Learn numpy slicing techniques to simplify large data processing
Master the Numpy slicing operation method and easily process large-scale data. Specific code examples are required
Summary:
Use appropriate tools when processing large-scale data Very important. Numpy is a commonly used library in Python that provides high-performance numerical calculation tools. This article will introduce Numpy's slicing operation method, and use code examples to demonstrate how to easily operate and extract data when processing large-scale data.
- Introduction
Numpy is a commonly used numerical calculation library in Python, providing efficient data processing tools. The slicing operation is a very powerful function in Numpy, which can be used to quickly access and operate the elements of an array. The slicing operation can perform flexible operations on one-dimensional, two-dimensional, and multi-dimensional arrays, saving the process of writing loops and improving the operation speed. - One-dimensional array slicing
First, let’s look at the slicing operation method of one-dimensional array. Suppose we have a one-dimensional array a containing 10 elements:
import numpy as np a = np.array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
We can use colon: to specify the range of the slice. The sample code is as follows:
# 切片操作 b = a[2:6] # 从下标2到下标5的元素 print(b) # 输出:[2 3 4 5] c = a[:4] # 从开头到下标3的元素 print(c) # 输出:[0 1 2 3] d = a[6:] # 从下标6到末尾的元素 print(d) # 输出:[6 7 8 9] e = a[::3] # 每隔2个元素取一个 print(e) # 输出:[0 3 6 9]
- Two-dimensional array slicing
Next, let’s look at the slicing operation method of the two-dimensional array. Suppose we have a 2x3 two-dimensional array b:
b = np.array([[0, 1, 2], [3, 4, 5]])
We can specify the range of the slice by using commas. The sample code is as follows:
# 切片操作 c = b[0] # 提取第0行的元素 print(c) # 输出:[0 1 2] d = b[:, 1] # 提取所有行的第1列元素 print(d) # 输出:[1 4] e = b[:2, 1:] # 提取前两行以及第二列之后的元素 print(e) # 输出:[[1 2] # [4 5]]
- Multidimensional array slicing
Slicing operations are also very convenient when processing multidimensional arrays. Suppose we have a 3x3x3 three-dimensional array c:
c = np.array([[[0, 1, 2], [3, 4, 5], [6, 7, 8]], [[9, 10, 11], [12, 13, 14], [15, 16, 17]], [[18, 19, 20], [21, 22, 23], [24, 25, 26]]])
We can specify the range of the slice by increasing the number of commas. The sample code is as follows:
# 切片操作 d = c[0] # 提取第0个二维数组 print(d) # 输出:[[0 1 2] # [3 4 5] # [6 7 8]] e = c[:, 1, :] # 提取所有二维数组的第1行的元素 print(e) # 输出:[[ 3 4 5] # [12 13 14] # [21 22 23]] f = c[:, :, ::2] # 提取所有二维数组的每隔一个元素的列 print(f) # 输出:[[[ 0 2] # [ 3 5] # [ 6 8]] # [[ 9 11] # [12 14] # [15 17]] # [[18 20] # [21 23] # [24 26]]]
- Summary
This article introduces Numpy's slicing operation method, and illustrates through specific code examples how to use slicing operations to easily process large-scale data. Slicing operations can perform flexible operations on one-dimensional, two-dimensional, and multi-dimensional arrays, which can greatly improve the efficiency of data processing and the readability of code. Once you master the Numpy slicing operation method, it will become easier to process large-scale data.
References:
- Travis E, Oliphant. (2006). A guide to NumPy. USA: Trelgol Publishing
- https://numpy .org/doc/stable/reference/
- https://numpy.org/doc/stable/user/quickstart.html
Code example:
import numpy as np # 一维数组切片 a = np.array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]) b = a[2:6] c = a[:4] d = a[6:] e = a[::3] # 二维数组切片 b = np.array([[0, 1, 2], [3, 4, 5]]) c = b[0] d = b[:, 1] e = b[:2, 1:] # 多维数组切片 c = np.array([[[0, 1, 2], [3, 4, 5], [6, 7, 8]], [[9, 10, 11], [12, 13, 14], [15, 16, 17]], [[18, 19, 20], [21, 22, 23], [24, 25, 26]]]) d = c[0] e = c[:, 1, :] f = c[:, :, ::2]
The above is the detailed content of Learn numpy slicing techniques to simplify large data processing. For more information, please follow other related articles on the PHP Chinese website!

HTML, CSS and JavaScript are the core technologies for building modern web pages: 1. HTML defines the web page structure, 2. CSS is responsible for the appearance of the web page, 3. JavaScript provides web page dynamics and interactivity, and they work together to create a website with a good user experience.

The function of HTML is to define the structure and content of a web page, and its purpose is to provide a standardized way to display information. 1) HTML organizes various parts of the web page through tags and attributes, such as titles and paragraphs. 2) It supports the separation of content and performance and improves maintenance efficiency. 3) HTML is extensible, allowing custom tags to enhance SEO.

The future trends of HTML are semantics and web components, the future trends of CSS are CSS-in-JS and CSSHoudini, and the future trends of JavaScript are WebAssembly and Serverless. 1. HTML semantics improve accessibility and SEO effects, and Web components improve development efficiency, but attention should be paid to browser compatibility. 2. CSS-in-JS enhances style management flexibility but may increase file size. CSSHoudini allows direct operation of CSS rendering. 3.WebAssembly optimizes browser application performance but has a steep learning curve, and Serverless simplifies development but requires optimization of cold start problems.

The roles of HTML, CSS and JavaScript in web development are: 1. HTML defines the web page structure, 2. CSS controls the web page style, and 3. JavaScript adds dynamic behavior. Together, they build the framework, aesthetics and interactivity of modern websites.

The future of HTML is full of infinite possibilities. 1) New features and standards will include more semantic tags and the popularity of WebComponents. 2) The web design trend will continue to develop towards responsive and accessible design. 3) Performance optimization will improve the user experience through responsive image loading and lazy loading technologies.

The roles of HTML, CSS and JavaScript in web development are: HTML is responsible for content structure, CSS is responsible for style, and JavaScript is responsible for dynamic behavior. 1. HTML defines the web page structure and content through tags to ensure semantics. 2. CSS controls the web page style through selectors and attributes to make it beautiful and easy to read. 3. JavaScript controls web page behavior through scripts to achieve dynamic and interactive functions.

HTMLisnotaprogramminglanguage;itisamarkuplanguage.1)HTMLstructuresandformatswebcontentusingtags.2)ItworkswithCSSforstylingandJavaScriptforinteractivity,enhancingwebdevelopment.

HTML is the cornerstone of building web page structure. 1. HTML defines the content structure and semantics, and uses, etc. tags. 2. Provide semantic markers, such as, etc., to improve SEO effect. 3. To realize user interaction through tags, pay attention to form verification. 4. Use advanced elements such as, combined with JavaScript to achieve dynamic effects. 5. Common errors include unclosed labels and unquoted attribute values, and verification tools are required. 6. Optimization strategies include reducing HTTP requests, compressing HTML, using semantic tags, etc.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
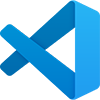
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!