There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1. TinyXML-2 is suitable for resource-limited environments, lightweight but limited in functionality. 2. PugiXML is fast and supports XPath queries, suitable for complex XML structures. 3. Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
introduction
In C programming, processing XML files is one of the common tasks. Whether you need to parse XML data or generate XML documents, choosing a suitable library is crucial. This article aims to make in-depth comparisons and comparisons of commonly used XML libraries in C, so that you can make wise choices when facing different needs. By reading this article, you will learn about the characteristics, advantages and disadvantages of different libraries, and their performance in practical applications.
Review of basic knowledge
XML (Extensible Markup Language) is a format used to store and transfer data, and it is widely used in web services, configuration files, and data exchange. As an efficient programming language, C provides a variety of libraries to process XML data. Understanding the basic functions and usage scenarios of these libraries is the basis for us to start comparing.
Common C XML libraries include:
- TinyXML-2 : A lightweight XML parser for resource-limited environments.
- PugiXML : A fast and easy-to-use XML parsing library that supports XPath queries.
- Xerces-C : A powerful XML parser developed by the Apache Software Foundation and supports DOM and SAX parsing.
- RapidXML : A very fast XML parsing library focusing on performance.
Core concept or function analysis
XML parsing and generation
The main function of the XML library is to parse and generate XML documents. The parsing process involves converting an XML file into a data structure in memory, while the generation process is the opposite. Different libraries have their own characteristics and advantages and disadvantages when implementing these functions.
TinyXML-2
TinyXML-2 is a very lightweight library suitable for use in environments with limited memory and CPU resources. Its API is simple to use, but its functionality is relatively limited.
#include "tinyxml2.h" <p>int main() { tinyxml2::XMLDocument doc; doc.LoadFile("example.xml");</p><pre class='brush:php;toolbar:false;'> tinyxml2::XMLElement* root = doc.RootElement(); if (root) { const char* text = root->GetText(); printf("Root text: %s\n", text); } return 0;
}
The advantage of TinyXML-2 is its compactness and ease of use, but the disadvantage is that it does not support XPath queries and complex XML operations.
PugiXML
PugiXML is known for its speed and ease of use. It supports XPath queries, which is very useful when dealing with complex XML structures.
#include "pugixml.hpp" <p>int main() { pugi::xml_document doc; pugi::xml_parse_result result = doc.load_file("example.xml");</p><pre class='brush:php;toolbar:false;'> pugi::xpath_node_set nodes = doc.select_nodes("//item"); for (auto& node : nodes) { pugi::xml_node item = node.node(); printf("Item: %s\n", item.child_value()); } return 0;
}
The advantages of PugiXML are its speed and XPath support, but it may experience memory problems when dealing with very large XML files.
Xerces-C
Xerces-C is a powerful XML parser that supports DOM and SAX parsing modes. It is suitable for scenarios where complex XML processing is required.
#include<xercesc> #include<xercesc><p> int main() { xercesc::XMLPlatformUtils::Initialize();</p><pre class='brush:php;toolbar:false;'> xercesc::XercesDOMParser* parser = new xercesc::XercesDOMParser(); parser->parse("example.xml"); xercesc::DOMDocument* doc = parser->getDocument(); xercesc::DOMElement* root = doc->getDocumentElement(); xercesc::DOMNodeList* nodes = root->getElementsByTagName(X("item")); for (XMLSize_t i = 0; i < nodes->getLength(); i ) { xercesc::DOMElement* item = dynamic_cast<xercesc::DOMElement*>(nodes->item(i)); char* text = xercesc::XMLString::transcode(item->getTextContent()); printf("Item: %s\n", text); xercesc::XMLString::release(&text); } delete parser; xercesc::XMLPlatformUtils::Terminate(); return 0;
}
The advantages of Xerces-C are its powerful and flexible functions, but its disadvantages are its complexity and large dependency libraries.
RapidXML
RapidXML focuses on performance and its parsing speed is very fast and is suitable for scenarios where XML is processed efficiently.
#include "rapidxml/rapidxml.hpp" #include "rapidxml/rapidxml_utils.hpp" <p>int main() { rapidxml::file xmlFile("example.xml"); rapidxml::xml_document doc; doc.parse(xmlFile.data());</p><pre class='brush:php;toolbar:false;'> for (rapidxml::xml_node<>* node = doc.first_node("item"); node; node = node->next_sibling("item")) { printf("Item: %s\n", node->value()); } return 0;
}
The advantage of RapidXML is its extremely high parsing speed, but the disadvantage is that it does not support XPath queries and complex XML operations.
