


PHP database connection automation: Simplify management with scripts and tools
PHP provides solutions for automated database connections, including: using PDO to connect to different DBMS and perform queries and modifications; using scripts to regularly perform maintenance tasks, such as cleaning and optimization; using third-party tools such as phpMyAdmin and Doctrine DBAL to simplify management ;Use scripts and PDO to automate the backup process and create SQL files containing the database structure and data.
PHP Database Connection Automation: Use Scripts and Tools to Simplify Management
In modern web applications, database connection management is a a vital task. Managing connections manually can be complex and error-prone, especially when dealing with multiple databases or frequent connections. PHP provides a wide range of tools and scripts to automate database connections, simplifying management.
Using PHP Data Objects (PDO)
PDO is a PHP extension that provides a convenient and unified way to connect to different database management systems (DBMS ), such as MySQL, PostgreSQL and Oracle. By using PDO, you can use the same methods to connect, query, and modify different types of databases, simplifying development and maintenance.
Create PDO instance
To connect to the database, you need to create a PDO instance:
$dsn = 'mysql:host=localhost;dbname=my_database'; $user = 'root'; $password = 'secret'; $pdo = new PDO($dsn, $user, $password);
Perform queries and modifications
Using PDO, you can execute queries using the query()
method, and prepare and execute using the prepare()
and execute()
methods Parameterized queries. For example, executing a SELECT
query:
$sql = "SELECT * FROM users WHERE name = ?"; $stmt = $pdo->prepare($sql); $stmt->execute([$name]); $users = $stmt->fetchAll();
Using Script Automation
Writing scripts can further automate database connection management. For example, create the following script to periodically connect to the database and perform maintenance tasks:
<?php // 连接到数据库 $dsn = 'mysql:host=localhost;dbname=my_database'; $user = 'root'; $password = 'secret'; $pdo = new PDO($dsn, $user, $password); // 执行维护任务 $pdo->exec('VACUUM ANALYZE'); $pdo->exec('OPTIMIZE TABLE users'); // 关闭连接 $pdo = null; ?>
Simplify with Tools
There are also many third-party tools that simplify database connection management, such as:
- phpMyAdmin: A popular web interface that allows you to manage databases and perform queries.
- Doctrine DBAL: A PHP object-relational mapper (ORM) library that provides an object-oriented query interface.
Practical case: Backing up the database
Using PDO and a simple script, you can automate the database backup process:
<?php // 设置备份文件路径 $backup_file = 'backup.sql'; // 连接到数据库 $dsn = 'mysql:host=localhost;dbname=my_database'; $user = 'root'; $password = 'secret'; $pdo = new PDO($dsn, $user, password); // 提取数据库结构和数据 $sql = "SHOW TABLES"; $stmt = $pdo->query($sql); $tables = $stmt->fetchAll(PDO::FETCH_COLUMN); foreach ($tables as $table) { $stmt = $pdo->query("DESCRIBE $table"); $fields = $stmt->fetchAll(PDO::FETCH_COLUMN); $fp = fopen($backup_file, 'a+'); fwrite($fp, "CREATE TABLE $table (\n"); foreach ($fields as $field) { fwrite($fp, " $field\n"); } fwrite($fp, ");\n"); $stmt = $pdo->qurey("SELECT * FROM $table"); $data = $stmt->fetchAll(PDO::FETCH_ASSOC); foreach ($data as $row) { $values = implode(',', array_map(function ($value) { return "'$value'"; }, $row)); fwrite($fp, "INSERT INTO $table VALUES ($values);\n"); } fclose($fp); } // 关闭连接 $pdo = null; ?>
Run this script A SQL backup file containing the database structure and data will be created.
The above is the detailed content of PHP database connection automation: Simplify management with scripts and tools. For more information, please follow other related articles on the PHP Chinese website!
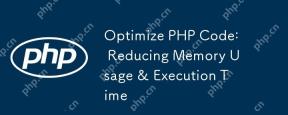
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
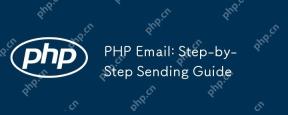
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
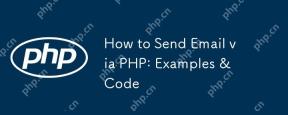
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
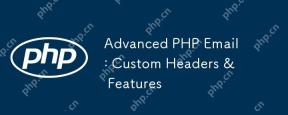
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
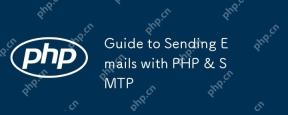
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
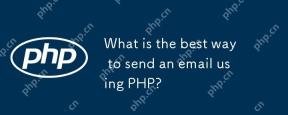
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
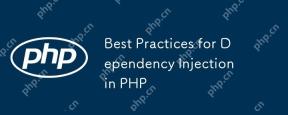
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
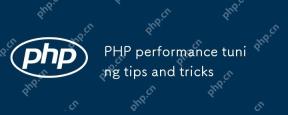
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
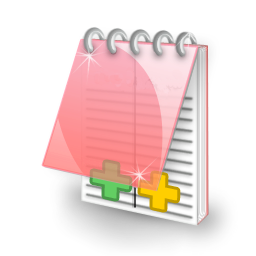
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
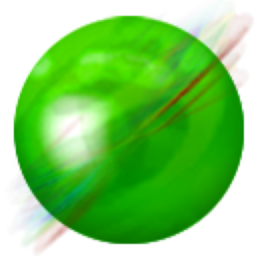
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
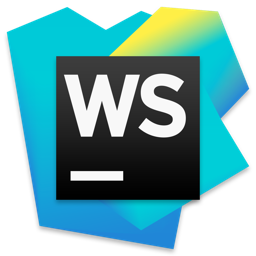
WebStorm Mac version
Useful JavaScript development tools
