How to use buffers to optimize file reading and writing in Golang?
File read and write performance in Golang can be optimized by using buffers: buffers store data read or written from disk, thereby reducing the number of disk operations. Examples of read and write functions that use buffers: readFileBuffered and writeFileBuffered. Practical example: Using buffers can reduce the number of disk operations for a 1GB file from 1,000,000 to 1,024. Using buffering technology improves application efficiency when processing large files.
#How to use buffers to optimize file reading and writing in Golang?
Background
In Golang, directly use ioutil.ReadFile
or ioutil.WriteFile
to read files While writing, you may encounter performance issues. This is because these functions read or write the entire file from disk with each operation, which is inefficient when working with large files.
Advantages of buffers
Buffers can effectively alleviate this problem. A buffer is an area in memory used to store data read from or written to disk. By using buffers, we can read or write data in chunks, reducing the number of disk operations and thus improving performance.
Code example: Use buffering to optimize file reading and writing
import ( "bytes" "io" "os" ) // 读文件 func readFileBuffered(filename string) ([]byte, error) { f, err := os.Open(filename) if err != nil { return nil, err } defer f.Close() // 创建一个缓冲器,缓冲区大小为 1024 字节 buf := bytes.NewBuffer(nil) _, err = io.CopyBuffer(buf, f, 1024) if err != nil { return nil, err } return buf.Bytes(), nil } // 写文件 func writeFileBuffered(filename string, data []byte) error { f, err := os.Create(filename) if err != nil { return err } defer f.Close() // 使用缓冲器写入文件 buf := bytes.NewBuffer(data) _, err = io.CopyBuffer(f, buf, 1024) if err != nil { return err } return nil }
Actual case
Suppose we have a 1GB file, Need to read from disk and write to another file. Using the readFileBuffered
and writeFileBuffered
functions we can reduce the disk operations to approximately 1024 times, compared to what is required when using ReadFile
and WriteFile
directly Perform 1,000,000 operations.
Conclusion
By using buffers, we can significantly optimize file reading and writing performance in Golang. It is recommended to use buffering technology when processing large files to reduce the overhead of disk operations and improve application efficiency.
The above is the detailed content of How to use buffers to optimize file reading and writing in Golang?. For more information, please follow other related articles on the PHP Chinese website!
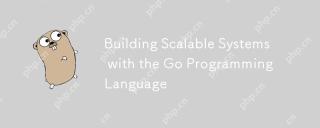
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
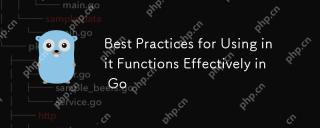
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
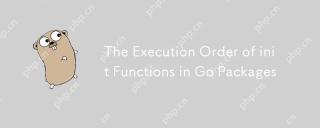
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations
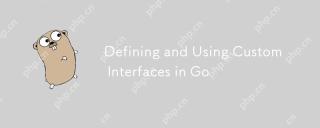
CustominterfacesinGoarecrucialforwritingflexible,maintainable,andtestablecode.Theyenabledeveloperstofocusonbehavioroverimplementation,enhancingmodularityandrobustness.Bydefiningmethodsignaturesthattypesmustimplement,interfacesallowforcodereusabilitya
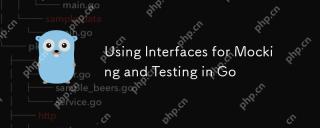
The reason for using interfaces for simulation and testing is that the interface allows the definition of contracts without specifying implementations, making the tests more isolated and easy to maintain. 1) Implicit implementation of the interface makes it simple to create mock objects, which can replace real implementations in testing. 2) Using interfaces can easily replace the real implementation of the service in unit tests, reducing test complexity and time. 3) The flexibility provided by the interface allows for changes in simulated behavior for different test cases. 4) Interfaces help design testable code from the beginning, improving the modularity and maintainability of the code.
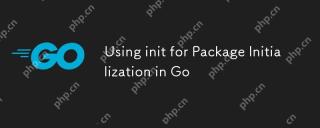
In Go, the init function is used for package initialization. 1) The init function is automatically called when package initialization, and is suitable for initializing global variables, setting connections and loading configuration files. 2) There can be multiple init functions that can be executed in file order. 3) When using it, the execution order, test difficulty and performance impact should be considered. 4) It is recommended to reduce side effects, use dependency injection and delay initialization to optimize the use of init functions.
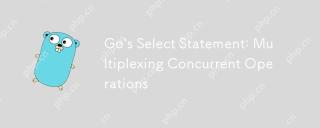
Go'sselectstatementstreamlinesconcurrentprogrammingbymultiplexingoperations.1)Itallowswaitingonmultiplechanneloperations,executingthefirstreadyone.2)Thedefaultcasepreventsdeadlocksbyallowingtheprogramtoproceedifnooperationisready.3)Itcanbeusedforsend
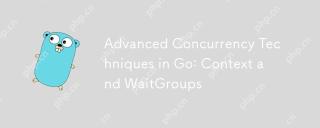
ContextandWaitGroupsarecrucialinGoformanaginggoroutineseffectively.1)ContextallowssignalingcancellationanddeadlinesacrossAPIboundaries,ensuringgoroutinescanbestoppedgracefully.2)WaitGroupssynchronizegoroutines,ensuringallcompletebeforeproceeding,prev


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
