Memory management of function return values follows value semantics. Functions pass parameters and return values by value. Changes to the copy do not affect the original variable. Pointer passing allows functions to directly modify the original variable. By applying these concepts, slice copying can be implemented efficiently, directly modifying elements in the target slice and avoiding the creation of new copies.
Memory management of function return values in Go
In Go, the memory management of function return values is affected by value semantics, which is an important A language feature that states that a variable always contains a copy of its value.
Value passing
Go functions pass parameters and return values through value passing. This means that a copy is created and passed, not a reference to the original value.
For example, the following code defines an Add
function that accepts two value type parameters and returns a value type result:
package main func Add(a, b int) int { return a + b } func main() { x := 1 y := 2 sum := Add(x, y) fmt.Println(sum) // 输出:3 }
In Add
Inside the function, copies of x
and y
are passed. Any changes made to these copies will not affect the value of the original variable. So when Add
returns, it returns a copy of the sum of x
and y
.
Pointer passing
You can avoid creating a copy during value passing by passing a value of pointer type. This allows the function to directly modify the value of the original variable.
For example, the following code defines an Inc
function that accepts a parameter of pointer type and increments it:
package main import "fmt" func Inc(ptr *int) { *ptr++ } func main() { x := 1 Inc(&x) fmt.Println(x) // 输出:2 }
In the Inc
function , the value of pointer ptr
is the address of original variable x
. Changes to *ptr
are applied directly to the original variable. Therefore, when Inc
returns, the value of x
has been incremented.
Practical Case: Implementing Slice Copy
We can apply these concepts to actual combat, such as implementing a function that copies a slice without creating a newly allocated copy:
package main func CopySlice(dst, src []int) { for i := range src { dst[i] = src[i] } } func main() { src := []int{1, 2, 3} dst := make([]int, len(src)) CopySlice(dst, src) fmt.Println(dst) // 输出:[]int{1, 2, 3} }
In the CopySlice
function, we use pointer arithmetic to directly modify the elements in the dst
slice, thereby avoiding creating a copy of the src
slice.
The above is the detailed content of How does Golang function return value manage memory?. For more information, please follow other related articles on the PHP Chinese website!
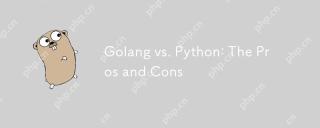
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
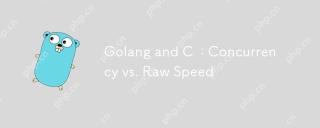
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
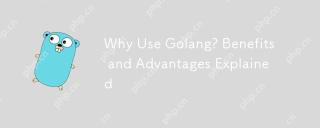
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
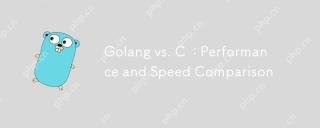
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
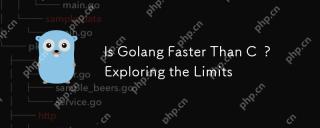
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
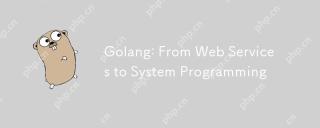
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
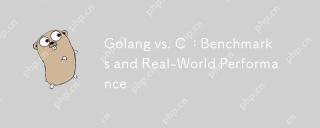
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
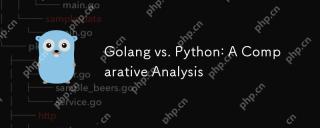
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
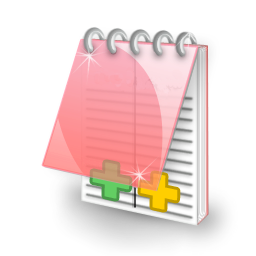
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
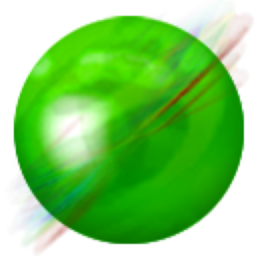
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
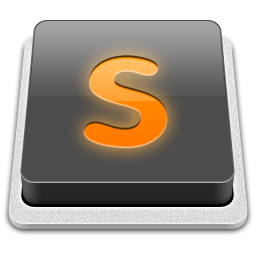
SublimeText3 Mac version
God-level code editing software (SublimeText3)