1.表格基础知识
序号 |
标签 |
描述 |
1 |
<table> |
定义表格, 必选 |
2 |
<tr> |
定义表格中的行, 必选 |
3 |
<th> |
定义表格头部中的单元格, 必选 |
4 |
<td> |
定义表格主体中的单元格, 必选 |
序号 |
标签 |
描述 |
1 |
<option> |
定义表格标题, 可选 |
2 |
<thead> |
定义表格头格, 只需定义一次, 可选 |
3 |
<tbody> |
定义表格主体, 允许定义多次, 可选 |
4 |
<tfooter> |
定义表格底, 只需定义一次, 可选 |
序号 |
属性 |
所属标签 |
描述 |
1 |
border |
<table> |
添加表格框 |
2 |
cellpadding |
<table> |
设置单元格内边距 |
2 |
cellspacing |
<table> |
设置单元格边框间隙 |
2 |
align |
不限 |
设置单元格内容水平居中 |
2 |
bgcolor |
不限 |
设置背景色 |
2.表格制作购物车实战页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>表格实战:购物车</title>
<style>
/* 设置表格字体大小 */
table {
font-size: 14px;
}
/* 将单元格之间间隙去掉 */
table {
border-collapse: collapse;
width: 70%;
margin: auto;
color: #888;
font-size: larger;
text-align: center;
}
td,
th {
border-bottom: 1px solid #ccc;
padding: 10px;
}
/* 标题样式 */
table caption {
font-size: 1.5rem;
color: yellowgreen;
margin-bottom: 20px;
}
table th {
font-weight: bolder;
color: white;
}
table thead tr:first-of-type {
background-color: teal;
}
/* 隔行变色 */
table tbody tr:nth-of-type(even) {
background-color: yellowgreen;
color: white;
}
/* 鼠标悬停效果 */
table tbody tr:hover {
background-color: violet;
color: black;
}
/* 表格底部样式 */
table tfoot td {
border-bottom: none;
color: red;
font-size: 1.2rem;
}
/* 结算按钮 */
body div:last-of-type {
width: 70%;
margin: 10px auto;
}
body div:first-of-type button {
/* 右浮动,靠右 */
float: right;
width: 120px;
height: 32px;
background-color: seagreen;
color: white;
border: none;
/* 设置鼠标样式 */
cursor: pointer;
}
body div:first-of-type button:hover {
background-color: sandybrown;
font-size: 1.1rem;
}
</style>
</head>
<body>
<!-- 表格 -->
<table>
<!-- 标题 -->
<caption>
购物车
</caption>
<!-- 表格头部 -->
<thead>
<tr>
<th>ID</th>
<th>品名</th>
<th>单价/元</th>
<th>单位</th>
<th>数量</th>
<th>金额/元</th>
</tr>
</thead>
<!-- 表格主体 -->
<tbody>
<tr>
<td>SN-1010</td>
<td>MacBook Pro电脑</td>
<td>18999</td>
<td>台</td>
<td>1</td>
<td>18999</td>
</tr>
<tr>
<td>SN-1020</td>
<td>iPhone手机</td>
<td>4999</td>
<td>部</td>
<td>2</td>
<td>9998</td>
</tr>
<tr>
<td>SN-1030</td>
<td>智能AI音箱</td>
<td>399</td>
<td>只</td>
<td>5</td>
<td>1995</td>
</tr>
<tr>
<td>SN-1040</td>
<td>SSD移动硬盘</td>
<td>888</td>
<td>个</td>
<td>2</td>
<td>1776</td>
</tr>
<tr>
<td>SN-1050</td>
<td>黄山毛峰</td>
<td>999</td>
<td>斤</td>
<td>3</td>
<td>2997</td>
</tr>
</tbody>
<!-- 表格尾部 -->
<tfoot>
