A queue is a collection of object it represents in the form of FIFO (First-In-First-Out) order the element which is added first will come out first, in C# Queue collection class present in the namespace System.Collection. Queue stores the element in FIFO order in which we can retrieve in a first-in, first-out manner of accessing elements. A queue is exactly just opposite to Stack Collection, where Stack is LIFO (Last-In-First-Out). The collection of Queue allows numerous null and duplicate values. Queue uses two methods called Enqueue() and Dequeue() which used for adding and retrieving values respectively.
Syntax:
The queue is created using the data type called Queue. Here the “new” keyword is used for creating an object of the queue. In queue collection for adding an item, we using the Enqueue method and for deleting an item we using the Dequeue method.
Queue QueueObject = new Queue() // creation of Queue
QueueObject.Enqueue(element) // to add element to Queue
QueueObject.Dequeue() //to remove element to Queue
How Queue works in C#?
Queue present in the form of FIFO (First-In-First-Out) it’s a collection of objects, this process is used when we need to access in first-in, first-out access of items. The queue is a non-generic it uses the type of collection which is defined in the System.Collections namespace. In general, a queue is useful when we use the information in the way which we stored in the queue collection.
The Queue implements through the interfaces called IEnumerable, ICloneable, ICollection. For the reference types, it accepts the null valid values. In queue collection for adding an item, we using the Enqueue method and for deleting an item we using the Dequeue method when adding an item to queue the total capacity is automatically increase for required internal memory.
Example:
using System; using System.Collections; public class QueueProgram { static public void Main() { // to create a queue - using Queue class Queue _objQueue = new Queue(); // to add an elements in Queue - using Enqueue() method _objQueue.Enqueue("DotNet"); _objQueue.Enqueue("SQL"); _objQueue.Enqueue("Java"); _objQueue.Enqueue("PHP"); _objQueue.Enqueue("Android"); Console.WriteLine("Working Process of Queue\n"); Console.WriteLine("Number of Elements Present in Object(_objQueue) : {0}", _objQueue.Count); // to obtain the topmost element of _objQueue - using Dequeue method Console.WriteLine("\nTo Get the topmost element in Queue" + " is : {0}", _objQueue.Dequeue()); Console.WriteLine("\nNumber of Elements Present in Object(_objQueue) : {0}", _objQueue.Count); // to obtain the topmost element of _objQueue - using Peek method Console.WriteLine("\nTo Get the topmost element in Queue is : {0}", _objQueue.Peek()); Console.WriteLine("\nNumber of Elements Present in Object(_objQueue) : {0}", _objQueue.Count); // to check hether the element is present in the Queue if (_objQueue.Contains("SQL") == true) { Console.WriteLine("\nElement is Present !"); } else { Console.WriteLine("\nElement is not Present !"); } } }
In the above program, we declare the Queue as _ objQueue to hold the items of Queue. For adding the new element we using the method Enqueue() and for deleting the element we using the Dequeue() method. The property Count is used to get the total number of elements in the queue, the return value of this property is a number. Another method Contains() is used to check whether the given value/element is present, it returns the bool value either true or false. The Peek() is used to obtain the topmost value in the queue collection.
Output:
From the above output, it shows that the items of the Queue are displayed. Firstly it displays the total number of elements present in the queue by using the Count() method and then it displays the topmost element by using the Peek() method. By using the Contains() method it checks whether the element present in the queue collection.
Constructors
In Queue class it consists of constructors that are used to create a queue.
- Queue(): The constructor Queue() is used for creating the instance of queue class, it helps in the use of default growth factor.
- Queue(ICollection): This constructor is used for creating an instance of the queue and it contains the items copied from the specified collection and having the same capacity as the number of items copied. It also uses the default initial growth factor.
- Queue(Int32): This constructor is used to create a Queue class instance that is empty and has initial capacity specified, and uses the default growth factor.
- Queue(Int32, Single): This constructor is used to create a Queue class instance that is empty and has initial capacity specified, and uses the default growth factor.
Queue functions in C# method
Let’s see the following functions list which is commonly used methods of the Queue class −
- Enqueue(): Enqueue method is used when adding an element in Queue, it is a non-generic collection so we can add an element of any datatype in this method. The signature used for this method is void Enqueue(object obj)
- Dequeue(): Dequeue method is for access queue which is used to retrieve the topmost element in the queue. By the FIFO approach Dequeue used to remove and its resultant one which returns the first element in the queue collection, the Dequeue() is called only when the total count of the queue is always greater than zero otherwise it throws an exception. The signature used for this method is object Dequeue()
- Peek(): This method will return always the first element from the queue collection without removing from the queue. It throws an exception if the empty queue collection is called.
- The signature used for this method is object Peek().
- Clear(): This method is used to remove objects from the queue collection. The signature used for this method is void Clear().
- Contains(): This method is used to check whether an element exists in the collection of Queue. The signature used for this method is bool Contains(object obj).
- Clone(): Clone() method is used for creating a shallow copy of queue collection.
- Equals(Object): This method is used to check whether the particular object is equal to the current object.
- Synchronized(Queue): This method returns a new queue which encloses the original queue.
- TrimToSize(): This method is used to set the capacity to which the actual number of items in the queue collection.
Conclusion
In this article, we came to know the Queue() usage in C#, it is based on the FIFO concept, for adding and deleting the queue we using the Enqueue() and Dequeue() methods respectively.
The above is the detailed content of Queue in C#. For more information, please follow other related articles on the PHP Chinese website!
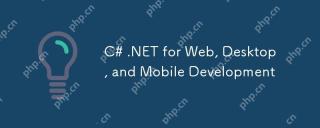
C# and .NET are suitable for web, desktop and mobile development. 1) In web development, ASP.NETCore supports cross-platform development. 2) Desktop development uses WPF and WinForms, which are suitable for different needs. 3) Mobile development realizes cross-platform applications through Xamarin.
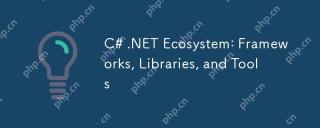
The C#.NET ecosystem provides rich frameworks and libraries to help developers build applications efficiently. 1.ASP.NETCore is used to build high-performance web applications, 2.EntityFrameworkCore is used for database operations. By understanding the use and best practices of these tools, developers can improve the quality and performance of their applications.

How to deploy a C# .NET app to Azure or AWS? The answer is to use AzureAppService and AWSElasticBeanstalk. 1. On Azure, automate deployment using AzureAppService and AzurePipelines. 2. On AWS, use Amazon ElasticBeanstalk and AWSLambda to implement deployment and serverless compute.
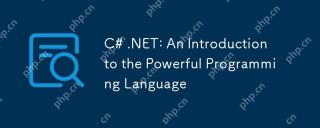
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
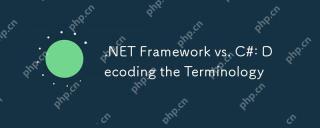
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
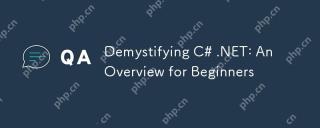
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
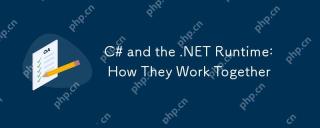
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
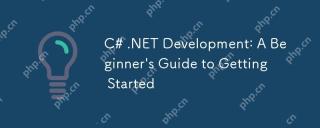
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
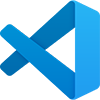
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
