Go 1.18 introduces generic functions, supports type parameterization, and enhances code reusability. The syntax of a generic function is func function name [type parameter] (input parameter type parameter) type parameter, and type parameterized types can be used as input and return types. For example, Min[T number] (a, b T) T, where T must be of numeric type, a and b are input parameters of type T, and the smaller number is returned. Generic functions greatly improve the reusability of code, allowing you to write general code that is suitable for various types.
Function Application of Generics in Go
Generics introduced in Go 1.18 and later allow functions to accept and return Type parameterized types. This greatly enhances code reusability and flexibility.
Syntax
The syntax of a generic function is as follows:
func myFunc[T any](input T) T { // ... }
Among them:
-
myFunc
is the function name. -
T any
is a type parameter. It can be of any type, including custom types. -
input
is an input parameter with a type parameterized type. -
T
is the return type with a type parameterized type.
Practical Case
Suppose we want to create a function to calculate the minimum of two numbers. Generic functions allow us to represent these two numbers with any number type without having to create multiple functions with specific type signatures.
We can write the following generic function:
func Min[T number](a, b T) T { if a < b { return a } return b }
Where:
-
T number
means that the type parameter T must be of numeric type (for example int, float64). -
a
andb
are input parameters of type T. -
if-else
statement compares two numbers and returns the smaller number.
We can use this function to calculate the minimum value of different types of numbers:
var a int8 = 10 var b int16 = 20 min := Min(a, b) // 类型推断为 int16 fmt.Println(min) // 输出:10
Conclusion
Go generics accept by allowing functions and return typed types, greatly improving code reusability and flexibility. By using generic functions, we can write generic code that works across a variety of types.
The above is the detailed content of Golang generic function application. For more information, please follow other related articles on the PHP Chinese website!
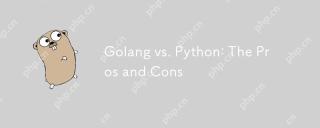
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
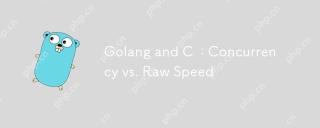
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
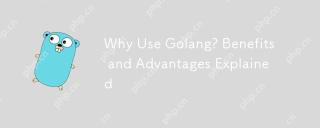
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
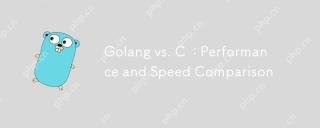
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
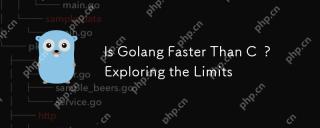
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
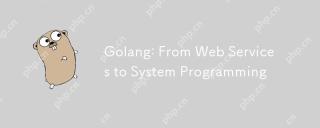
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
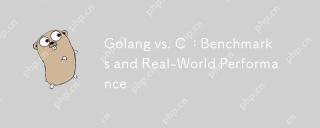
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
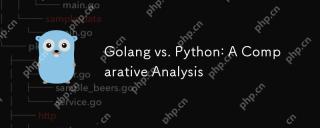
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
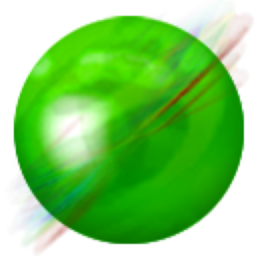
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
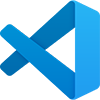
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version