Python: A Deep Dive into Compilation and Interpretation
Python uses a hybrid model of compilation and interpretation: 1) The Python interpreter compiles source code into platform-independent bytecode. 2) The Python Virtual Machine (PVM) then executes this bytecode, balancing ease of use with performance.
Diving into Python's world, the question often arises: how does Python handle code execution? Is it compiled or interpreted? The answer isn't as straightforward as one might hope. Python employs a unique approach that blends both compilation and interpretation. Let's explore this fascinating journey from source code to execution.
Python's execution model is a hybrid of compilation and interpretation, often referred to as "compile to bytecode and interpret." When you run a Python script, the Python interpreter first compiles the source code into bytecode, which is a platform-independent, intermediate representation of the code. This bytecode is then executed by the Python Virtual Machine (PVM).
Let's break down this process with some code and insights.
When you write a Python script, say example.py
, and run it, here's what happens behind the scenes:
# example.py def greet(name): return f"Hello, {name}!" print(greet("World"))
The Python interpreter (python
or python3
) reads the source code and compiles it into bytecode. You can see this bytecode using the dis
module:
import dis def greet(name): return f"Hello, {name}!" dis.dis(greet)
This will output the bytecode, which looks something like this:
2 0 LOAD_CONST 1 ('Hello, {}!') 2 LOAD_FAST 0 (name) 4 FORMAT_VALUE 0 6 BUILD_STRING 2 8 RETURN_VALUE
This bytecode is what the PVM executes. The compilation to bytecode happens on-the-fly, and the resulting bytecode is stored in .pyc
files for future runs, speeding up subsequent executions.
Now, let's delve deeper into the advantages and potential pitfalls of this approach.
Advantages:
- Portability: Bytecode is platform-independent, allowing Python code to run on any system with a Python interpreter.
- Performance: Compiling to bytecode once and reusing it can significantly speed up execution, especially for larger scripts.
- Dynamic Typing: Python's dynamic nature is preserved, allowing for flexible and expressive code.
Potential Pitfalls:
- Startup Time: The initial compilation step can introduce a slight delay, especially for small scripts.
- Debugging Complexity: Debugging at the bytecode level can be challenging, requiring specialized tools and knowledge.
- Memory Usage: The PVM and bytecode can consume more memory compared to purely compiled languages.
In my experience, the hybrid model strikes a great balance between ease of use and performance. I've worked on projects where the initial compilation time was negligible compared to the overall execution time, making Python a great choice for rapid prototyping and development.
However, for applications where every millisecond counts, such as high-frequency trading systems, the initial compilation delay and memory usage might be a concern. In such cases, tools like Cython or Numba, which compile Python to native code, can be valuable.
To optimize Python's performance, consider the following:
-
Use
.pyc
files: Ensure that.pyc
files are generated and used to speed up subsequent runs. -
Profile your code: Use tools like
cProfile
to identify bottlenecks and optimize them. - Leverage libraries: For computationally intensive tasks, use libraries like NumPy or Pandas, which are optimized for performance.
Here's an example of how you can use cProfile
to identify performance bottlenecks:
import cProfile def slow_function(): result = 0 for i in range(1000000): result = i return result cProfile.run('slow_function()')
This will output profiling information, helping you pinpoint where your code spends most of its time.
In conclusion, Python's approach to compilation and interpretation is a testament to its design philosophy of simplicity and efficiency. By understanding this process, you can better appreciate Python's strengths and optimize your code to leverage its full potential. Whether you're a beginner or an experienced developer, this knowledge can help you write more efficient and effective Python code.
The above is the detailed content of Python: A Deep Dive into Compilation and Interpretation. For more information, please follow other related articles on the PHP Chinese website!
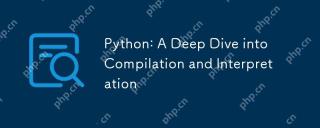
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
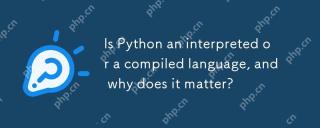
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
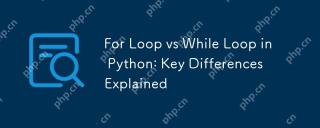
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
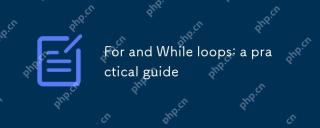
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond
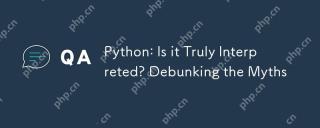
Pythonisnotpurelyinterpreted;itusesahybridapproachofbytecodecompilationandruntimeinterpretation.1)Pythoncompilessourcecodeintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).2)Thisprocessallowsforrapiddevelopmentbutcanimpactperformance,req
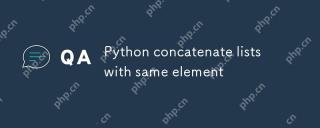
ToconcatenatelistsinPythonwiththesameelements,use:1)the operatortokeepduplicates,2)asettoremoveduplicates,or3)listcomprehensionforcontroloverduplicates,eachmethodhasdifferentperformanceandorderimplications.
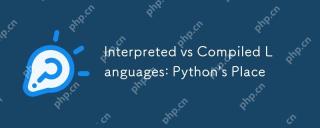
Pythonisaninterpretedlanguage,offeringeaseofuseandflexibilitybutfacingperformancelimitationsincriticalapplications.1)InterpretedlanguageslikePythonexecuteline-by-line,allowingimmediatefeedbackandrapidprototyping.2)CompiledlanguageslikeC/C transformt
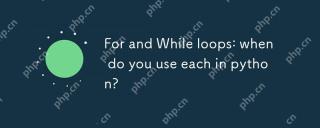
Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
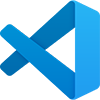
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