How it works
Different XML libraries adopt different strategies and data structures when parsing and generating XML. TinyXML-2 and PugiXML use DOM (Document Object Model) parsing method to load the entire XML document into memory for operation. Xerces-C supports two parsing modes: DOM and SAX (simple API for XML). SAX parsing can stream XML files, which is suitable for processing large XML files. RapidXML also uses DOM parsing, but its implementation is lighter and more efficient.
RapidXML and PugiXML generally perform better when it comes to performance because they focus on parsing speed and memory efficiency. Although Xerces-C is powerful, its parsing speed and memory consumption are relatively high. TinyXML-2 performs well in resource-limited environments, but has limited functionality.
Example of usage
Basic usage
Each library has its basic methods of parsing and generating XML files. Here is an example of using TinyXML-2 to generate a simple XML file:
#include "tinyxml2.h" <p>int main() { tinyxml2::XMLDocument doc; tinyxml2::XMLElement* root = doc.NewElement("root"); doc.InsertFirstChild(root);</p><pre class='brush:php;toolbar:false;'> tinyxml2::XMLElement* item = doc.NewElement("item"); item->SetText("Example"); root->InsertEndChild(item); doc.SaveFile("output.xml"); return 0;
}
Advanced Usage
For scenarios where complex operations are required, PugiXML's XPath query function is very useful. Here is an example of using PugiXML for XPath query:
#include "pugixml.hpp" <p>int main() { pugi::xml_document doc; pugi::xml_parse_result result = doc.load_file("example.xml");</p><pre class='brush:php;toolbar:false;'> pugi::xpath_node_set nodes = doc.select_nodes("//item[@id='1']"); for (auto& node : nodes) { pugi::xml_node item = node.node(); printf("Item with id 1: %s\n", item.child_value()); } return 0;
}
Common Errors and Debugging Tips
Common errors when using XML libraries include:
- Parse error : The XML file format is incorrect, resulting in parsing failure. Debug using the error message provided by the library.
- Memory Leaks : Especially when using DOM parsing, make sure the memory is freed correctly.
- XPath query error : The XPath expression is incorrect, causing the query to fail. Double-check the XPath expression to ensure its correctness.
Debugging skills include:
- Using the debugger : Set breakpoints in the code and debug the XML parsing and generation process step by step.
- Logging : Record key steps and error messages to help locate problems.
- Test cases : Write test cases to ensure the correctness of the library in different scenarios.
Performance optimization and best practices
In practical applications, it is very important to optimize the performance of XML processing. Here are some optimization suggestions:
- Choose the right library : Choose the right library according to specific needs. For example, if efficient parsing is required, choose RapidXML; if complex operations are required, choose Xerces-C.
- Using SAX parsing : For large XML files, using SAX parsing can reduce memory consumption.
- Avoid unnecessary memory allocation : When using DOM parsing, minimize unnecessary memory allocation and copy operations.
Best practices include:
- Code readability : Use clear naming and annotation to improve the readability of the code.
- Error handling : Write robust error handling code to ensure that the program can handle errors correctly when encountering errors.
- Modular design : encapsulate XML processing logic into independent modules to improve the maintainability of the code.
By comparing and analyzing commonly used XML libraries in C, we can better understand their characteristics and applicable scenarios. In actual projects, selecting the appropriate library according to specific needs and following best practices can greatly improve the efficiency and reliability of XML processing.
The above is the detailed content of C XML Libraries: Comparing and Contrasting Options. For more information, please follow other related articles on the PHP Chinese website!
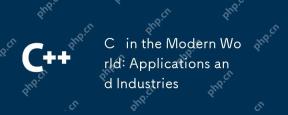
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
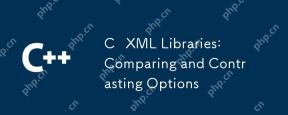
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
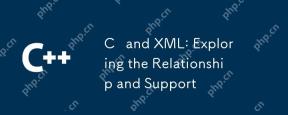
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
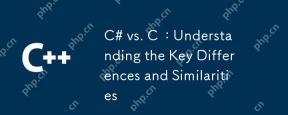
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
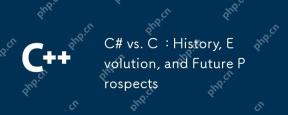
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
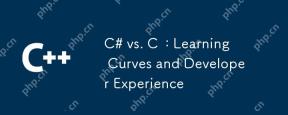
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
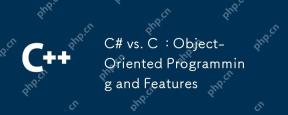
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
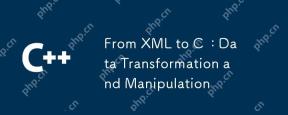
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),