Advanced tips for PHP project development: Use namespaces to organize code and avoid name conflicts. Use dependency injection to enhance code flexibility and improve testability. Ensure that the code runs accurately through unit testing. Use Composer to manage dependencies and simplify installation and updates. Deployment strategies ensure high availability and fault tolerance, such as blue-green deployment and automatic scaling.
Advanced skills in PHP project development
1. Use namespaces
Namespaces are used to organize and isolate classes and functions and constants to prevent name conflicts. By using namespaces, you can create unique and meaningful namespaces for your project while avoiding conflicts with external libraries or modules.
Practical case:
<?php // 创建一个名为 "MyProject" 的命名空间 namespace MyProject; // 定义 "User" 类 class User { ... 代码 ... }
2. Dependency injection
Dependency injection is a design pattern that allows you to pass dependencies at runtime to object. This allows you to create loosely coupled modules and components, increasing flexibility in testing and maintenance.
Practical case:
<?php class UserRepository { private $database; public function __construct(Database $database) { $this->database = $database; } ... 代码 ... } // 在控制器中使用 UserRepository class UserController { private $userRepository; public function __construct(UserRepository $userRepository) { $this->userRepository = $userRepository; } ... 代码 ... }
3. Unit testing
Writing unit tests ensures that your code runs as expected. PHPUnit is a popular unit testing framework that provides a powerful set of assertions and tools to help you verify your code.
Practical case:
<?php class UserTest extends PHPUnit\Framework\TestCase { public function testCreate() { $user = new User([ 'name' => 'John Doe', 'email' => 'john.doe@example.com', ]); $this->assertEquals('John Doe', $user->getName()); $this->assertEquals('john.doe@example.com', $user->getEmail()); } }
4. Use Composer for dependency management
Composer is a dependency manager used to manage third-party libraries and its dependencies. It can automatically install, update, and uninstall libraries, simplifying your project's dependency management process.
Practical example:
composer require laravel/framework
This will use Composer to install the Laravel framework and its dependencies.
5. Deployment Strategy
Choosing an appropriate deployment strategy is critical to ensuring high availability and fault tolerance of your project. Zero-downtime deployments (such as blue-green deployments) and failover strategies (such as autoscaling) can help you minimize downtime and keep your applications running.
Practical case:
For blue-green deployment, you can run your application in one environment (blue) while preparing another environment (green) . When the green environment is ready, you can switch traffic from the blue environment to the green environment without any downtime.
The above is the detailed content of Advanced skills in PHP project development. For more information, please follow other related articles on the PHP Chinese website!
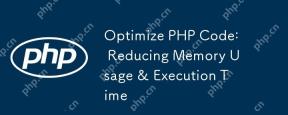
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
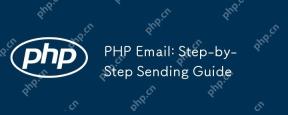
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
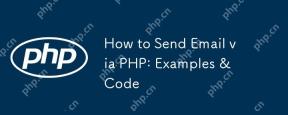
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
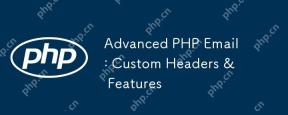
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
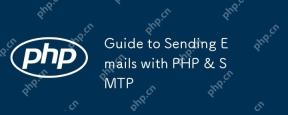
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
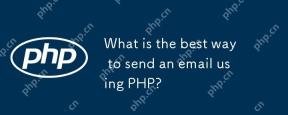
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
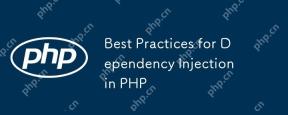
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
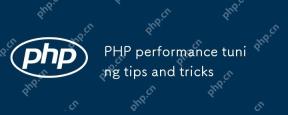
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
