PHP inbuilt supports a few data types. Apart from these, PHP also supports many functions that are used while working on some data. PHP String functions are some of those functions that are used to manipulate string data. All these functions are predefined. There is a need for installing any plugins. Let’s look at some of the PHP string functions.
ADVERTISEMENT Popular Course in this category PHP DEVELOPER - Specialization | 8 Course Series | 3 Mock TestsStart Your Free Software Development Course
Web development, programming languages, Software testing & others
Below are some of the string functions, and examples are illustrated with the following syntax.
<?php echo func( "<data>" ); ?>
Examples of String Functions in PHP
The string function is easy to use. Here we will discuss how to use string function in PHP programming with the help of examples.
1. Addcslashes()
This returns a string with backslashes in front of specific characters
E.g.:
echo addcslashes ("Hello World!","W");
Output:
Hellow World
2. Addslashes()
This returns string with backslashes in front of predefined characters
E.g.:
echo addcslashes('Hello "World" you');
Output:
Hello ” World” you.
3. bin2hex()
Converts binary data into hexadecimal data
E.g.:
echo bin2hex ("Hello");
Output:
48656c6c6f
4. chop()
Removes whitespaces or any predefined characters from the right end if specified
E.g.:
echo chop ("WelcomeBack" , "Back");
Output:
Welcome
5. chr()
This PHP string function returns the character of a specified ASCII value.
E.g.:
echo char(52);
Output:
4
6. chunk_split()
Used to split a string into smaller parts
E.g.:
echo chunk_split ($str, 2,", ");
Output:
We,lc,om,e,
7. convert_uudecode()
This decodes a uuencoded string
E.g.:
echo convert_uudecode ("+22!L;W9E( %!( 4\"$`\n` ");
Output:
I love PHP!
convert_uuencode() does the reverse of convert_uudecode()
8. count_chars()
This PHP string function outputs the data about the number of characters in a string.
E.g.:
echo count_chars ("Hello", 3);
Output:
Helo
Note: The integer value is the mode which is used to specify the type of output required- 0 – an array with the byte-value as key and the frequency of every byte as value.
- 1 – same as 0, but only byte-values with a frequency greater than zero are listed.
- 2 – same as 0, but only byte-values with a frequency equal to zero are listed.
- 3 – a string containing all unique characters is returned.
- 4 – a string containing all not used characters is returned.
9. crc32()
This calculates the 32-bit cyclic redundancy checksum( A Mathematical function) of a string.
E.g.:
crc32 ("Hello World!");
Output:
472456355
10. Implode()
This joins the array elements with a specified string
E.g.:
$array = array ('lastname', 'email', 'phone'); echo implode(",", $array);
Output:
lastname, email, phone
Note: join() also does the same. It is the alias of implode().11. htmlspecialchars()
This converts some predefined characters to HTML entities, i.e. it shows the source.
Eg:
$str = "I am <b>Bold</b>"; echo $str; => I am <strong>Bold</strong> echo htmlspecialchars($str);
Output:
I am Bold
12. ltrim()
This PHP string function removes white spaces or predefined characters from the left of the string.
E.g.:
echo ltrim ("Just a sample", "Just");
Output:
a sample
Note: rtrim() does a similar job from righttrim() does the same from both ends.
13. number_format()
This formats the number with grouped thousands
E.g.:
echo number_format (1000000);
Output:
1,000,000
14. print()
This simply outputs the string and is slower than the echo
Also, the print should not be used with ()
E.g.:
print "Hello";
Output:
Hello
15. md5()
This calculates the md5 hash of the string
E.g.:
echo md5 ("Hello");
Output:
8b1a9953c4611296a827abf8c47804d7
16. strtok()
This splits a string into smaller strings
E.g.:
$string = "This is to break a string"; $token = strtok ($string, " "); echo($token); => This To get all words of string, while ($token !== false){ echo "$token<br>"; $token = strtok(" "); }
Output:
This
is
to
break
string
17. strupper()
This converts a string to uppercase characters
E.g.:
echo strupper ("Beautiful Day");
Output:
BEAUTIFUL DAY
Note: strlower() converts strings to all lowercase characters.18. substr()
This returns part of the string starting with the index specified
E.g.:
echo subst ("A Hot Day", 3);
Output:
ot Day
19. substr_replace()
This PHP string function replaces a part of the string with the string specified.