<td colspan="4">总计:</td>
<td>13</td>
<td>35765</td>
</tfoot>
</table>
<!-- 结算按钮 -->
<div>
<button>结算</button>
</div>
</body>
</html>
运行结果截图:
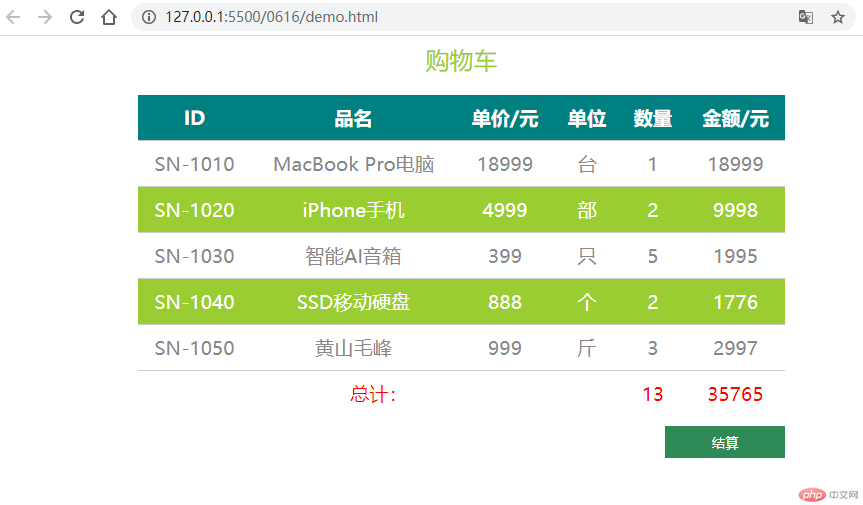
3.表单
序号 |
元素 |
名称 |
描述 |
1 |
<form> |
表单容器 |
表单应该放在该标签内提交 |
2 |
<input> |
输入控件 |
由type 属性指定控件类型 |
3 |
<label> |
控件标签 |
用于控件功能描述与内容关联 |
4 |
<select> |
下拉列表 |
用于选择预置的输入内容 |
5 |
<datalist> |
预置列表 |
用于展示预置的输入内容 |
6 |
<option> |
预置选项 |
与<select> ,<datalist> 配合 |
7 |
<textarea> |
文本域 |
多行文本框,常与富文本编辑器配合 |
8 |
<button> |
按钮 |
用于提交表单 |
表单元素的公共属性(并非所有元素都具备)
序号 |
属性 |
描述 |
1 |
name |
元素/控件名称,用做服务器端脚本的变量名称 |
2 |
value |
提交到服务器端的数据 |
3 |
placeholder |
输入框的提示信息 |
4 |
form |
所属的表单,与<form name=""> 对应 |
5 |
autofocus |
页面加载时,自动获取焦点 |
6 |
required |
必填 / 必选项 |
7 |
readonly |
该控件内容只读 |
8 |
disabled |
是否禁用 |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>基本表单元素</title>
</head>
<body>
<h3>用户注册</h3>
<!-- form+input -->
<form action="" method="POST">
<fieldset>
<legend>基本信息(必填)</legend>
<div>
<label for="username">账号:</label>
<input
type="text"
id="my-username"
name="username"
placeholder="6-15位字符"
/>
</div>
<div>
<label for="email-id">邮箱:</label>
<input
type="email"
name="email"
id="email-id"
placeholder="demo@qq.com"
required
/>
</div>
<!-- 密码 框-->
<div>
<label for="pwd-1">密码:</label>
<input
type="password"
name="password1"
id="pwd-2"
placeholder="不少于6位且字母+数字"
/>
<!-- 点击显示密码 -->
<!-- <button onclick="ShowPsw()" id="btn" type="button">显示密码</button> -->
</div>
<div>
<label for="pwd-2">确认:</label>
<input type="password" name="password2" id="pwd-1" />
</div>
</fieldset>
<fieldset>
<legend>扩展信息(选填)</legend>
<div>
<label
>生日:
<input type="date" name="birthday" />
</label>
</div>
<!-- 单选按钮 -->
<div>
<label for="secret">性别:</label>
<!-- 单选按钮中在name属性名必须相同 -->
<input type="radio" value="male" name="gender" id="male" /><label
for=""
>男</label
>
<input type="radio" value="female" name="gender" id="female" /><label
for=""
>女</label
>
<input
type="radio"
value="secret"
name="gender"
id="secret"
checked
/><label for="secret">保密</label>
</div>
<!-- 复选框 -->
<div>
<label for="programme">爱好</label>
<!-- 因为复选框返回是一个或多个值,最方便后端用数组来处理, 所以将name名称设置为数组形式便于后端脚本处理 -->
<input type="checkbox" name="hobby[]" id="game" value="game" />
<label for="game">打游戏</label>
<input type="checkbox" name="hobby[]" id="shoot" value="shoot" />
