


Pandas sorting skills revealed: How to sort according to specific conditions requires specific code examples
In the process of data processing and analysis, sorting is a very common task operate. The Pandas library is one of the powerful tools for data analysis in Python. It provides rich sorting functions that can sort data according to specific conditions. This article will introduce several commonly used sorting techniques and provide specific code examples.
1. Sort by a single column
First, let’s look at how to sort by a single column. The sort_values()
function in Pandas can sort DataFrame or Series objects. Below is an example data set, we will sort by the "score" column in descending order:
import pandas as pd data = {'name': ['Alice', 'Bob', 'Tom', 'Jerry'], 'score': [90, 80, 95, 85], 'age': [25, 30, 27, 23]} df = pd.DataFrame(data) df_sorted = df.sort_values(by='score', ascending=False) print(df_sorted)
Output results:
name score age 2 Tom 95 27 0 Alice 90 25 3 Jerry 85 23 1 Bob 80 30
In the above code, we use sort_values()
Function and set the parameter by
to the column name to be sorted. In addition, ascending=False
means descending sorting. If you want to sort in ascending order, set it to ascending=True
.
2. Sort by multiple columns
In addition to sorting by single column, we can also sort by multiple columns. When there are multiple sorting conditions, you can use the by
parameter of the sort_values()
function to pass in a list containing multiple column names. The following example will be sorted in descending order according to the "score" column. If the "score" columns are the same, then sorted in ascending order according to the "age" column:
import pandas as pd data = {'name': ['Alice', 'Bob', 'Tom', 'Jerry'], 'score': [90, 80, 95, 85], 'age': [25, 30, 27, 23]} df = pd.DataFrame(data) df_sorted = df.sort_values(by=['score', 'age'], ascending=[False, True]) print(df_sorted)
Output result:
name score age 2 Tom 95 27 0 Alice 90 25 3 Jerry 85 23 1 Bob 80 30
In the above code , we passed in a list containing two elements as the by
parameter, corresponding to the two sorting conditions. At the same time, we can set the sort order of each sorting condition by passing in a list of Boolean values.
3. Sort by index
In addition to sorting by columns, we can also sort by index. The sort_index()
function in Pandas can implement index sorting. Here is an example:
import pandas as pd data = {'name': ['Alice', 'Bob', 'Tom', 'Jerry'], 'score': [90, 80, 95, 85], 'age': [25, 30, 27, 23]} df = pd.DataFrame(data) df_sorted = df.sort_index(ascending=False) print(df_sorted)
Output result:
name score age 3 Jerry 85 23 2 Tom 95 27 1 Bob 80 30 0 Alice 90 25
In the above code, we sort the index by calling the sort_index()
function. The parameter ascending=False
indicates descending sorting. If you want to sort in ascending order, set it to ascending=True
.
4. Custom sorting function
Sometimes, we need to sort according to a custom function. The sort_values()
function in Pandas provides the parameter key
, which can be passed in a function for sorting. The following is an example:
import pandas as pd data = {'name': ['Alice', 'Bob', 'Tom', 'Jerry'], 'score': [90, 80, 95, 85], 'age': [25, 30, 27, 23]} df = pd.DataFrame(data) # 自定义排序函数,按照年龄和成绩之和进行排序 def custom_sort(row): return row['age'] + row['score'] df_sorted = df.sort_values(by='', key=custom_sort, ascending=False) print(df_sorted)
Output result:
name score age 2 Tom 95 27 3 Jerry 85 23 0 Alice 90 25 1 Bob 80 30
In the above code, we customized a sorting function custom_sort()
and passed it insort_values()
In the key
parameter of the function. This function compares sizes based on the sum of the "age" and "score" columns of the input rows.
Summary:
This article introduces several aspects of Pandas sorting techniques: sorting by single column, sorting by multiple columns, sorting by index, and custom sorting functions. The flexible use of these sorting functions makes it easy to sort data according to specific conditions. I hope the sample code in this article will be helpful to everyone in practice.
The above is the detailed content of Revealed: Detailed explanation of pandas techniques for sorting by specific conditions. For more information, please follow other related articles on the PHP Chinese website!
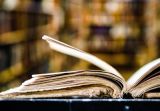
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
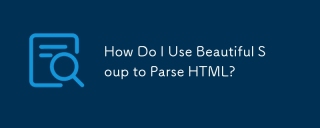
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
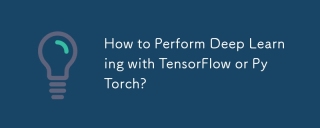
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
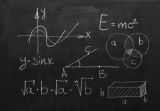
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
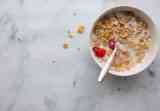
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
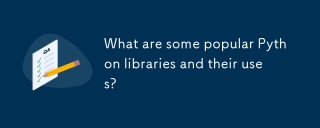
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
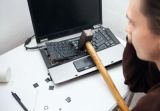
In this tutorial you'll learn how to handle error conditions in Python from a whole system point of view. Error handling is a critical aspect of design, and it crosses from the lowest levels (sometimes the hardware) all the way to the end users. If y
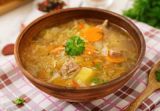
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
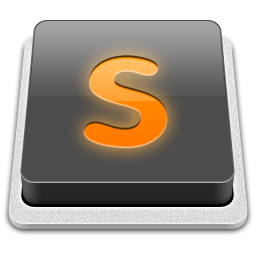
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
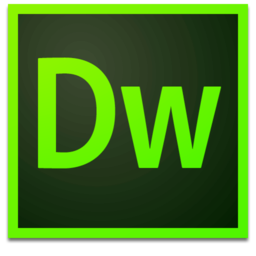
Dreamweaver Mac version
Visual web development tools
