As we all know that PHP is one of the most widely used languages for web development. Understanding the basic concepts is very important in any programming language before diving deep into the advanced ones. Loops are one of the largely and most commonly used while writing any piece of code as their main purpose is to execute the same piece of code repeatedly according to specific requirements of a programmer. Code/statements inside the while loop in PHP execute until the programmer’s condition remains ‘true’. There is no need to specify the exact number of iterations for which a while loop should run, unlike ‘for’ loops.
ADVERTISEMENT Popular Course in this category PHP DEVELOPER - Specialization | 8 Course Series | 3 Mock TestsStart Your Free Software Development Course
Web development, programming languages, Software testing & others
Below mentioned is the syntax of while loop in PHP:
Syntax:
while (condition to be true) { .. .. // Set of Statements to be executed .. .. .. }
Statements inside the while loop will not execute once the loop’s condition is evaluated to be false.
Flowchart
Below given is the basic flowchart expressing the process of how the while loop performs its action.
How While Loop works in PHP?
As explained above, while loop works until the condition specified is satisfied. Working of while loop in PHP is explained in the below steps:
- First, the condition given inside the brackets after the while keyword is checked.
- If the condition is satisfied or is true, then the control is moved inside the loop.
- The statements inside the loop are executed.
- Once all the statements inside the loop are executed, the condition is checked again, and if it is true, the execution continues.
- When the condition is evaluated to be false, the control will not move inside the loop, and the while loop terminates.
Examples of While Loop in PHP
Below are the different examples of a while loop in PHP:
Example #1
Printing the value of a field according to the specific condition.
Code:
<title>PHP while Loop Example 1</title> <?PHP $value = 10; while ((int)$value > 5) { echo "The value of the field is : $value <br>"; $value--; } ?>
Output:
Explanation
In the above program, a variable with the name ‘value’ is assigned with the value 10. Now the while loop condition is checked, i.e. 10 > 5, which is true, so the statements inside the loop will execute. The value of variable ‘value’ is decreased by 1 and again checked with the while condition. Execution of statements inside the while loop continues until the value of the variable becomes 6. Once the value becomes 5 and the condition evaluates to be false (5 > 5), the while loop terminates, and the echo statement inside the while loop will not execute.
Example #2
Printing the sum of digits of a given number.
Code:
<title>PHP while Loop Example 2</title> <?PHP $number = 107; $sum=0; $rem=0; while((int)$number != 0) { $rem=$number%10; $sum = $sum + $rem; $number=$number/10; } echo "The Sum of digits of number given 107 is $sum"; ?>
Output:
Explanation
In the above example, the sum of the digits of the number ‘107’ is calculated, which is 1+0+7. First the condition of while loop, i.e. 107 != 0, is checked. As the condition evaluates to be true, control will move inside the loop remainder (rem) is calculated (107%10), i.e. 7 and is added to the sum variable, which becomes 0+7 =7. Number now becomes 107/10 = 10. Again the number 10 is checked against the while condition, which is set to be true, and the control will again move inside the loop. Rem variable now is 10%10 =0 and sum becomes 7+ 0 = 7 . number variable now becomes 10/10 =1, which is again not equal to 0 and move inside the while loop, so the rem variable becomes 1%10 =1. sum =7+1 =8. Number variable becomes 1/10 =0. Now the while condition is evaluated to be false, so the cursor will not move inside the while loop, and the sum final value becomes 8, which is printed on the screen.
Example #3
Generate and print the table of number 6.
Code:
<title>PHP while Loop Example 2</title> <?PHP $table_number= 6; $mult =1; while((int)$mult<=10) { echo "$table_number * $mult"; echo "<br>"; $mult++; } ?>
Output:
Explanation
In the above program, the table of the variable, ‘table_number’, is printed. In general, a number whose table needs to be printed remains the same, i.e. 6 in this case, whereas the multiples keep on incrementing from 1 till 10. For the first time, when the value of the ‘mult’ variable is 1, so the condition of while loop, i.e. 1
Conclusion
The above explanation clearly describes the syntax of a while loop along with its working in a program. Though there are 4 types of loops used in PHP, and every loop is used in a particular situation. The programmer mainly uses a loop when the iterations are not fixed, and we need to execute the set of statements until the main condition is evaluated. It is important to understand the working of loops before using them, as partial knowledge of them can sometimes lead to unexpected results.