<label for="game">编程</label>
<input
type="checkbox"
name="hobby[]"
id="programme"
value="programme"
checked
/>
<label for="game">编程</label>
</div>
<!-- 选项列表 -->
<div>
<label for="brand">手机:</label>
<input type="search" list="phone" id="brand" name="brand" />
<datalist id="phone">
<option value="apple"> </option>
<option value="huawei">华为</option>
<option value="mi" label="小米"> </option>
</datalist>
</div>
</fieldset>
<fieldset>
<legend>其它信息(选填)</legend>
<!--文件上传-->
<div>
<label for="uploads">上传头像:</label>
<input
type="file"
name="user_pic"
id="uploads"
accept="image/png, image/jpeg, image/gif"
/>
</div>
<!--文本域-->
<div>
<label for="resume">简历:</label>
<!--注意文本域没有value属性-->
<textarea
name="resume"
id="resume"
cols="30"
rows="5"
placeholder="不超过100字"
></textarea>
</div>
</fieldset>
<!-- 隐藏域, 例如用户的Id, 注册,登录的时间,无需用户填写 -->
<input type="hidden" name="user_id" value="123" />
<p>
<input type="submit" value="提交" class="btn" />
<button class="btn">提交</button>
</p>
</form>
<script>
//输入密码,点击名文显示
// function ShowPsw(ele) {
// const pwd = document.querySelector("#pwd-2");
// pwd.type = "text";
// 代码写成一行
// document.querySelector("#pwd-2").type = "text";
// }
</script>
</body>
</html>
运行结果截图
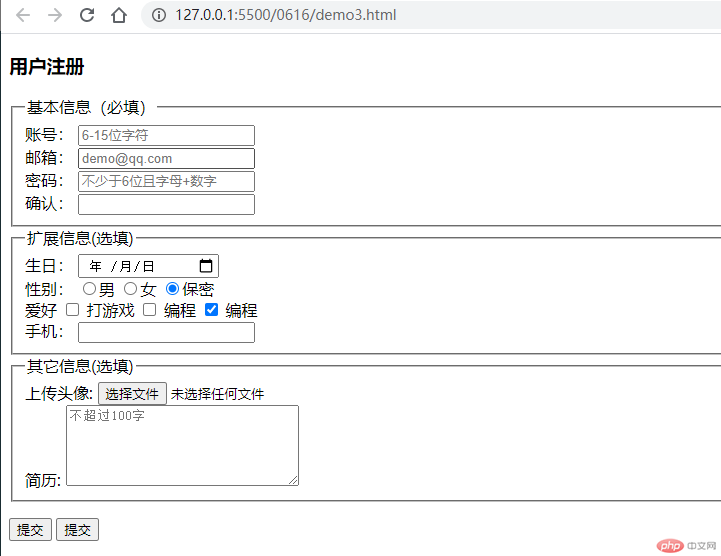
<style>
body {
color: #555;
}
h3 {
text-align: center;
}
form {
width: 450px;
margin: 30px auto;
border-top: 1px solid #aaa;
}
form fieldset {
border: 1px solid seagreen;
background-color: lightcyan;
box-shadow: 2px 2px 4px #bbb;
border-radius: 10px;
margin: 20px;
}
form fieldset legend {
background-color: rgb(178, 231, 201);
border-radius: 10px;
color: seagreen;
padding: 5px 15px;
}
form div {
margin: 5px;
}
form p {
text-align: center;
}
form .btn {
width: 80px;
height: 30px;
border: none;
background-color: seagreen;
color: #ddd;
}
form .btn:hover {
background-color: coral;
color: white;
cursor: pointer;
}
input:focus {
background-color: rgb(226, 226, 175);
}
</style>
运行结果截图
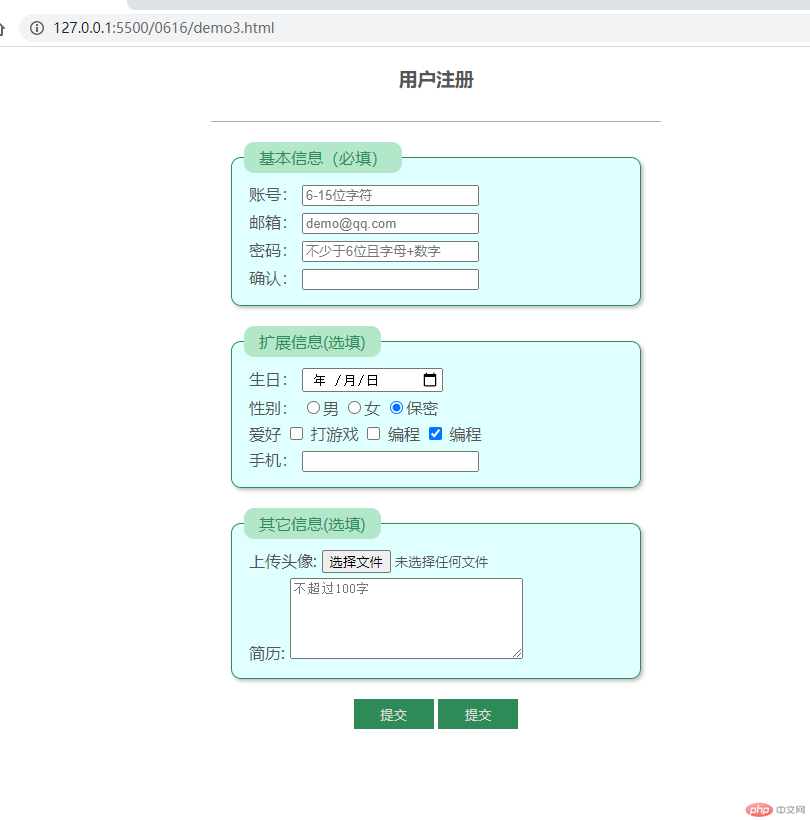
4.总结
- 表格是数据格式化的工具
- 完整表格 9 个标签:( table + caption + colgroup + thead + tbody + tfoot + tr + th + td)
- 表格常用 7 个标签:table+capiton+thead+tbody+tr+th+tr+td
- 最简单表格要用的 6 个标签:table+caption+tbody+tr+th/td
- 表单是网站页面与用户之间实现互动一种工具,通常用来实现用户的注册登陆,信息收集
- 表单元素添加
disabled
禁用属性的字段数据不会被提交,但是readonly
只读属性的字段允许提交 - 表单元素隐藏域的内容不会在 HTML 页面中显示,但是
value
属性的数据会被提交