Web 前端 - CSS - 选择器
一、简单选择器
序号 |
选择器 |
描述 |
格式 |
举例 |
1 |
元素选择器 |
根据元素标签名称进行匹配 |
ele_name |
div {...} |
2 |
属性选择器 |
根据元素属性进行匹配 |
ele_name[property] |
*[...] |
3 |
类选择器 |
根据元素 class 属性进行匹配 |
.ele_class_name |
*.active {...} |
4 |
id 选择器 |
根据元素 id 属性进行匹配 |
#ele_id |
*#top {...} |
5 |
群组选择器 |
同时选择多个不同类型的元素 |
e1, e2, e3... |
h1,h2,h3{...} |
6 |
通配选择器 |
选择全部元素,不区分类型 |
* |
* {...} |
二、上下文选择器
序号 |
选择器 |
操作符 |
描述 |
举例 |
1 |
后代选择器 |
空格 |
选择当前元素的所有后代元素,递归 |
div p , body * |
2 |
父子选择器 |
> |
选择当前元素的所有子元素,仅二级 |
div > h2 |
3 |
同级相邻选择器 |
+ |
选择拥有共同父级且相邻的元素,该元素紧接着的下一个元素 |
li.red + li |
4 |
同级所有选择器 |
~ |
选择拥有共同父级的后续所有元素,该元素紧接着的后面的所有元素 |
li.red ~ li |
序号 |
角色 |
描述 |
1 |
祖先元素 |
拥有子元素,孙元素等所有层级的后代元素 |
2 |
父级元素 |
仅拥有子元素层级的元素 |
3 |
后代元素 |
与其它层级元素一起拥有共同祖先元素 |
4 |
子元素 |
与其它同级元素一起拥有共同父级元素 |
三、伪类选择器
1. 结构伪类
1.1 不分组匹配
序号 |
选择器 |
描述 |
举例 |
1 |
:first-child |
匹配第一个子元素 |
div :first-child |
2 |
:last-child |
匹配最后一个子元素 |
div :last-child |
3 |
:only-child |
选择元素的唯一子元素 |
div :only-child |
4 |
:nth-child(n) |
匹配任意位置的子元素 |
div :nth-child(n) |
5 |
:nth-last-child(n) |
匹配倒数任意位置的子元素 |
div :nth-last-child(n) |
1.2 分组匹配
序号 |
选择器 |
描述 |
举例 |
1 |
:first-of-type |
匹配按类型分组后的第一个子元素 |
div :first-of-type |
2 |
:last-of-type |
匹配按类型分组后的最后一个子元素 |
div :last-of-type |
3 |
:only-of-type |
匹配按类型分组后的唯一子元素 |
div :only-of-type |
4 |
:nth-of-type(n) |
匹配按类型分组后的任意位置的子元素 |
div :nth-of-type(n) |
5 |
:nth-last-of-type(n) |
匹配按类型分组后倒数任意位置的子元素 |
div :nth-last-of-type(n) |
1.3 n支持表达式
序号 |
表达式 |
描述 |
举例 |
1 |
2n/even |
代表所有偶数 |
div :nth-child(even)/div :nth-of-type(even) |
2 |
2n-1/odd |
代表所有奇数 |
div :nth-child(odd)/div :nth-of-type(odd) |
3 |
-n + n |
代表前n个 |
div :nth-child(-n + 3)/div :nth-of-type(-n + 3) |
4 |
n + n |
代表从第n个开始一直到最后一个 |
div :nth-child(n + 3)/div :nth-of-type(n + 3) |
2. 其他伪类
序号 |
选择器 |
描述 |
1 |
:active |
向被激活的元素添加样式 |
2 |
:focus |
向拥有键盘输入焦点的元素添加样式 |
3 |
:hover |
当鼠标悬浮在元素上方时,向元素添加样式 |
4 |
:link |
向未被访问的链接添加样式 |
5 |
:visited |
向已被访问的链接添加样式 |
5 |
:root |
根元素,通常是html |
5 |
:empty |
选择没有任何子元素的元素(含文本节点) |
5 |
:not() |
排除与选择器参数匹配的元素 |
四、选择器的优先级
- 内联 > ID选择器 > 类选择器 > 标签选择器
五、代码示例
<!DOCTYPE html>
<html lang="zh_hans">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>选择器</title>
<style>
/*元素选择器*/
div {
color: saddlebrown;
