How to check the Django version and develop accordingly
Django is a popular Python web framework that provides rich functions and flexible development methods. As Django versions are constantly updated, it is important for developers to understand and use the appropriate version. This article will introduce how to check the Django version and develop accordingly, and provide relevant code examples.
The method to check the Django version is very simple, we can get it through the command line. At the command line, enter the following command to view the Django version:
python -m django --version
After running the command, the currently installed Django version number will be displayed. For example, the output result is 3.2.7
, indicating that the current Django version is 3.2.7.
For different Django versions, different development methods may be required. Below, we will take Django 3.2 as an example to introduce how to develop accordingly.
First, we need to install Django 3.2 version. At the command line, enter the following command to install:
pip install Django==3.2
After the installation is complete, we can create a new Django project. At the command line, enter the following command:
django-admin startproject myproject
This will create a new project named myproject
. Enter the project directory and we can start development.
Django 3.2 introduces many new features and improvements, the most important of which is new asynchronous view support. The new version also provides better type hints and optimized database connection pool management. Below we will demonstrate the use of these features through a specific code example.
Suppose we want to develop a simple blog application. First, we need to create a blog model and define the title, content, publication date, etc. of the blog. In the models.py
file in the project directory, add the following code:
from django.db import models class Blog(models.Model): title = models.CharField(max_length=200) content = models.TextField() publish_date = models.DateTimeField(auto_now_add=True)
Next, we need to create a blog view to display the blog list and detailed content. In the views.py
file in the project directory, add the following code:
from django.shortcuts import render from .models import Blog def blog_list(request): blogs = Blog.objects.all() return render(request, 'blog_list.html', {'blogs': blogs}) def blog_detail(request, blog_id): blog = Blog.objects.get(id=blog_id) return render(request, 'blog_detail.html', {'blog': blog})
Then, we create a blog list template blog_list.html
for displaying blogs list. In the templates
folder under the project directory, create the blog_list.html
file and add the following code:
{% for blog in blogs %} <h2 id="blog-title">{{ blog.title }}</h2> <p>{{ blog.publish_date }}</p> {% endfor %}
At the same time, we create a blog details templateblog_detail.html
, used to display the detailed content of the blog. In the templates
folder, create the blog_detail.html
file and add the following code:
<h2 id="blog-title">{{ blog.title }}</h2> <p>{{ blog.content }}</p>
Finally, we need to configure URL routing to combine the blog list and details view associated with the corresponding URL path. In the urls.py
file in the project directory, add the following code:
from django.urls import path from .views import blog_list, blog_detail urlpatterns = [ path('blogs/', blog_list, name='blog_list'), path('blogs/<int:blog_id>/', blog_detail, name='blog_detail'), ]
Now, we can run the development server and view the results. At the command line, enter the following command:
python manage.py runserver
Then, visit http://localhost:8000/blogs/
in the browser, the blog list page will be displayed.
The above is a simple example of development using Django 3.2. Of course, depending on specific needs, we can also use other functions and features provided by Django, such as user authentication, form processing, RESTful API, etc.
Through the method introduced in this article, we can easily check the Django version and develop accordingly. In actual projects, choose the appropriate Django version according to your own needs, and make good use of the functions it provides to improve development efficiency.
The above is the detailed content of Django version query and development guide. For more information, please follow other related articles on the PHP Chinese website!
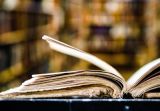
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
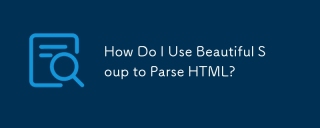
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
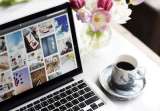
Dealing with noisy images is a common problem, especially with mobile phone or low-resolution camera photos. This tutorial explores image filtering techniques in Python using OpenCV to tackle this issue. Image Filtering: A Powerful Tool Image filter
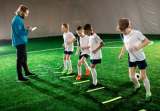
Python, a favorite for data science and processing, offers a rich ecosystem for high-performance computing. However, parallel programming in Python presents unique challenges. This tutorial explores these challenges, focusing on the Global Interprete
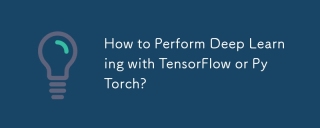
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
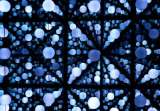
This tutorial demonstrates creating a custom pipeline data structure in Python 3, leveraging classes and operator overloading for enhanced functionality. The pipeline's flexibility lies in its ability to apply a series of functions to a data set, ge
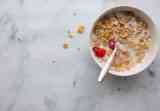
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
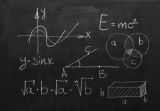
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
