The roles of HTML, CSS and JavaScript in web development are: 1. HTML defines the web page structure, 2. CSS controls the web page style, and 3. JavaScript adds dynamic behavior. Together, they build the framework, aesthetics and interactivity of modern websites.
introduction
In the world of web development, HTML, CSS and JavaScript have jointly built the modern website we see. They are like the three pillars of architecture: HTML provides structure, like the framework of a house; CSS is responsible for the style, making the house beautiful; JavaScript gives behavior, making the house smart. This article will take you into the depth of the functions and collaboration of these three, helping you understand how to use them to build a dynamic and beautiful website. You will learn a comprehensive range of knowledge from basic concepts to practical applications, and learn how to use these techniques efficiently in your project.
Review of basic knowledge
HTML (HyperText Markup Language) is the cornerstone of building web pages, which uses tag tags to describe the structure of a web page. Imagine HTML is like a woodworker that frames your web pages, and these tags are wood and nails. CSS (Cascading Style Sheets) is responsible for beautifying this framework, which defines the appearance and layout of a web page, including everything from colors, fonts to animation effects. JavaScript is the soul of web pages, which makes web pages no longer static images, but a dynamic entity that can interact with users.
Core concept or function analysis
HTML: The Art of Building Structure
The role of HTML is to define the structure and content of a web page. Through a series of tags, HTML can organize elements such as text, images, and links to form a logical structure. Its advantages are its simplicity and easy to learn, and good readability and maintainability.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>My First Webpage</title> </head> <body> <h1 id="Welcome-to-My-Website">Welcome to My Website</h1> <p>This is a paragraph of text.</p> <img src="/static/imghwm/default1.png" data-src="image.jpg" class="lazy" alt="An image"> <a href="https://www.example.com">Visit Example.com</a> </body> </html>
HTML works by parsing these tags to build a DOM (Document Object Model), which is a tree structure that represents the hierarchical relationship of a web page. Understanding DOM is crucial for subsequent CSS and JavaScript operations.
CSS: The color that gives life to web pages
The function of CSS is to control the appearance and layout of a web page. It defines the style of elements through selectors and attributes, making the web page not only clear structure, but also beautiful and elegant. The advantage of CSS is that it can be independent of HTML content, separate styles and structures, and improves the maintainability and reusability of the code.
body { font-family: Arial, sans-serif; background-color: #f0f0f0; } h1 { color: #333; text-align: center; } p { color: #666; line-height: 1.5; } img { max-width: 100%; height: auto; } a { color: #0066cc; text-decoration: none; } a:hover { text-decoration: underline; }
CSS works by applying styles to the DOM through the browser's rendering engine. The selector matches elements in the DOM and applies the corresponding style attributes. Understanding the cascading and inheritance of CSS is essential for efficient use of CSS.
JavaScript: Magic to make web pages move
The role of JavaScript is to add dynamic behavior to web pages. It can respond to user actions, modify the DOM, process data, and even communicate with the server. The advantage of JavaScript is that it can make web pages more interactive and intelligent and improve user experience.
document.addEventListener('DOMContentLoaded', function() { const button = document.querySelector('button'); button.addEventListener('click', function() { alert('Button clicked!'); }); const input = document.querySelector('input'); input.addEventListener('input', function() { console.log('Input value:', input.value); }); });
JavaScript works by responding to user operations and system events through an event-driven model. It can directly manipulate the DOM and modify the attributes and contents of elements. Understanding event loops and asynchronous programming is essential for writing efficient JavaScript code.
Example of usage
Basic usage of HTML
The basic usage of HTML is to define the structure of a web page through tags. Here is a simple HTML page example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>My First Webpage</title> </head> <body> <h1 id="Welcome-to-My-Website">Welcome to My Website</h1> <p>This is a paragraph of text.</p> <img src="/static/imghwm/default1.png" data-src="image.jpg" class="lazy" alt="An image"> <a href="https://www.example.com">Visit Example.com</a> </body> </html>
Each line of code has its own specific function: <h1></h1>
defines a title, <p></p>
defines a paragraph, <img alt="HTML: The Structure, CSS: The Style, JavaScript: The Behavior" >
inserts an image, and <a></a>
creates a link.
Advanced usage of CSS
Advanced usage of CSS includes using Flexbox or Grid to create complex layouts, and using animations and transition effects to enhance the user experience. Here is an example using Flexbox:
.container { display: flex; justify-content: space-between; align-items: center; padding: 20px; } .item { flex: 1; margin: 10px; padding: 20px; background-color: #eee; border: 1px solid #ddd; transition: all 0.3s ease; } .item:hover { transform: scale(1.05); box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); }
This example shows how to use Flexbox to create a responsive layout and enhance user interaction through transition effects and transformations.
Common Errors and Debugging Tips for JavaScript
Common errors in JavaScript development include syntax errors, type errors, and logical errors. Here are some common errors and their debugging tips:
- Syntax error : Use the browser's developer tools to view the console output and find the specific location of the error.
- Type error : Use
typeof
operator to check the variable type to ensure that the operators and methods are used correctly. - Logical error : Use
console.log
ordebugger
statement to track the code execution process and find out the logical problem.
// Syntax error example function greet(name) { console.log('Hello, ' name!); // Error: There is an exclamation mark} // Type error example let number = '123'; // String type console.log(number 10); // Output '12310' instead of 133 // Logical error example function calculateTotal(price, taxRate) { let total = price taxRate; // Error: price * taxRate should be used return total; }
Performance optimization and best practices
In practical applications, optimizing HTML, CSS and JavaScript code can significantly improve the performance and user experience of web pages. Here are some recommendations for optimization and best practices:
- HTML optimization : Use semantic tags to reduce nesting levels and compress HTML code.
- CSS optimization : Use efficient selectors to avoid overuse!important, compress CSS code.
- JavaScript optimization : Reduce DOM operations, use event delegates, asynchronous loading of scripts, and compress JavaScript code.
Here is an example of comparing the performance differences between different methods:
// Unoptimized version function slowFunction() { let result = 0; for (let i = 0; i < 1000000; i ) { result = i; } return result; } // Optimized version function fastFunction() { return (1000000 * 999999) / 2; // Use mathematical formulas to calculate directly} console.time('slowFunction'); slowFunction(); console.timeEnd('slowFunction'); console.time('fastFunction'); fastFunction(); console.timeEnd('fastFunction');
This example shows how to use mathematical formulas to significantly reduce calculation time.
When it comes to programming habits and best practices, it is crucial to keep the code readable and maintained. Using meaningful variable names and function names, adding appropriate comments, and following code style guides are key to improving code quality.
By deeply understanding the capabilities and collaboration of HTML, CSS and JavaScript, you will be able to build more efficient, beautiful and interactive web pages. Hope this article provides valuable guidance and inspiration for your web development journey.
The above is the detailed content of HTML: The Structure, CSS: The Style, JavaScript: The Behavior. For more information, please follow other related articles on the PHP Chinese website!