The above is the detailed content of While Loop in PHP. For more information, please follow other related articles on the PHP Chinese website!
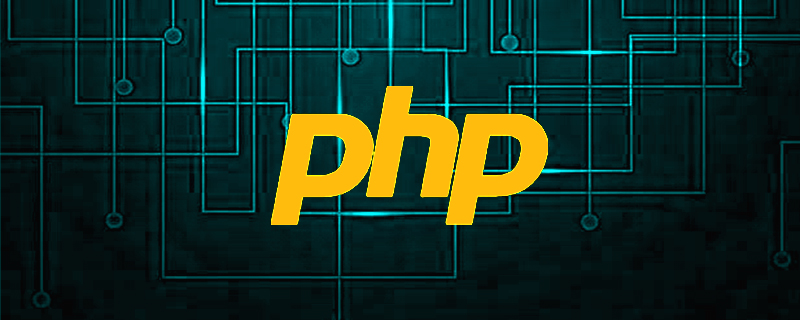
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
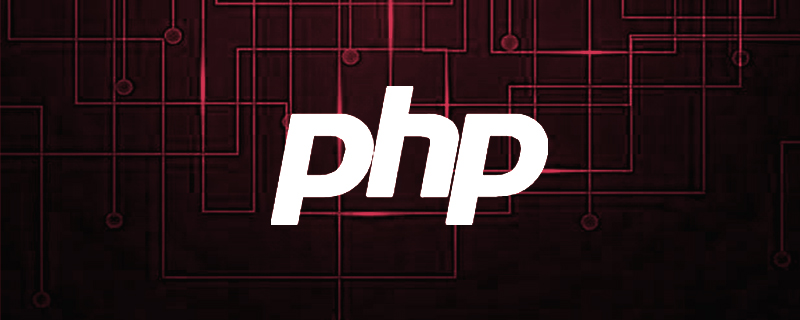
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
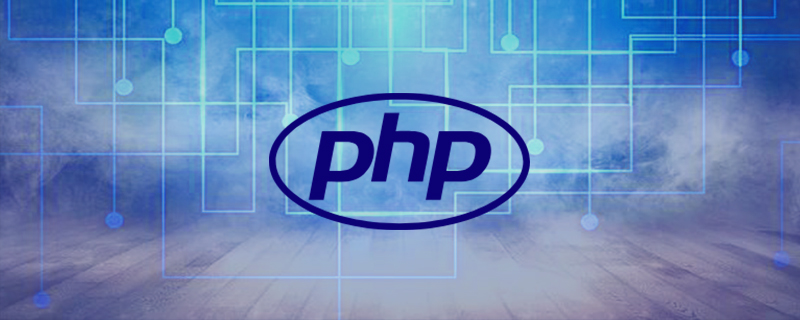
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
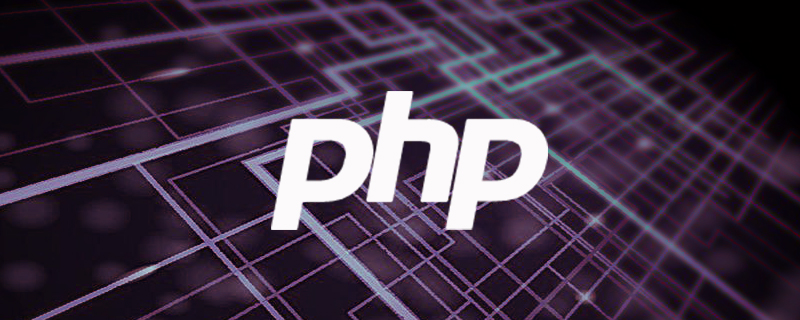
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
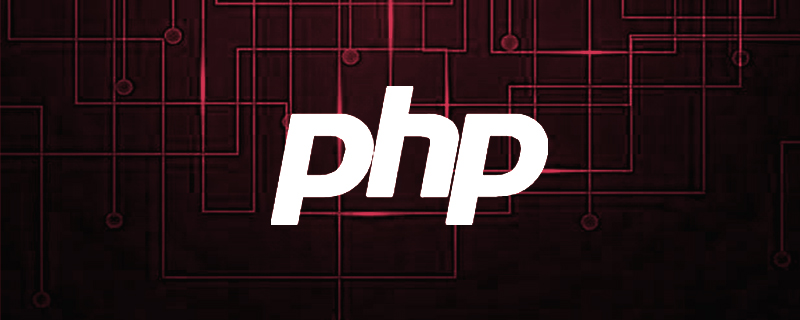
