
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
