Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request.
Serialization and deserialization are the most boring things in the world in a sense. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time.
This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format, or protocol you choose may determine how quickly the program runs, security, freedom of maintenance status, and the degree of interoperability with other systems.
There are so many options because different situations require different solutions. The "one-size-fits-all" approach doesn't work. In this two-part tutorial, I will:
- Overview of the advantages and disadvantages of the most successful serialization and deserialization schemes
- Show how to use them
- Provides guidelines for choosing between specific use cases
Running example
In the following section, we will serialize and deserialize the same Python object graph using different serializers. To avoid duplication, let's define these object graphs here.
Simple object diagram
A simple object graph is a dictionary containing a list of integers, strings, floating point numbers, boolean and datetime objects, as well as a user-defined class instance with dump, load, and dump() methods that can be serialized to an open file (file-like object).
-
The
load() method deserializes from an open file-like object.
-
TypeError: as follows: ``` Traceback (most recent call last):
File "serialize.py", line 49, in
print(json.dumps(complex)
File "/usr/lib/python3.8/json/init.py", line 231, in dumps
return _default_encoder.encode(obj)
File "/usr/lib/python3.8/json/encoder.py", line 199, in encode
chunks = self.iterencode(o, _one_shot=True)
File "/usr/lib/python3.8/json/encoder.py", line 257, in iterencode
return _iterencode(o, 0)
File "/usr/lib/python3.8/json/encoder.py", line 179, in default
raise TypeError(f'Object of type {o.class.name} '
TypeError: Object of type A is not JSON serializable<code> 哇!这看起来一点也不好。发生了什么?错误消息是 JSONEncoder 类使用的 default() 方法在 JSON 编码器遇到无法序列化的对象时调用的。 自定义编码器的任务是将其转换为 JSON 编码器能够编码的 Python 对象图。在本例中,我们有两个需要特殊编码的对象:A 类。以下编码器可以完成这项工作。每个特殊对象都转换为“\_\_A\_\_”和 pprint 函数的 load() 和 object_hook 参数,允许您提供自定义函数来将字典转换为对象。 </code>
def decode_object(o):
if 'A' in o:
a = A()
a.dict.update(o['A'])
return a
elif 'datetime' in o:
return datetime.strptime(o['datetime'], '%Y-%m-%dT%H:%M:%S')
return o<code> 让我们使用 object_hook 参数进行解码。 </code>
deserialized = json.loads(serialized, object_hook=decode_object)
print(deserialized)
# prints: {'a': main.a at="" object="">, 'when': datetime.datetime(2016, 3, 7, 0, 0)}
deserialized == complex
# evaluates to False
main.a><code> 结论 ---------- 在本教程的第一部分中,您学习了 Python 对象序列化和反序列化的通用概念,并探讨了使用 Pickle 和 JSON 序列化 Python 对象的来龙去脉。 在第二部分中,您将学习 YAML、性能和安全问题,以及对其他序列化方案的快速回顾。 *这篇文章已更新,并包含 Esther Vaati 的贡献。Esther 是 Envato Tuts+ 的软件开发人员和撰稿人。*</code>
The above is the detailed content of Serialization and Deserialization of Python Objects: Part 1. For more information, please follow other related articles on the PHP Chinese website!
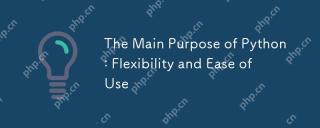
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
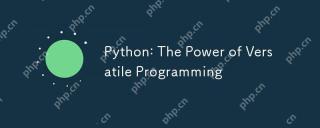
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
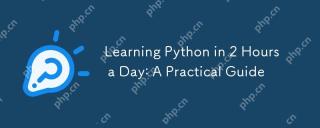
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.
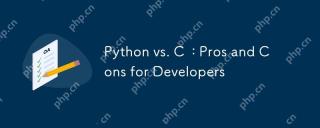
Python is suitable for rapid development and data processing, while C is suitable for high performance and underlying control. 1) Python is easy to use, with concise syntax, and is suitable for data science and web development. 2) C has high performance and accurate control, and is often used in gaming and system programming.
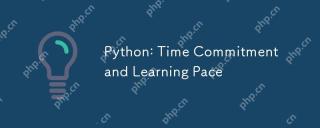
The time required to learn Python varies from person to person, mainly influenced by previous programming experience, learning motivation, learning resources and methods, and learning rhythm. Set realistic learning goals and learn best through practical projects.
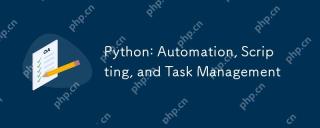
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
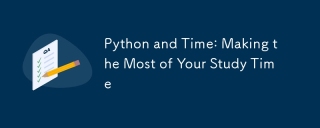
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
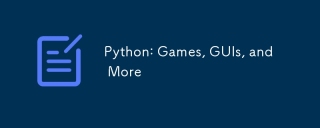
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
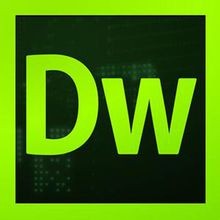
Dreamweaver CS6
Visual web development tools
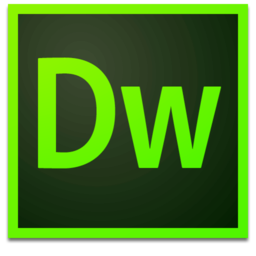
Dreamweaver Mac version
Visual web development tools