Use smart pointers in C++ to prevent memory leaks
Smart pointers are special pointers used to prevent C memory leaks. They can automatically release the memory they manage, eliminating the possibility of memory leaks. The C standard library provides two main types of smart pointers: std::unique_ptr
Use smart pointers in C to prevent memory leaks
Memory leaks are a common trap in C, which will increase over time leads to serious performance issues. A memory leak occurs when a program incorrectly holds a reference to memory after it is no longer needed. This can lead to wasted memory, program crashes, and other unpredictable behavior.
Smart pointer
A smart pointer is a special pointer in C that is responsible for managing the lifetime of the memory pointed to. Smart pointers automatically release the memory they manage, eliminating the possibility of memory leaks.
The C standard library provides two main types of smart pointers:
-
std::unique_ptr<t></t>
: used to manage a uniquely owned object. Onceunique_ptr
is destroyed, the memory it points to will be automatically released. -
std::shared_ptr<t></t>
: Used to manage an object with shared ownership. Multipleshared_ptr
can point to the same block of memory, and the memory will only be released when allshared_ptr
have been destroyed.
Practical case
Consider the following code, which uses raw pointers to manage a Foo
object:
Foo* foo = new Foo(); // ... 使用 foo ... delete foo; // 手动释放 foo
If you forget to call delete foo
, a memory leak will occur.
Use smart pointers to avoid this situation:
std::unique_ptr<Foo> foo(new Foo()); // ... 使用 foo ...
unique_ptr
will automatically release the Foo
object when it goes out of scope. This ensures that memory is always released when no longer needed.
Conclusion
Using smart pointers is an effective way to prevent memory leaks in C. They automatically manage memory lifetime, eliminating the possibility of manual memory management errors. By avoiding memory leaks, programs can remain efficient and stable.
The above is the detailed content of Use smart pointers in C++ to prevent memory leaks. For more information, please follow other related articles on the PHP Chinese website!
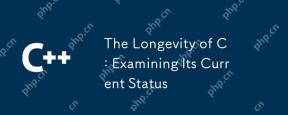
C is still important in modern programming because of its efficient, flexible and powerful nature. 1)C supports object-oriented programming, suitable for system programming, game development and embedded systems. 2) Polymorphism is the highlight of C, allowing the call to derived class methods through base class pointers or references to enhance the flexibility and scalability of the code.
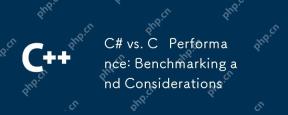
The performance differences between C# and C are mainly reflected in execution speed and resource management: 1) C usually performs better in numerical calculations and string operations because it is closer to hardware and has no additional overhead such as garbage collection; 2) C# is more concise in multi-threaded programming, but its performance is slightly inferior to C; 3) Which language to choose should be determined based on project requirements and team technology stack.
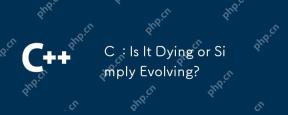
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
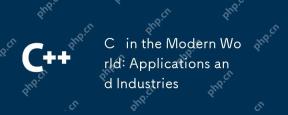
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
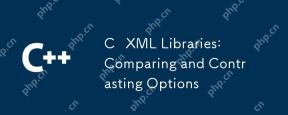
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
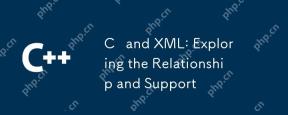
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
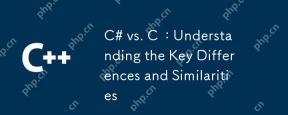
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
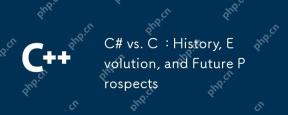
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
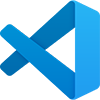
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