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
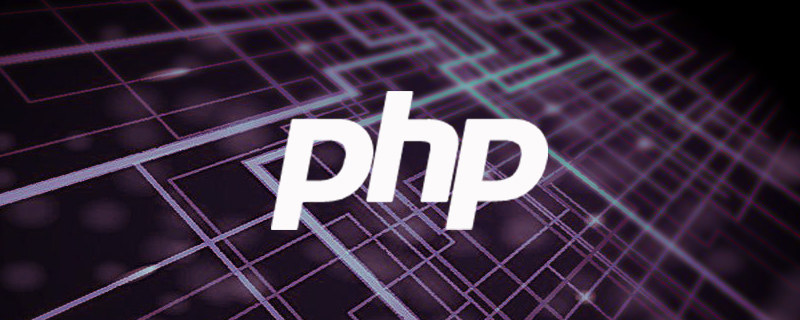
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
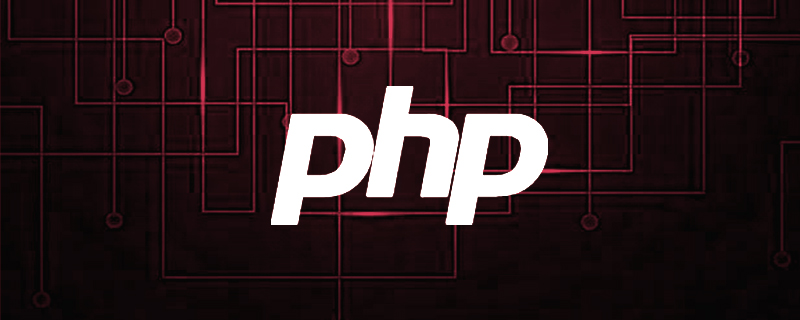
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
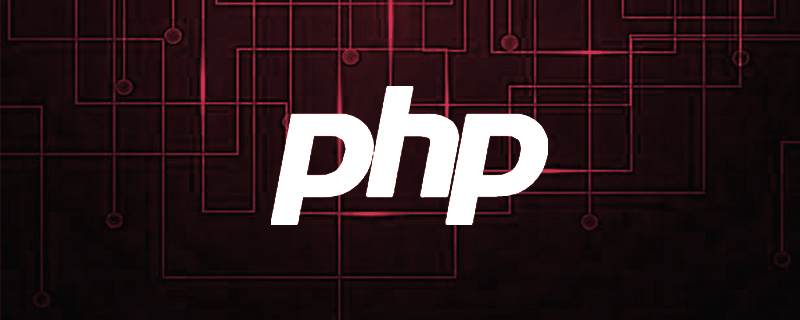
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
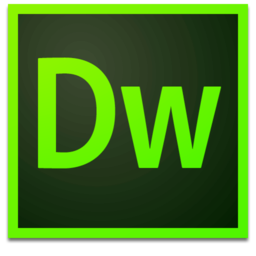
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
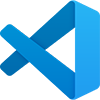
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
