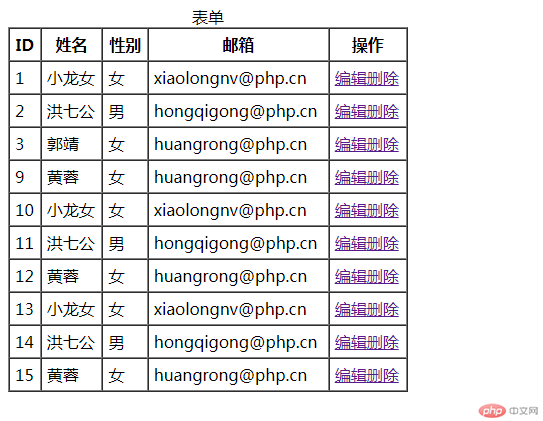
core文件夹中的文件(原始mvc)
controller.php
<?php
namespace shiyans;
class controller
{
protected $model;
protected $view;
public function __construct($model, $view)
{
$this -> model = $model;
$this -> view = $view;
}
public function show()
{
$data = $this ->model->getAll();
$this->view->display($data);
}
}
model.php
<?php
namespace shiyans;
use PDO;
class model
{
protected $db;
public function __construct($sdb, $username, $password)
{
$this->db=new PDO($sdb, $username, $password);
}
public function getAll($n = 10)
{
$stmt =$this ->db->prepare('SELECT * FROM `student` LIMIT ?');
$stmt ->bindParam(1, $n, PDO::PARAM_INT);
$stmt ->execute();
return $stmt->fetchAll();
}
}
view.php
<?php
namespace shiyans;
class view
{
public function display($data)
{
$tables = $data;
include FATE.'/view/'.'shiyan.php';
}
}
controller文件夹中的文件(自定义控制器,动态测试文件)
newcontroller.php(自定义控制器)
<?php
namespace shiyans;
class newcontroller extends controller
{
}
textcontroller.php
<?php
namespace shiyans;
class textcontroller
{
public function text()
{
return '<p>测试文件输出结果:Hello World</p>';
}
}
动态测试结果

model文件夹中的文件(自定义模型)
newmodel.php
<?php
namespace shiyans;
class newmodel extends model
{
}
view文件夹中的文件(视图)
shiyan.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<table border="1" cellspacing="0" cellpadding="5" width="400">
<caption>表单</caption>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>性别</th>
<th>邮箱</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<!-- <?php foreach ($tables as $table): ?>
<tr>
<td><?= $table['id'] ?>
</td>
<td><?= $table['name'] ?>
</td>
<td><?= $table['sex']?'女':'男' ?>
</td>
<td><?= $table['email'] ?>
</td>
<td><a href="">编辑</a><a href="">删除</a></td>
</tr>
<?php endforeach ?> -->
<?php foreach ($tables as [$id,$name,$sex,$email]): ?>
<tr>
<td><?= $id ?>
</td>
<td><?= $name ?>
</td>
<td><?= $sex?'女':'男' ?>
</td>
<td><?= $email ?>
</td>
<td><a href="">编辑</a><a href="">删除</a></td>
</tr>
<?php endforeach ?>
</tbody>
</table>
</body>
</html>
根目录里的文件(信息存储文件,串联实现功能文件)
config.php(信息存储文件)
<?php
// 信息储存文件
//数据库信息
define('DATABASE', [
'type' => 'mysql',
'dbname' => 'php',
'username' => 'root',
'password' => 'root'
]);
//文件目录信息
define('FILE', [
'new_controller' => 'new',
'new_action' =>'show'
]);
//载入文件信息
define(
'FATE',
__DIR__
);
index.php(实现文件)
<?php
namespace shiyans;
require __DIR__ . '/config.php';
require __DIR__ . '/core/controller.php';
require __DIR__ . '/core/model.php';
require __DIR__ . '/core/view.php';
require __DIR__ . '/model/newmodel.php';
//解构信息页面里的数组
extract(DATABASE);
//数据库连接
$sdn = sprintf('%s:dbname=%s', $type, $dbname);
$model = new newmodel($sdn, $username, $password);
//动态获取浏览器地址中的控制器及控制器方法,并设置默认值
$a = $_GET['a'] ?? FILE['new_action'];
$c = $_GET['c'] ?? FILE['new_controller'];
//输出预览是否获取
// echo $a,$c;
//加载获取类名
$class = $c.'controller';
require __DIR__ . '/controller/' .$class . '.php';
//加载类
$view = new view();
//获取控制器类名
$full = __NAMESPACE__.'\\' .$class;
//输出查看是否获取成功
// echo $full;
$controller = new $full($model, $view);
echo $controller ->$a();