Python is an interpreted language, offering ease of use and flexibility but facing performance limitations in critical applications. 1) Interpreted languages like Python execute line-by-line, allowing immediate feedback and rapid prototyping. 2) Compiled languages like C/C transform to machine code for faster execution but require compilation. 3) Python uses Just-In-Time (JIT) compilation tools like PyPy to enhance performance. 4) The Global Interpreter Lock (GIL) in Python can limit multi-threading efficiency, suggesting alternatives like multiprocessing or different Python implementations.
When diving into the world of programming, one of the first distinctions you encounter is between interpreted and compiled languages. Python, being an interpreted language, often sparks curiosity about its performance and efficiency compared to compiled languages. Let's explore this fascinating topic and see where Python fits in this spectrum.
Python's role as an interpreted language brings both advantages and challenges. As someone who's spent countless hours coding in Python, I've come to appreciate its flexibility and ease of use, but I've also bumped into its limitations, particularly when it comes to performance-critical applications.
Interpreted languages like Python are executed line by line by an interpreter at runtime. This means you can write a script, run it, see the results immediately, and then tweak it without the need for a separate compilation step. It's like having a conversation with your code—immediate feedback, which is incredibly helpful for learning and rapid prototyping.
# A simple Python script to demonstrate interpreted nature print("Hello, World!") for i in range(5): print(f"Counting: {i}")
This code runs directly, and you can see the output without any compilation. It's this simplicity that makes Python a favorite for beginners and experts alike.
On the other hand, compiled languages like C or C are transformed into machine code before execution. This process can take time, but the resulting executable runs much faster. Here's a quick example in C:
#include <stdio.h> int main() { printf("Hello, World!\n"); for (int i = 0; i < 5; i ) { printf("Counting: %d\n", i); } return 0; }
To run this, you'd need to compile it first, which might look something like this on a Unix-like system:
gcc hello.c -o hello ./hello
The compiled version will generally run faster than the Python script, but at the cost of that immediate feedback loop.
Now, where does Python stand in this debate? Python's interpreted nature makes it incredibly versatile and accessible. It's perfect for scripting, data analysis, machine learning, and web development. However, when it comes to performance-intensive tasks, Python can sometimes fall short.
One of the ways Python tries to bridge this gap is through Just-In-Time (JIT) compilation. Tools like PyPy use JIT compilation to improve performance by compiling Python code into machine code at runtime. Here's a quick example of how you might use PyPy:
# Install PyPy pip install pypy # Run your Python script with PyPy pypy your_script.py
While this can significantly boost performance, it's not a silver bullet. You might still encounter situations where a compiled language is more suitable.
From my experience, the choice between interpreted and compiled languages often boils down to the specific needs of your project. If you're building a web application or a data analysis tool, Python's ease of use and rich ecosystem of libraries can be a huge advantage. But if you're developing a high-performance game engine or a real-time system, you might want to consider a compiled language.
One of the pitfalls I've encountered with Python is its Global Interpreter Lock (GIL), which can limit true parallelism in multi-threaded applications. This is something to keep in mind if you're working on CPU-bound tasks. Here's a simple example to illustrate the GIL's impact:
import threading import time def cpu_bound_task(n): count = 0 for i in range(n): count = i return count def main(): start = time.time() threads = [] for _ in range(4): t = threading.Thread(target=cpu_bound_task, args=(10**8,)) threads.append(t) t.start() for t in threads: t.join() end = time.time() print(f"Time taken: {end - start} seconds") if __name__ == "__main__": main()
Running this script, you'll notice that the performance doesn't scale linearly with the number of threads due to the GIL. This is a classic example of where Python's interpreted nature can be a limitation.
To mitigate such issues, you might consider using multiprocessing instead of threading, or even looking into alternative Python implementations like Jython or IronPython, which don't have a GIL.
In conclusion, Python's place as an interpreted language is both its strength and its challenge. It offers unparalleled ease of use and flexibility, making it a go-to choice for many applications. However, understanding its limitations, particularly in performance-critical scenarios, is crucial for making informed decisions in your programming journey. Whether you're a beginner or a seasoned developer, embracing Python's interpreted nature while being aware of its constraints can lead to more effective and efficient coding practices.
The above is the detailed content of Interpreted vs Compiled Languages: Python's Place. For more information, please follow other related articles on the PHP Chinese website!
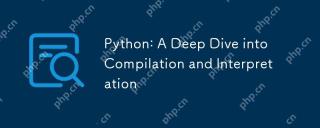
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
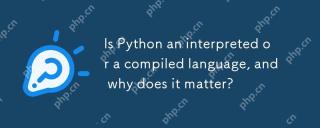
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
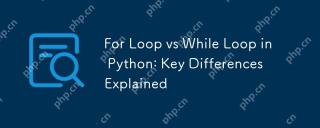
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
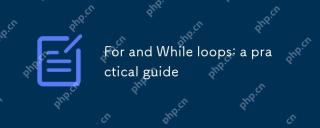
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond
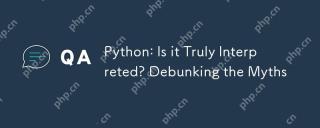
Pythonisnotpurelyinterpreted;itusesahybridapproachofbytecodecompilationandruntimeinterpretation.1)Pythoncompilessourcecodeintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).2)Thisprocessallowsforrapiddevelopmentbutcanimpactperformance,req
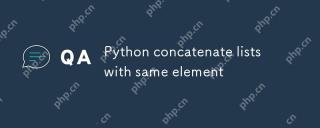
ToconcatenatelistsinPythonwiththesameelements,use:1)the operatortokeepduplicates,2)asettoremoveduplicates,or3)listcomprehensionforcontroloverduplicates,eachmethodhasdifferentperformanceandorderimplications.
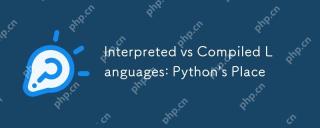
Pythonisaninterpretedlanguage,offeringeaseofuseandflexibilitybutfacingperformancelimitationsincriticalapplications.1)InterpretedlanguageslikePythonexecuteline-by-line,allowingimmediatefeedbackandrapidprototyping.2)CompiledlanguageslikeC/C transformt
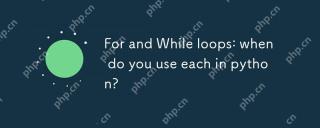
Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
