Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1. Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.
introduction
In today's rapidly evolving programming world, choosing the right programming language is often the key to project success. As the best in modern programming languages, Golang and Python are each shining in different scenarios. This article aims to make an in-depth comparison of Golang and Python, from their features, usage scenarios to performance, and help you make informed choices. After reading this article, you will have a more comprehensive understanding of the pros and cons of both languages and be able to better decide which language to use in future projects.
Review of basic knowledge
Golang, the full name Go language, was released by Google in 2009. The main design goal is to improve programming efficiency and development speed. It is known for its concurrent programming capabilities and efficient compilation speed. Python is a high-level programming language developed by Guido van Rossum in the late 1980s. It is known for its concise syntax and a strong library ecosystem, especially in the fields of data science and machine learning.
Both support cross-platform development, but Golang is more inclined to system-level programming, while Python is more suitable for rapid prototyping and scripting tasks.
Core concept or function analysis
Language Characteristics and Design Philosophy
Golang's design philosophy emphasizes simplicity and efficiency. The language itself does not have complex characteristics such as inheritance and exception handling, and aims to reduce the cognitive burden of developers. Its concurrency model is based on CSP (Communicating Sequential Processes), and realizes efficient concurrent programming through goroutine and channel.
Python's design philosophy emphasizes "explicit over implicit", and is known for its "Pythonic" coding style. Python supports a variety of programming paradigms, including object-oriented, functional programming, etc. Its dynamic type system and rich libraries allow developers to quickly get started and implement complex functions.
How it works
Golang is extremely fast to compile and generates relatively small binary files, thanks to its static typing system and garbage collection mechanism. Golang's concurrency model allows developers to easily write efficient concurrent programs, but it should be noted that too many goroutines may lead to performance degradation.
Python's explanatory features make it run relatively slow, but its dynamic typing system and "duck typing" make it extremely fast to develop. Python's GIL (Global Interpreter Lock) will become a performance bottleneck in a multi-threaded environment, but it can be alleviated by multi-process or using optimization libraries such as Numpy.
Example of usage
Basic usage of Golang
package main import "fmt" func main() { fmt.Println("Hello, Golang!") }
Golang's syntax is concise and clear, and the above code shows how to print a simple string. Golang's standard library is very powerful, with many commonly used functions built in, reducing dependence on third-party libraries.
Basic usage of Python
print("Hello, Python!")
Python's syntax is equally concise, but its dynamic typing system makes the code more flexible. Python's standard library is also very rich, especially in data processing and network programming.
Advanced Usage
Golang concurrent programming
package main import ( "fmt" "time" ) func says(s string) { for i := 0; i < 5; i { time.Sleep(100 * time.Millisecond) fmt.Println(s) } } func main() { go says("world") say("hello") }
Golang's concurrent programming is implemented through goroutine and channel. The above code shows how to execute two functions concurrently. It should be noted that the use of goroutine needs to be managed with caution to avoid resource leakage.
Python asynchronous programming
import asyncio async def says_after(delay, what): await asyncio.sleep(delay) print(what) async def main(): await asyncio.gather( say_after(1, 'hello'), say_after(2, 'world') ) asyncio.run(main())
Python's asynchronous programming is implemented through the asyncio library. The above code shows how to execute two functions asynchronously. Python's asynchronous programming performs well in I/O-intensive tasks, but it should be noted that the learning curve of asynchronous programming is steep.
Common Errors and Debugging Tips
Common errors for Golang developers include goroutine leaks and improper error handling. These problems can be detected and fixed by using tools such as go vet
and go test
.
Common errors for Python developers include indentation errors and type errors. These problems can be detected and fixed by using tools such as Pylint and PyCharm.
Performance optimization and best practices
Golang's performance optimization mainly focuses on concurrent programming and memory management. The performance bottlenecks of the program can be analyzed by using the pprof
tool and improve performance by reducing the number of goroutines and optimizing memory allocation.
Python's performance optimization mainly focuses on avoiding the impact of GIL and optimizing data structures. The execution speed of Python code can be improved by using tools such as Cython and Numba, and the efficiency of data processing can be improved by using optimization libraries such as Pandas and Numpy.
In terms of best practices, Golang developers should follow the principles of simplicity and efficiency, avoiding overly complex designs. Python developers should follow the "Pythonic" coding style to ensure the readability and maintainability of the code.
In-depth insights and suggestions
When choosing Golang or Python, you need to consider the specific needs of the project and the team's technical stack. Golang is suitable for scenarios where high performance and concurrent programming are required, such as backend services and microservice architectures. Python is suitable for scenarios that require rapid development and data processing, such as data science and machine learning projects.
In actual projects, I once encountered a web service project that requires high concurrency processing, and finally chose Golang because its concurrency model and efficient compilation speed greatly improved development efficiency. But in another data analysis project, I chose Python because of its rich library ecosystem and strong data processing capabilities that make the development process smoother.
It should be noted that both languages have their own advantages and disadvantages and applicable scenarios. When choosing, you should comprehensively consider the project's needs and the team's technical capabilities. At the same time, learning and mastering two languages can greatly expand your programming horizons and problem-solving abilities.
The above is the detailed content of Golang vs. Python: A Comparative Analysis. For more information, please follow other related articles on the PHP Chinese website!
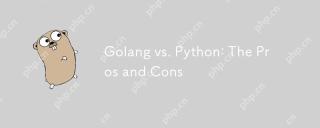
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
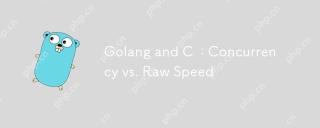
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
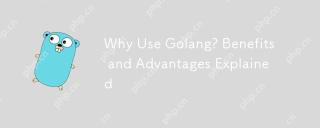
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
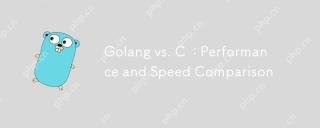
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
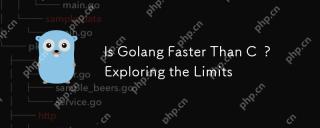
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
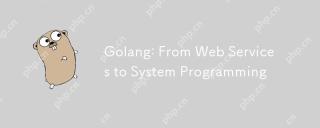
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
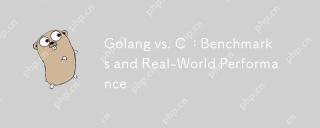
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
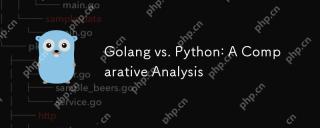
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
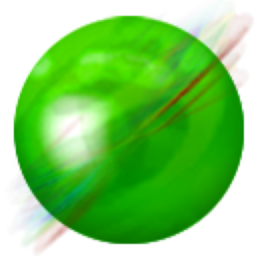
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
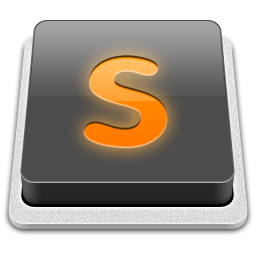
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
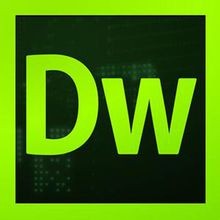
Dreamweaver CS6
Visual web development tools