Automatic garbage collection in C++ memory management
Automatic garbage collection in C requires the use of third-party tools or libraries. You can use smart pointers or garbage collector libraries. Smart pointers automatically release the underlying objects, and the garbage collector library uses algorithms to keep track of data structures that are no longer used. Case: Use smart pointer std::shared_ptr; use libgc library GC_MALLOC and GC_FREE.
Automatic garbage collection in C
Implementing automatic garbage collection in C requires the use of third-party tools or libraries. Although there is no built-in garbage collector in the C standard library, there are several options for achieving similar behavior.
Library options:
- Smart pointers: This is the most common C garbage collection method. A smart pointer is a special type of pointer that is responsible for automatically releasing the underlying object at the end of its lifetime.
- Garbage collector library: There is a library that can provide automatic garbage collection for C programs. These libraries typically use reference counting or mark-and-sweep algorithms to keep track of data structures that are no longer in use.
Practical case:
Use smart pointers:
#include <memory> std::shared_ptr<int> ptr = std::make_shared<int>(10);
std::shared_ptr
is a smart pointer that keeps track of the number of references to its underlying object. The object is automatically released when it is no longer referenced by any pointers.
Use third-party garbage collection library:
libgc: This is a widely used garbage collection library in C. It uses a mark-and-sweep algorithm to identify data structures that are no longer used.
#include "gc/gc.h" int* ptr = (int*)GC_MALLOC(sizeof(int)); *ptr = 10; GC_FREE(ptr);
When GC_FREE
is called, the memory pointed to by ptr
will be marked as no longer used and will be recycled in the next garbage collection cycle.
The above is the detailed content of Automatic garbage collection in C++ memory management. For more information, please follow other related articles on the PHP Chinese website!
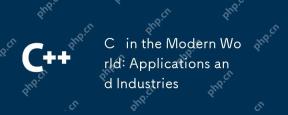
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
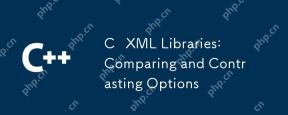
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
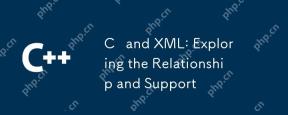
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
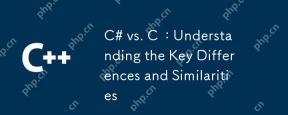
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
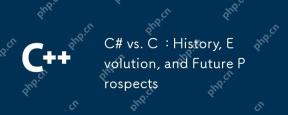
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
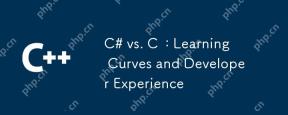
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
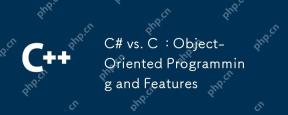
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
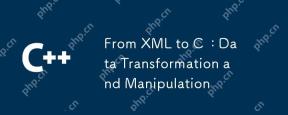
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
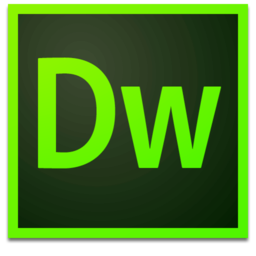
Dreamweaver Mac version
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
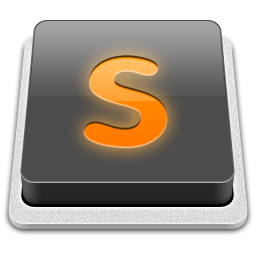
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use