The roles of HTML, CSS and JavaScript in web development are: 1. HTML is used to build web page structure; 2. CSS is used to beautify the appearance of web pages; 3. JavaScript is used to achieve dynamic interaction. Through tags, styles and scripts, these three together build the core functions of modern web pages.

Setting the lang attributes of a tag is a key step in optimizing web accessibility and SEO. 1) Set the lang attribute in the tag, such as. 2) In multilingual content, set lang attributes for different language parts, such as. 3) Use language codes that comply with ISO639-1 standards, such as "en", "fr", "zh", etc. Correctly setting the lang attribute can improve the accessibility of web pages and search engine rankings.

HTMLattributesareessentialforenhancingwebelements'functionalityandappearance.Theyaddinformationtodefinebehavior,appearance,andinteraction,makingwebsitesinteractive,responsive,andvisuallyappealing.Attributeslikesrc,href,class,type,anddisabledtransform

TocreatealistinHTML,useforunorderedlistsandfororderedlists:1)Forunorderedlists,wrapitemsinanduseforeachitem,renderingasabulletedlist.2)Fororderedlists,useandfornumberedlists,customizablewiththetypeattributefordifferentnumberingstyles.

HTML is used to build websites with clear structure. 1) Use tags such as, and define the website structure. 2) Examples show the structure of blogs and e-commerce websites. 3) Avoid common mistakes such as incorrect label nesting. 4) Optimize performance by reducing HTTP requests and using semantic tags.

ToinsertanimageintoanHTMLpage,usethetagwithsrcandaltattributes.1)UsealttextforaccessibilityandSEO.2)Implementsrcsetforresponsiveimages.3)Applylazyloadingwithloading="lazy"tooptimizeperformance.4)OptimizeimagesusingtoolslikeImageOptimtoreduc

The core purpose of HTML is to enable the browser to understand and display web content. 1. HTML defines the web page structure and content through tags, such as, to, etc. 2. HTML5 enhances multimedia support and introduces and tags. 3.HTML provides form elements to support user interaction. 4. Optimizing HTML code can improve web page performance, such as reducing HTTP requests and compressing HTML.

HTMLtagsareessentialforwebdevelopmentastheystructureandenhancewebpages.1)Theydefinelayout,semantics,andinteractivity.2)SemantictagsimproveaccessibilityandSEO.3)Properuseoftagscanoptimizeperformanceandensurecross-browsercompatibility.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
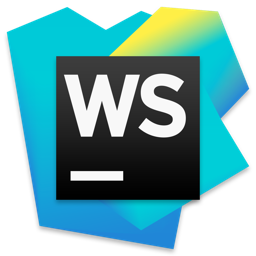
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