E.g.:
echo substr_replace ("Hot", "Day",0);
Output:
Day
20. wordwrap()
This wraps a string to a number of characters
E.g.:
echo wordwrap ("Hello World", 5, "\n");
Output:
Hello
World
21. Strlen()
This is used to determine the length of the string passed
E.g.:
echo strlen ("Hello");
Output:
5
22. Strrev()
This PHP string function is used to get the reverse of the string
E.g.:
echo strrev ("welcome");
Output:
emoclew
23. Strpos()
This returns the position of the first occurrence of a string inside a string
E.g.:
echo strops("There you go", "go");
Output:
11
24. Str_repeat()
This repeats a string specified number of times
E.g.:
echo str_repeat ('b', 5);
Output:
bbbbb
25. Str_replace()
This PHP string function finds the specified word, replaces that with a specified word, and return the string
E.g.:
echo str_replace ("great", "wonderful", "have a great day");
Output:
have a wonderful day
26. Nl2br()
This PHP string function inserts html line breaks in front of each new line of the string
E.g.:
echo nl2br ("Lets break \nthe sentence");
Output:
Lets break
the sentence
27. similar_text()
This calculates the similarity between two strings
E.g.:
echo similar_text ("Hello World","Great World");
Output:
7
28. sprintf()
This PHP string function writes a formatted string to a variable
E.g.:
echo sprintf ("There are %u wonders in the World",7);
Output:
There are 7 wonders in the World
29. Str_ireplace()
This replaces characters in the string with specific characters. This function is case insensitive.
E.g.:
echo str_ireplace ("great", "WOW", "This is a great place");
Output:
This is a wow place
30. str_shuffle()
This randomly shuffles all characters in a string
E.g.:
echo str_shuffle("Hello World");
Output:
lloeWlHdro
31. str_word_count()
This PHP string function returns the number of words in the given string
E.g.:
echo str_word_count ("a nice day");
Output:
3
32. Strcspn()
This returns the number of characters before the specified character
echo strcspn ("Hello world!","w");
Output:
6
33. str_pad()
This function is used to pad to the right side of the string, a specified number of characters with the specified character
E.g.:
echo str_pad ("Hello", 10, ".");
Output:
Hello…..
34. Ord()
This PHP string function returns the ASCII value of the first character of the string.
E.g.:
echo ord ("hello");
Output:
104
35. Strchr()
Find the first occurrence of a specified string within a string
E.g.:
echo strchr ("Hello world!", "world");
Output:
world!
36. Strspn()
This returns the number of characters found in the string that contains characters from the specified string.
E.g.:
echo strspn ("Hello world!", "Hl");
Output:
1
There are few more string functions available in PHP. The above string functions are commonly used functions in PHP for various requirements.
The above is the detailed content of PHP String Functions. For more information, please follow other related articles on the PHP Chinese website!
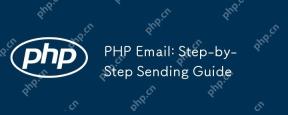
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
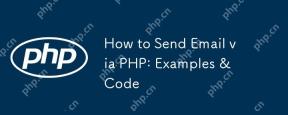
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
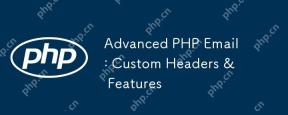
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
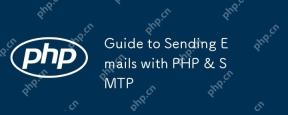
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
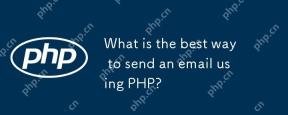
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
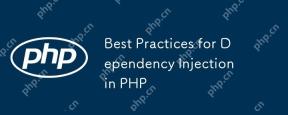
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
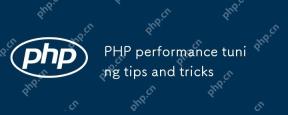
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.
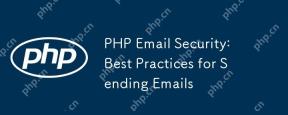
ThebestpracticesforsendingemailssecurelyinPHPinclude:1)UsingsecureconfigurationswithSMTPandSTARTTLSencryption,2)Validatingandsanitizinginputstopreventinjectionattacks,3)EncryptingsensitivedatawithinemailsusingOpenSSL,4)Properlyhandlingemailheaderstoa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
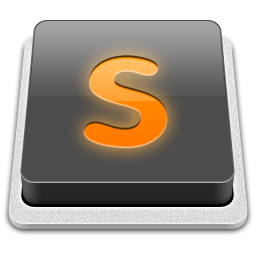
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version
