Golang function cache hotkey processing strategy revealed
Question: How to use hotkey handling strategy to improve function cache performance in Go? Specific strategy: Use hotkey caching to identify frequently called functions. Store frequently called functions in a quick access area. When the frequently called function is called again, it is obtained directly from the fast access area to reduce cache overhead.
Go Function Cache: Hotkey Handling Strategy Revealed
In Go, function caching is widely used to improve application performance , but when a large number of functions are called frequently, the performance of the cache may be affected. To solve this problem, we can use hotkey handling strategy.
Strategy Overview
The purpose of the hotkey processing strategy is to identify and process frequently called functions and cache them into a separate quick access area. In this way, when these functions are called again, they can be quickly fetched from this fast access area, thereby reducing cache overhead.
Implementation Example
In Go, we can use the sync.Map
type to implement the hotkey handling strategy. Here is an example:
import "sync" // 定义热键缓存 var hotKeyCache sync.Map // 定义函数缓存 var funcCache sync.Map // 添加函数到热键缓存 func AddToHotKeyCache(key string, fn interface{}) { hotKeyCache.Store(key, true) } // 从缓存中获取函数 func GetFromCache(key string) (interface{}, bool) { // 检查热键缓存 if _, ok := hotKeyCache.Load(key); ok { return funcCache.Load(key) } // 从函数缓存中获取 return funcCache.Load(key) } // 增加函数调用次数 func IncrementCallCount(key string) { // 检查热键缓存 if _, ok := hotKeyCache.Load(key); ok { return } // 如果函数调用次数达到某个阈值,将其添加到热键缓存 if callCount := funcCache.Store(key, callCount + 1); callCount >= 10 { AddToHotKeyCache(key, funcCache.Load(key)) } }
Practical case
Suppose we have a function that calculates Fibonacci numbers:
func fib(n int) int { if n == 0 || n == 1 { return 1 } return fib(n-1) + fib(n-2) }
If called in large numbersfib
function, we can use the above strategy to optimize its performance:
// 将 fib 函数添加到缓存 funcCache.Store("fib", fib) // 为 fib 函数增加调用次数 func IncrementFibCallCount() { IncrementCallCount("fib") }
Every time the fib
function is called, the IncrementCallCount
function will increase the number of function calls frequency. When the number of calls reaches a certain threshold (such as 10), the fib
function will be added to the hotkey cache, providing faster access for subsequent calls.
The above is the detailed content of Golang function cache hotkey processing strategy revealed. For more information, please follow other related articles on the PHP Chinese website!
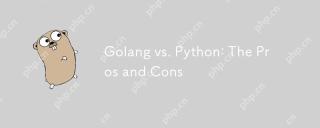
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
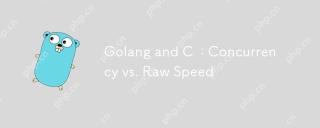
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
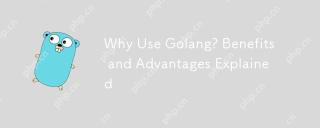
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
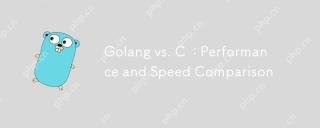
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
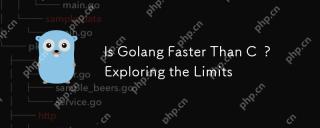
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
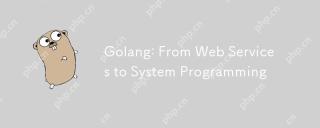
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
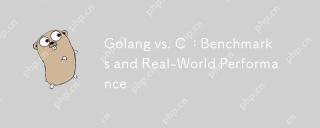
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
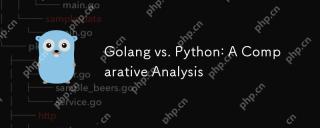
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
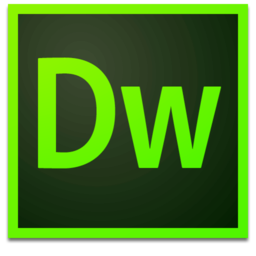
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
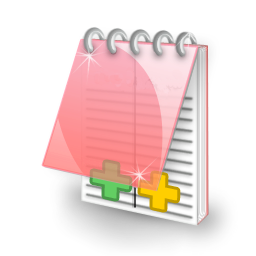
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function