You can integrate database query results into HTML pages by following these steps: Establish a database connection. Execute the query and store the results. Loop through the query results and display them in HTML elements.
Integrate database queries with HTML using PHP
Integrating database query results and HTML pages allows you to create dynamic and interactive web applications. This article walks you through the steps to do this using PHP and provides a practical example to illustrate the process.
Step 1: Establish database connection
$servername = "localhost"; $username = "root"; $password = ""; $dbname = "myDB"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname);
Step 2: Execute query
To get data, use mysqli_query()
Function executes query.
$sql = "SELECT * FROM users"; $result = $conn->query($sql);
Step 3: Get the query results
To iterate over the query results, use the mysqli_fetch_assoc()
function. It returns an associative array containing key-value pairs.
while ($row = $result->fetch_assoc()) { echo "{$row['id']}: {$row['name']}<br>"; }
Practical case: display user list
The following example shows how to query the user list from the database into an HTML table:
index.php
<!DOCTYPE html> <html> <head> <title>用户列表</title> </head> <body> <h1 id="用户列表">用户列表</h1> <table> <thead> <tr> <th>ID</th> <th>姓名</th> </tr> </thead> <tbody> <?php include 'db_connect.php'; $sql = "SELECT * FROM users"; $result = $conn->query($sql); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { echo "<tr><td>{$row['id']}</td><td>{$row['name']}</td></tr>"; } } else { echo "<tr><td colspan='2'>没有用户</td></tr>"; } ?> </tbody> </table> </body> </html>
db_connect.php
// 数据库连接信息 $servername = "localhost"; $username = "root"; $password = ""; $dbname = "myDB"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname);
The above is the detailed content of Database query and HTML integration. For more information, please follow other related articles on the PHP Chinese website!

HTML, CSS and JavaScript are the core technologies for building modern web pages: 1. HTML defines the web page structure, 2. CSS is responsible for the appearance of the web page, 3. JavaScript provides web page dynamics and interactivity, and they work together to create a website with a good user experience.

The function of HTML is to define the structure and content of a web page, and its purpose is to provide a standardized way to display information. 1) HTML organizes various parts of the web page through tags and attributes, such as titles and paragraphs. 2) It supports the separation of content and performance and improves maintenance efficiency. 3) HTML is extensible, allowing custom tags to enhance SEO.

The future trends of HTML are semantics and web components, the future trends of CSS are CSS-in-JS and CSSHoudini, and the future trends of JavaScript are WebAssembly and Serverless. 1. HTML semantics improve accessibility and SEO effects, and Web components improve development efficiency, but attention should be paid to browser compatibility. 2. CSS-in-JS enhances style management flexibility but may increase file size. CSSHoudini allows direct operation of CSS rendering. 3.WebAssembly optimizes browser application performance but has a steep learning curve, and Serverless simplifies development but requires optimization of cold start problems.

The roles of HTML, CSS and JavaScript in web development are: 1. HTML defines the web page structure, 2. CSS controls the web page style, and 3. JavaScript adds dynamic behavior. Together, they build the framework, aesthetics and interactivity of modern websites.

The future of HTML is full of infinite possibilities. 1) New features and standards will include more semantic tags and the popularity of WebComponents. 2) The web design trend will continue to develop towards responsive and accessible design. 3) Performance optimization will improve the user experience through responsive image loading and lazy loading technologies.

The roles of HTML, CSS and JavaScript in web development are: HTML is responsible for content structure, CSS is responsible for style, and JavaScript is responsible for dynamic behavior. 1. HTML defines the web page structure and content through tags to ensure semantics. 2. CSS controls the web page style through selectors and attributes to make it beautiful and easy to read. 3. JavaScript controls web page behavior through scripts to achieve dynamic and interactive functions.

HTMLisnotaprogramminglanguage;itisamarkuplanguage.1)HTMLstructuresandformatswebcontentusingtags.2)ItworkswithCSSforstylingandJavaScriptforinteractivity,enhancingwebdevelopment.

HTML is the cornerstone of building web page structure. 1. HTML defines the content structure and semantics, and uses, etc. tags. 2. Provide semantic markers, such as, etc., to improve SEO effect. 3. To realize user interaction through tags, pay attention to form verification. 4. Use advanced elements such as, combined with JavaScript to achieve dynamic effects. 5. Common errors include unclosed labels and unquoted attribute values, and verification tools are required. 6. Optimization strategies include reducing HTTP requests, compressing HTML, using semantic tags, etc.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
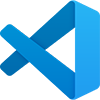
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.