}
span {
color: slateblue;
}
/*属性选择器*/
div[title="div"] {
color: teal;
}
/*类选择器*/
.s {
width: 20px;
height: 20px;
background-color: wheat;
}
/*id选择器*/
#box {
width: 200px;
height: 200px;
background-color: lightgreen;
}
#nav {
margin-left: 100px;
}
/*群组选择器*/
.d, .s {
font-size: larger;
}
/*通配选择器*/
* {
margin: 0;
padding: 0;
}
/*后代选择器*/
#box div {
font-family: 'Franklin Gothic Medium', 'Arial Narrow', Arial, sans-serif;
}
/*父子选择器*/
#box > span {
font-family: Cambria, Cochin, Georgia, Times, 'Times New Roman', serif;
}
/*同级相邻选择器*/
.d[title="div"] + div {
color: lightsteelblue;
}
/*同级所有选择器*/
.s[title="span"] ~ span {
color: magenta;
}
/*:first-child选择器*/
#box :first-child {
background-color: mediumpurple;
}
/*:last-child选择器*/
#box :last-child {
display: inline-block;
background-color: palevioletred;
}
/*:only-child选择器*/
#p :only-child {
color: gray;
}
/*:nth-child(n)选择器*/
#box :nth-child(3) {
background-color: hotpink;
}
#box :nth-child(-n + 2) {
margin-left: 50px;
}
/*:nth-last-child(n)选择器*/
#box :nth-last-child(2) {
margin-left: 20px;
}
/*:first-of-type选择器*/
#box span:first-of-type {
color: lightblue;
}
/*:last-of-type选择器*/
#box span:last-of-type {
color: lightblue;
}
/*:only-of-type选择器*/
#p p:only-of-type {
width: 100px;
background-color: lightcoral;
}
/*:nth-of-type(n)选择器*/
#box span:nth-of-type(3) {
color: lightgray;
}
#box span:nth-of-type(n + 2) {
background-color: lightsteelblue;
}
/*:nth-last-of-type(n)选择器*/
#box div:nth-last-of-type(1) {
background-color: mediumpurple;
}
/*:hover选择器*/
ul li:hover > a {
text-decoration: none;
}
</style>
</head>
<body>
<div id="box">
<div class="d" title="div">d1</div>
<div class="d">d2</div>
<div class="d">d3</div>
<div class="d">d4</div>
<span class="s">s1</span>
<span class="s" title="span">s2</span>
<span class="s">s3</span>
<span class="s">s4</span>
</div>
<div id="p"><p>p1</p></div>
<div id="nav">
<ul>
<li><a href="#">a1</a></li>
<li><a href="#">a2</a></li>
<li><a href="#">a3</a></li>
<li><a href="#">a4</a></li>
</ul>
</div>
</body>
</html>
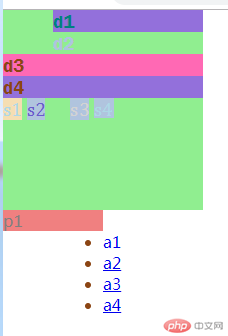
六、课程总结
- 今天学习了 CSS 常用的选择器,通过上课认真听讲和认真完成老师布置的作业,使得我对 CSS 的理解和运用更加深入和熟悉。最主要的知识点是明白了选择器都有哪些类型以及上下文选择器和伪类选择器各自的特点,以及了解并熟悉了常见的 css 选择器诸如上下文选择器和伪类选择器的用法。