


In-depth understanding of PHP object-oriented programming: extension and customization of object-oriented programming
PHP object-oriented programming can be extended through extensions and custom classes. An extended class inherits the properties and methods of the parent class and can add new properties and methods; a custom class implements specific functions by implementing interface methods. In the actual case, by extending the abstract class Shape, concrete shapes such as Circle and Rectangle are created, and the area can be calculated dynamically.
PHP Object-Oriented Programming: Extensions and Customization
Object-oriented programming (OOP) allows you to create reusable, maintainable code. In PHP, OOP can be further extended by extending and customizing existing classes.
Extended classes
Use the extends
keyword to extend a class. The extended class inherits all properties and methods of the parent class and can add new properties and methods.
class BaseClass { protected $name; public function __construct($name) { $this->name = $name; } public function getName() { return $this->name; } } class ExtendedClass extends BaseClass { private $age; public function __construct($name, $age) { parent::__construct($name); $this->age = $age; } public function getAge() { return $this->age; } }
Customized class
Use the implements
keyword to customize a class so that it implements one or more interfaces. An interface defines a set of methods that the class must implement.
interface MyInterface { public function doSomething(); } class MyClass implements MyInterface { public function doSomething() { // 具体实现 } }
Practical case
Consider an abstract class Shape
, which defines a getArea()
method. We extend this class to create concrete shapes such as Circle
and Rectangle
.
abstract class Shape { protected $color; public function __construct($color) { $this->color = $color; } abstract public function getArea(); } class Circle extends Shape { private $radius; public function __construct($color, $radius) { parent::__construct($color); $this->radius = $radius; } public function getArea() { return pi() * $this->radius ** 2; } } class Rectangle extends Shape { private $width; private $height; public function __construct($color, $width, $height) { parent::__construct($color); $this->width = $width; $this->height = $height; } public function getArea() { return $this->width * $this->height; } }
We can dynamically calculate the area by creating Circle
and Rectangle
objects and accessing their respective getArea()
methods.
The above is the detailed content of In-depth understanding of PHP object-oriented programming: extension and customization of object-oriented programming. For more information, please follow other related articles on the PHP Chinese website!
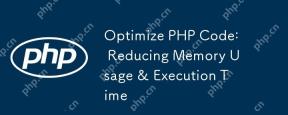
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
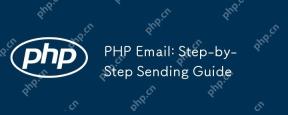
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
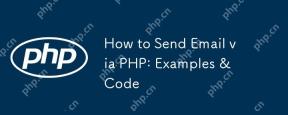
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
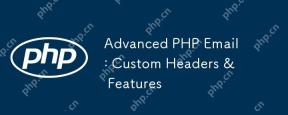
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
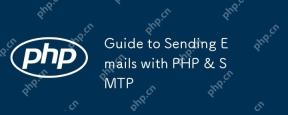
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
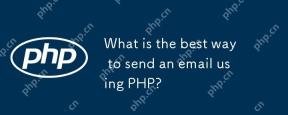
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
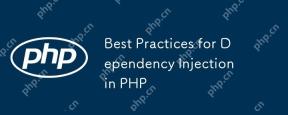
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
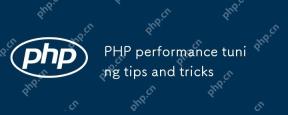
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
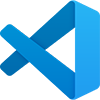
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
