Python Lambda expressions: Make code concise and clear
Introduction to Lambda expressions
Lambda expression is an anonymous function in python. It can define a function without defining a function name. The syntax is:
lambda arguments : expression
Among them, arguments are the parameters of the function, and expression is the expression of the function. For example, the following code defines a lambda expression that adds two numbers and returns the result:
lambda x, y: x + y
Advantages of Lambda expressions
The main advantages of Lambda expressions are simplicity and anonymity. It lets you define a function without defining its name, which is useful for some simple tasks. For example, the following code uses a lambda expression to square each element in a list:
numbers = [1, 2, 3, 4, 5] squared_numbers = map(lambda x: x ** 2, numbers) print(list(squared_numbers))
The output result is:
numbers = [1, 2, 3, 4, 5] even_numbers = filter(lambda x: x % 2 == 0, numbers) print(list(even_numbers))
The output result is:
[2, 4]
- List mapping: Each element in a list can be easily mapped to a new value using lambda expressions. For example, the following code uses a lambda expression to square each element in the list:
numbers = [1, 2, 3, 4, 5] squared_numbers = map(lambda x: x ** 2, numbers) print(list(squared_numbers))
The output result is:
strings = ["apple", "banana", "cherry", "durian", "elderberry"] sorted_strings = sorted(strings, key=lambda x: len(x)) print(sorted_strings)
The output result is:
["apple", "cherry", "banana", "elderberry", "durian"]
Conclusion
Lambda expressions are a powerful tool that allows you to write cleaner, more readable, and more efficient code. It can be used in a variety of scenarios, including operations such as list filtering, mapping, and sorting. If you want to write cleaner and more efficient code, lambda expressions are a tool worth mastering.
The above is the detailed content of Python Lambda expressions: Make code concise and clear. For more information, please follow other related articles on the PHP Chinese website!
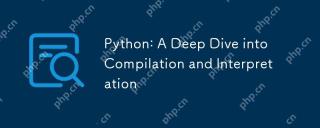
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
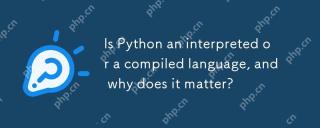
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
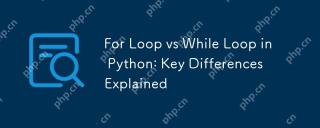
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
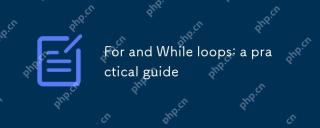
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond
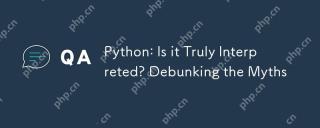
Pythonisnotpurelyinterpreted;itusesahybridapproachofbytecodecompilationandruntimeinterpretation.1)Pythoncompilessourcecodeintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).2)Thisprocessallowsforrapiddevelopmentbutcanimpactperformance,req
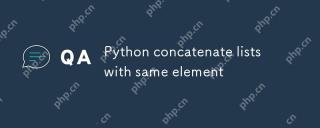
ToconcatenatelistsinPythonwiththesameelements,use:1)the operatortokeepduplicates,2)asettoremoveduplicates,or3)listcomprehensionforcontroloverduplicates,eachmethodhasdifferentperformanceandorderimplications.
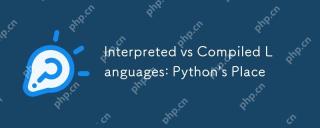
Pythonisaninterpretedlanguage,offeringeaseofuseandflexibilitybutfacingperformancelimitationsincriticalapplications.1)InterpretedlanguageslikePythonexecuteline-by-line,allowingimmediatefeedbackandrapidprototyping.2)CompiledlanguageslikeC/C transformt
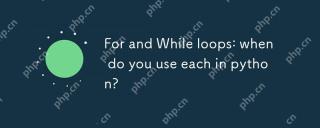
Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
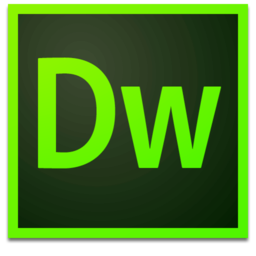
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!
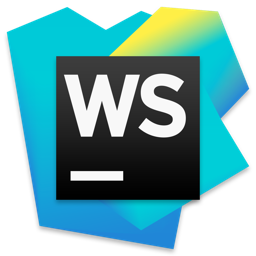
WebStorm Mac version
Useful JavaScript development tools
