Use for loops when the number of iterations is known in advance, and while loops when iterations depend on a condition. 1) For loops are ideal for sequences like lists or ranges. 2) While loops suit scenarios where the loop continues until a specific condition is met, useful for user inputs or algorithms reaching a state.
When it comes to choosing between for
and while
loops in Python, the decision often hinges on the nature of the iteration you're performing. I've seen countless scenarios where understanding this choice can make your code more efficient and readable.
When do you use each in Python?
For loops are your go-to when you know the number of iterations in advance. They're perfect for iterating over sequences like lists, tuples, or strings. I've used them extensively when I need to process every item in a collection, or when I'm working with a range of numbers.
While loops, on the other hand, are ideal when you're not sure how many times you'll need to loop, or when you want to continue looping based on a condition. I've found them invaluable for situations like reading input from a user until a specific condition is met, or when implementing algorithms that need to run until a certain state is achieved.
Let's dive deeper into these loops and explore their nuances.
When I first started programming in Python, I was fascinated by how for
loops could elegantly handle iteration over sequences. Here's a simple example where I used a for
loop to iterate over a list of fruits:
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(f"I love {fruit}!")
This approach is clean and straightforward. But what if you need to iterate a specific number of times? That's where range()
comes in handy:
for i in range(5): print(f"Counting: {i}")
I've often used for
loops in more complex scenarios, like processing data from a file or iterating over a dictionary. Here's an example where I used a for
loop to iterate over a dictionary's keys and values:
person = {"name": "Alice", "age": 30, "city": "New York"} for key, value in person.items(): print(f"{key}: {value}")
Now, let's shift gears to while
loops. I've used while
loops in situations where I needed to keep a program running until a certain condition was met. For instance, when creating a simple guessing game:
import random target_number = random.randint(1, 100) guess = 0 while guess != target_number: guess = int(input("Guess a number between 1 and 100: ")) if guess < target_number: print("Too low!") elif guess > target_number: print("Too high!") print(f"Congratulations! You guessed the number {target_number} correctly!")
This example showcases how while
loops are perfect for scenarios where you don't know how many iterations will be needed.
I've also used while
loops for more complex scenarios, like implementing algorithms that need to run until a certain state is reached. Here's an example of a simple algorithm to find the square root of a number using the Newton-Raphson method:
def square_root(n, tolerance=1e-6): guess = n / 2 while abs(guess * guess - n) > tolerance: guess = (guess n / guess) / 2 return guess print(square_root(16)) # Output: 4.0
When choosing between for
and while
loops, consider the following insights:
Efficiency:
For
loops are generally more efficient when you know the number of iterations in advance. They're optimized for sequences and can be faster thanwhile
loops in these cases.Readability:
For
loops can make your code more readable when iterating over sequences. However,while
loops can be clearer when dealing with conditions that aren't tied to a specific number of iterations.Control Flow:
While
loops give you more control over the flow of your program. You can break out of the loop at any point based on a condition, which can be useful in more complex scenarios.Potential Pitfalls: Be cautious with
while
loops to avoid infinite loops. Always ensure there's a way for the loop to terminate. Withfor
loops, you're less likely to run into this issue since they naturally terminate after iterating over the sequence.
In my experience, mixing both types of loops can sometimes lead to more elegant solutions. For instance, I've used a for
loop inside a while
loop to process a list of items until a certain condition is met:
numbers = [1, 2, 3, 4, 5] target_sum = 10 current_sum = 0 index = 0 while current_sum < target_sum and index < len(numbers): current_sum = numbers[index] index = 1 print(f"Sum: {current_sum}, Index: {index}")
This approach combines the benefits of both loop types, allowing you to iterate over a sequence while maintaining control over when to stop based on a condition.
In conclusion, understanding when to use for
and while
loops in Python is crucial for writing efficient and readable code. By considering the nature of your iteration and the specific requirements of your task, you can choose the right loop for the job. Remember, the best programmers are those who can adapt their tools to fit the problem at hand, and mastering these loops is a step in that direction.
The above is the detailed content of For and While loops: when do you use each in python?. For more information, please follow other related articles on the PHP Chinese website!
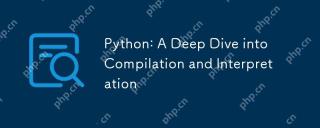
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
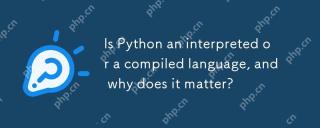
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
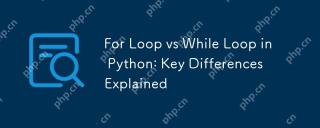
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
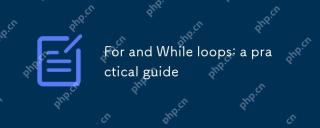
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond
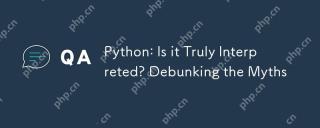
Pythonisnotpurelyinterpreted;itusesahybridapproachofbytecodecompilationandruntimeinterpretation.1)Pythoncompilessourcecodeintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).2)Thisprocessallowsforrapiddevelopmentbutcanimpactperformance,req
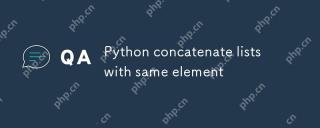
ToconcatenatelistsinPythonwiththesameelements,use:1)the operatortokeepduplicates,2)asettoremoveduplicates,or3)listcomprehensionforcontroloverduplicates,eachmethodhasdifferentperformanceandorderimplications.
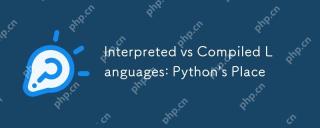
Pythonisaninterpretedlanguage,offeringeaseofuseandflexibilitybutfacingperformancelimitationsincriticalapplications.1)InterpretedlanguageslikePythonexecuteline-by-line,allowingimmediatefeedbackandrapidprototyping.2)CompiledlanguageslikeC/C transformt
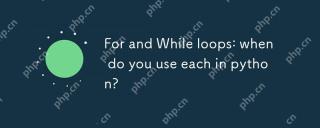
Useforloopswhenthenumberofiterationsisknowninadvance,andwhileloopswheniterationsdependonacondition.1)Forloopsareidealforsequenceslikelistsorranges.2)Whileloopssuitscenarioswheretheloopcontinuesuntilaspecificconditionismet,usefulforuserinputsoralgorit


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
